集成开发环境:IntelliJ IDEA;
使用插件:Scene Builder;
数据库:MySQL 8.0.23;
技术栈:JavaFx、SpringBoot,mybatisPlus
适用于课程设计,大作业,项目练习,学习演示等
简介
该系统角色采用了管理员和操作员模块,其中管理员模块对贫困种类和身份以及操作员进行管理(增删改查),操作员模块对户主及其户主下的家庭成员进行了管理!
界面展示
操作员涉及页面
登录页面
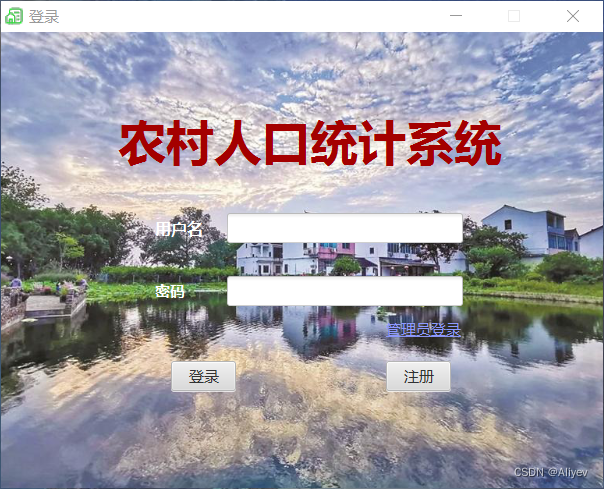
注册页面
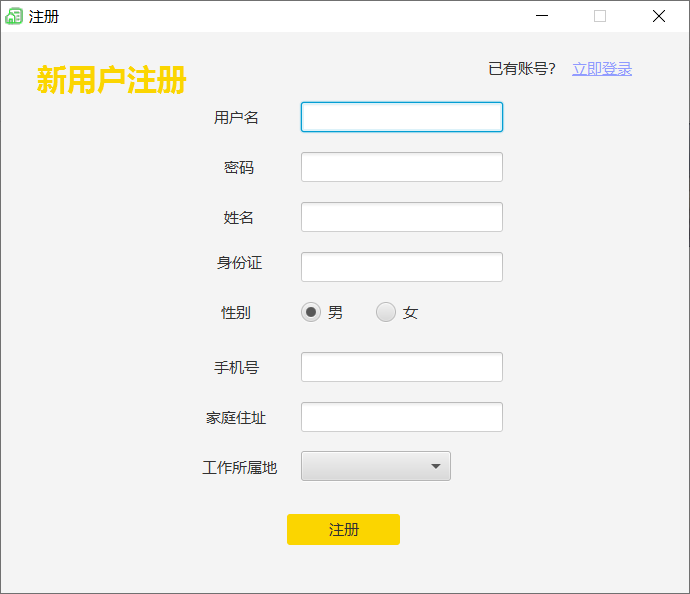
户主管理页面
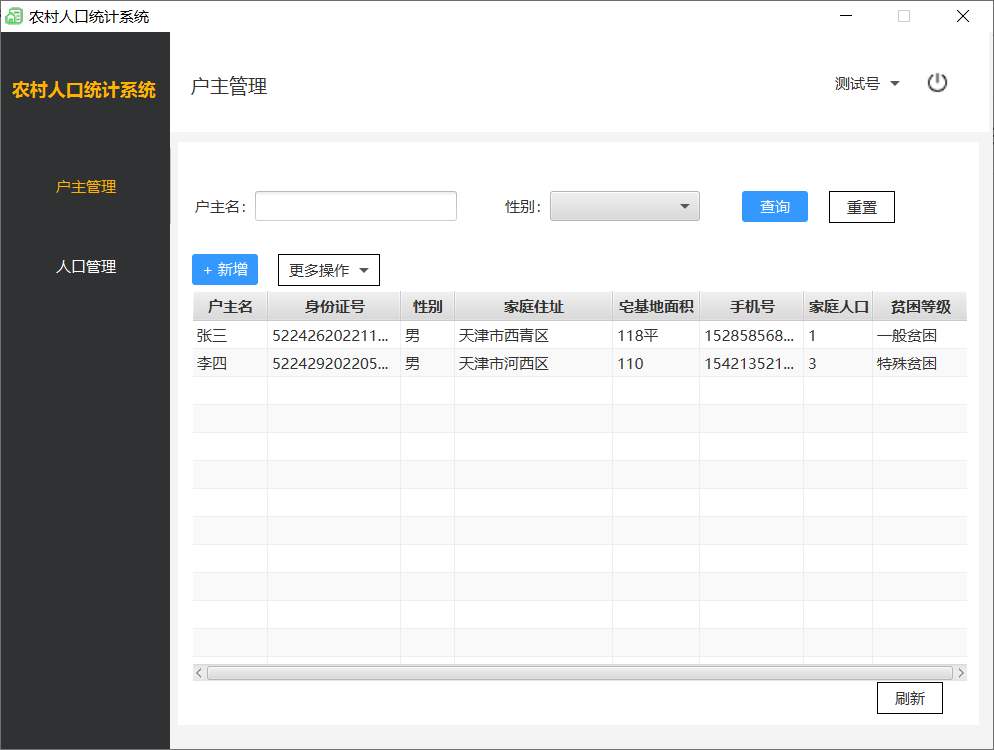
人口管理页面
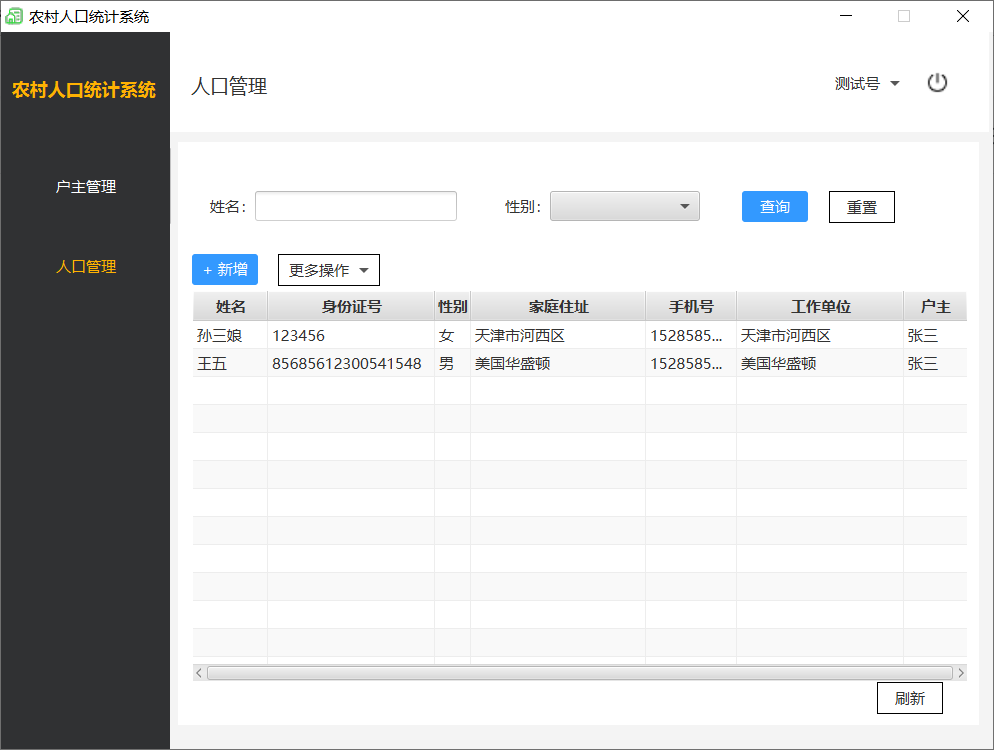
管理员涉及页面
登录页面
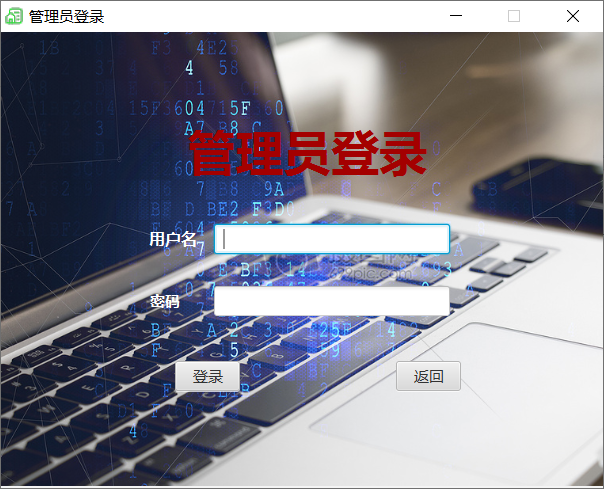
员工(操作员)管理页面
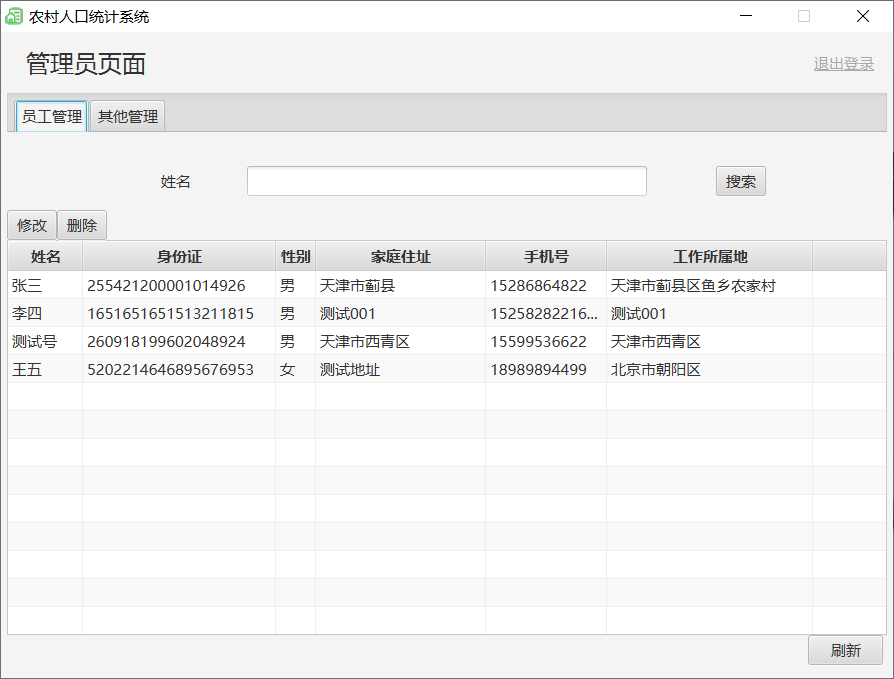
贫困等级及工作地管理页面
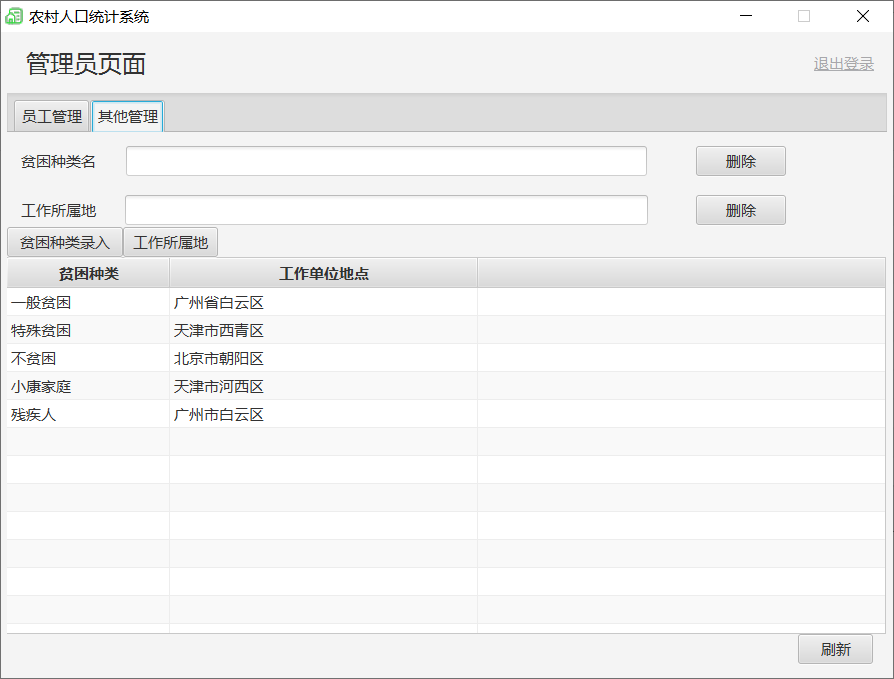
代码(部分)
启动类
package com.aliyev;
import com.aliyev.util.PageName;
import com.aliyev.view.AdminIndexView;
import com.aliyev.view.AdminLoginView;
import com.aliyev.view.LoginView;
import de.felixroske.jfxsupport.AbstractFxmlView;
import de.felixroske.jfxsupport.AbstractJavaFxApplicationSupport;
import javafx.scene.image.Image;
import javafx.stage.Stage;
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ConfigurableApplicationContext;
import org.springframework.stereotype.Service;
import java.util.Arrays;
import java.util.Collection;
@SpringBootApplication
@Slf4j
public class RpstApplication extends AbstractJavaFxApplicationSupport {
public static void main(String[] args) {
launch(RpstApplication.class, LoginView.class,args);
log.info("项目启动~~~");
}
@Override
