看到新奇又实用的第三方库,当然要分享出来咯~
Plottable
Plottable是一个Python库,用于在matplotlib基础上绘制精美表格。例如下图所示表格。
代码如下:
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from matplotlib.colors import LinearSegmentedColormap
from plottable import ColumnDefinition, Table
from plottable.formatters import decimal_to_percent
from plottable.plots import bar, percentile_bars, percentile_stars, progress_donut
cmap = LinearSegmentedColormap.from_list(
name="bugw", colors=["#ffffff", "#f2fbd2", "#c9ecb4", "#93d3ab", "#35b0ab"], N=256
)
fig, ax = plt.subplots(figsize=(6, 10))
d = pd.DataFrame(np.random.random((10, 4)), columns=["A", "B", "C", "D"]).round(2)
tab = Table(
d,
cell_kw={
"linewidth": 0,
"edgecolor": "k",
},
textprops={
"ha": "center"},
column_definitions=[
ColumnDefinition("index", textprops={
"ha": "left"}),
ColumnDefinition("A", plot_fn=percentile_bars, plot_kw={
"is_pct": True}),
ColumnDefinition(
"B", width=1.5, plot_fn=percentile_stars, plot_kw={
"is_pct": True}
),
ColumnDefinition(
"C",
plot_fn=progress_donut,
plot_kw={
"is_pct": True,
"formatter": "{:.0%}"
},
),
ColumnDefinition(
"D",
width=1.25,
plot_fn=bar,
plot_kw={
"cmap": cmap,
"plot_bg_bar": True,
"annotate": True,
"height": 0.5,
"lw": 0.5,
"formatter": decimal_to_percent,
},
),
],
)
plt.show()
Moviepy
MoviePy是一个用于视频编辑的Python模块,你可以用它实现一些基本的操作(如视频截取、拼接、添加字幕等)亦或自定义的高级特效等。
笔者这里clips_array函数为例,实现将多个视频同时显示在同一个画面上。代码如下:
from moviepy import editor
# margin: 设置外边距
video_clip = editor.VideoFileClip(r"demo.mp4").margin(10)
video_clip1 = video_clip.subclip(10, 20)
# editor.vfx.mirror_x: x轴镜像
video_clip2 = video_clip1.fx(editor.vfx.mirror_x)
# editor.vfx.mirror_y: y轴镜像
video_clip3 = video_clip1.fx(editor.vfx.mirror_y)
# resize: 等比缩放
video_clip4 = video_clip1.resize(0.8)
# 列表里面有两个列表,所以会将屏幕上下等分
# 上半部分显示video_clip1, video_clip2,下半部分显示video_clip3, video_clip4
clip = editor.clips_array([[video_clip1, video_clip2], [video_clip3, video_clip4]])
clip.ipython_display(width=600)
Plotly Express
Plotly Express是一个非常强大的Python开源数据可视化框架,它通过构建基于HTML的交互式图表来显示信息,可生成各种精美动画图。笔者在这里以下图所示动画图为例说明。
代码如下:
import plotly.express as px
df = px.data.gapminder()
fig = px.scatter(df, x="gdpPercap", y="lifeExp", animation_frame="year", animation_group="country",
size="pop", color="continent", hover_name="country",
log_x=True, size_max=55, range_x=[100,100000], range_y=[25,90])
fig.show()
You-Get
You-Get是一个非常优秀的网站视频下载工具。使用You-Get可以很轻松的下载到网络上的视频、图片及音乐。
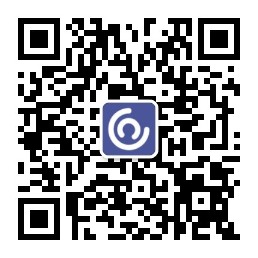
给网址就下视频啊! 太好用了,有木有?
Autopep8
Autopep8是一个将Python代码自动排版为PEP8风格的工具。它使用 pycodestyle程序来确定代码的哪些部分需要排版,可以修复大部分pycodestyle报告的排版问题。
例如下面一段代码。
- 排版前:
import math, sys;
def example1():
####This is a long comment. This should be wrapped to fit within 72 characters.
some_tuple=( 1,2, 3,'a' );
some_variable={
'long':'Long code lines should be wrapped within 79 characters.',
'other':[math.pi, 100,200,300,9876543210,'This is a long string that goes on'],
'more':{
'inner':'This whole logical line should be wrapped.',some_tuple:[1,
20,300,40000,500000000,60000000000000000]}}
return (some_tuple, some_variable)
def example2(): return {
'has_key() is deprecated':True}.has_key({
'f':2}.has_key(''));
class Example3( object ):
def __init__ ( self, bar ):
#Comments should have a space after the hash.
if bar : bar+=1; bar=bar* bar ; return bar
else:
some_string = """
Indentation in multiline strings should not be touched.
Only actual code should be reindented.
"""
return (sys.path, some_string)
- 排版后:
import math
import sys
def example1():
# This is a long comment. This should be wrapped to fit within 72
# characters.
some_tuple = (1, 2, 3, 'a')
some_variable = {
'long': 'Long code lines should be wrapped within 79 characters.',
'other': [
math.pi,
100,
200,
300,
9876543210,
'This is a long string that goes on'],
'more': {
'inner': 'This whole logical line should be wrapped.',
some_tuple: [
1,
20,
300,
40000,
500000000,
60000000000000000]}}
return (some_tuple, some_variable)
def example2(): return ('' in {
'f': 2}) in {
'has_key() is deprecated': True}
class Example3(object):
def __init__(self, bar):
# Comments should have a space after the hash.
if bar:
bar += 1
bar = bar * bar
return bar
else:
some_string = """
Indentation in multiline strings should not be touched.
Only actual code should be reindented.
"""
return (sys.path, some_string)
TextBlob
TextBlob是一个用Python编写的开源的文本处理库。它可以用来执行很多自然语言处理的任务,比如,词性标注、名词性成分提取、情感分析、文本翻译、拼接检查等等。笔者在这里以拼接检查为例说明,感兴趣的小伙伴可以去官方文档阅读TextBlob的所有特性。
from textblob import TextBlob
b = TextBlob("I havv goood speling!")
print(b.correct()) #I have good spelling!
输出结果为:“I have good spelling!” 可以看到,句子中的单词被更正了。
Pygame
Pygame是一组用来开发游戏软件的Python程序模块,基于SDL库的基础上实现。你可以利用Pygame实现各种功能丰富的游戏和多媒体程序,同时,他也是一个高移植性模块,可以支持多个操作系统。
下面我们使用pygame来制作一个小型的音乐播放器。
from pygame import mixer
import pygame
import sys
pygame.display.set_mode([300, 300])
music = "my_dream.mp3"
mixer.init()
mixer.music.load(music)
mixer.music.play()
# 点击×可以关闭界面的代码
while 1:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
运行上面的代码,电脑就会播放音乐。
pyqrcode
pyqrcode是用来生成二维码的第三方模块,可以在控制台输出二维码,也可以将二维码保存为图片,不过依赖pypng包。
以「百度一下」生成一个二维码为例,代码如下。
import logging
import os
import pyqrcode
logging.basicConfig(level = logging.DEBUG, format='%(levelname)s - %(message)s')
logger = logging.getLogger(__name__)
# 生成二维码
qr = pyqrcode.create("http://www.baidu.com")
if not os.path.exists('qrcode'):
os.mkdir('qrcode')
# 生成二维码图片
qr.png(os.path.join('qrcode', 'qrcode.png'), scale=8)
# 在终端打印
print(qr.terminal(quiet_zone=1))