abstract是抽象修饰符,用来修饰类和方法。
用abstract修饰的类都是抽象类。用abstract修饰的方法是抽象方法。
抽象方法只有方法体,并无具体的方法体和方法实现。
有抽象方法的类必须定义成抽象类(当然还有可能是接口),抽象类可以有抽象方法,也可以没有。
一般来说,抽象类必须有子类来继承,否则就失去了存在的意义。
abstract class Shape
{
public static final double PI = 3.14;
protected double length;
protected double width;
public Shape(double len, double wid)
{
this.length = len;
this.width = wid;
}
public Shape(double len)
{
this.length = len;
}
public abstract double area();
}
class Rect extends Shape
{
public Rect(double len, double wid) {
super(len, wid);
}
@Override
public double area()
{
return length * width;
}
}
class Circle extends Shape
{
public Circle(double len)
{
super(len);
}
//下面重写area()方法,重写时候不可降低访问权限
@Override
public double area()
{
return PI * length * length;
}
}
public class TestAbstract {
public static void main(String args[])
{
Rect a = new Rect(2.5, 1.5);
System.out.println(a.area());
Circle b = new Circle(2.5);
System.out.println(b.area());
}
}
运行结果:
3.75
19.625
接口中的方法都是抽象方法,接口需要类来实现。实现接口的类必须定义接口中所有的方法,否则这个类就必须定义成抽象类。(因为 有抽象方法的类必须定义成抽象类)。 Java中没有多继承,即一个类不能继承自多个类。但接口解决了多继承的问题。一个类可以实现多个接口
扫描二维码关注公众号,回复:
15108635 查看本文章
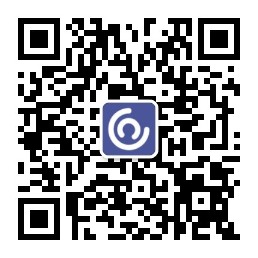
public class TestInterface
{
public static void main(String[] args)
{
Circle2 a = new Circle2(2);
System.out.println("a: " + a.area() + " " + a.cir());
Rect2 b = new Rect2(3, 2);
System.out.println("b: " + b.area() + " " + b.cir());
}
}
interface ShapeArea
{
final double pi = 3.14;
double area();
}
interface ShapeCir
{
double cir();
}
class Circle2 implements ShapeArea, ShapeCir //叫Circle2是因为同一个包中,已经定义了Circle类,叫Circle会出现重名报错
{
double redius;
Circle2(double red)
{
this.redius = red;
}
@Override
public double cir() {
return 2 * pi * redius;
}
@Override
public double area() {
return pi * redius * redius;
}
}
class Rect2 implements ShapeArea, ShapeCir
{
double length, width;
Rect2(double len, double wid)
{
this.length = len;
this.width = wid;
}
@Override
public double cir() {
return 2 * (length + width);
}
@Override
public double area() {
return length * width;
}
}
运行结果:
a: 12.56 12.56
b: 6.0 10.0