public void beforeInitialView(Stage stage, ConfigurableApplicationContext ctx) {
stage.setTitle(PageName.LOGIN);
stage.setResizable(false);
}
/**
* 修改默认图标
* @return
*/
@Override
public Collection<Image> loadDefaultIcons() {
return Arrays.asList(new Image("images/icon.png"));
}
/**
* 设置窗口标题
* @param title
*/
public static void setTitle(String title){
RpstApplication.getStage().setTitle(title);
}
public static void showNewView(Class<? extends AbstractFxmlView> newView,String title){
RpstApplication.showView(newView);
setTitle(title);
}
}
管理员登录
package com.aliyev.controller.login;
/**
* Sample Skeleton for 'adminLogin.fxml' Controller Class
*/
import com.aliyev.RpstApplication;
import com.aliyev.domain.Employee;
import com.aliyev.service.EmployeeService;
import com.aliyev.util.Rpstutils;
import com.aliyev.util.PageName;
import com.aliyev.util.SpringContextUtil;
import com.aliyev.view.AdminIndexView;
import com.aliyev.view.IndexView;
import com.aliyev.view.LoginView;
import de.felixroske.jfxsupport.FXMLController;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.*;
import javafx.scene.input.MouseEvent;
import javafx.stage.Stage;
import lombok.extern.slf4j.Slf4j;
import java.net.URL;
import java.util.List;
import java.util.Optional;
import java.util.ResourceBundle;
@FXMLController
@Slf4j
public class AdminLoginController implements Initializable {
@FXML // fx:id="login"
private Button login; // Value injected by FXMLLoader
@FXML // fx:id="button_href"
private Button button_href; // Value injected by FXMLLoader
@FXML // fx:id="username"
private TextField username; // Value injected by FXMLLoader
@FXML // fx:id="password"
private PasswordField password; // Value injected by FXMLLoader
public EmployeeService employeeService;
@FXML
void href(ActionEvent event) {
RpstApplication.showView(LoginView.class);
Stage stage = RpstApplication.getStage();
stage.setTitle(PageName.LOGIN);
}
/**
* 管理员登录
* @param event
*/
@FXML
void login(ActionEvent event) {
String username = this.username.getText().trim();
String password = this.password.getText().trim();
if (username.equals("")||password.equals("")){
Rpstutils.getAlter(Alert.AlertType.WARNING,"请输入账号和密码后再登录!!!");
return;
}
List<Employee> user = employeeService.getOneEmployee(username,0);
if(user.size()!=0){
for (Employee employee : user) {
if(password.equals(employee.getPassword())){
Stage window = (Stage) this.username.getScene().getWindow();
window.close();
RpstApplication.showView(AdminIndexView.class);
Stage stage = RpstApplication.getStage();
stage.setTitle(PageName.INDEX);
}else{
Rpstutils.getAlter(Alert.AlertType.WARNING,"用户名或密码错误!!!");
}
}
}else {
Rpstutils.getAlter(Alert.AlertType.ERROR,"该账号不存在!!1");
}
}
@Override
public void initialize(URL location, ResourceBundle resources) {
employeeService = SpringContextUtil.getBean(EmployeeService.class);
}
}
操作员登录
package com.aliyev.controller.login;
import com.aliyev.RpstApplication;
import com.aliyev.domain.Employee;
import com.aliyev.service.EmployeeService;
import com.aliyev.util.PageName;
import com.aliyev.util.Rpstutils;
import com.aliyev.util.SpringContextUtil;
import com.aliyev.view.AdminIndexView;
import com.aliyev.view.AdminLoginView;
import com.aliyev.view.IndexView;
import com.aliyev.view.RegisterView;
import de.felixroske.jfxsupport.FXMLController;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.*;
import javafx.scene.input.MouseEvent;
import javafx.stage.Stage;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import java.net.URL;
import java.util.List;
import java.util.ResourceBundle;
@FXMLController
@Slf4j
public class LoginController implements Initializable {
@FXML
private Button login;
@FXML
private Button redister;
@FXML
private Label admin;
@FXML
private TextField username;
@FXML
private PasswordField password;
private EmployeeService employeeService;
public static Employee LoginUser;
/**
* 管理员登录
*
* @param event
*/
@FXML
void adminLogin(MouseEvent event) {
RpstApplication.showView(AdminLoginView.class);
RpstApplication.getStage().setTitle(PageName.ADMINLOGIN);
}
/**
* 普通用户登录
*
* @param event
*/
@FXML
void login(ActionEvent event) {
String username = this.username.getText().trim();
String password = this.password.getText().trim();
if (username.equals("") || password.equals("")) {
Rpstutils.getAlter(Alert.AlertType.WARNING, "请输入账号和密码后再登录!!!");
return;
}
List<Employee> user = employeeService.getOneEmployee(username, 1);
if (user.size() != 0) {
for (Employee employee : user) {
if (password.equals(employee.getPassword())) {
LoginUser=employee;
Stage window = (Stage) this.username.getScene().getWindow();
window.close();
RpstApplication.showView(IndexView.class);
Stage stage = RpstApplication.getStage();
stage.setTitle(PageName.INDEX);
return;
} else {
Rpstutils.getAlter(Alert.AlertType.WARNING, "用户名或密码错误!!!");
}
}
} else {
Rpstutils.getAlter(Alert.AlertType.ERROR, "该账号不存在!!1");
}
}
/**
* 注册
*
* @param event
*/
@FXML
void register(ActionEvent event) {
Stage stage = (Stage) password.getScene().getWindow();
stage.close();
RpstApplication.showView(RegisterView.class);
RpstApplication.setTitle(PageName.REGISTER);
}
@Override
public void initialize(URL location, ResourceBundle resources) {
log.info("登录页面启动~");
employeeService = SpringContextUtil.getBean(EmployeeService.class);
}
}
由于所涉及代码过多,需要者留下邮箱或者加博主qq2690176953~~
创作不易,点点小赞哦^_^·····