目录
参考
小玩意 - 一篇带你玩转 SpringBoot 钉钉机器人
官方教程-自定义机器人接入
一、场景介绍
企业内部有较多系统支撑着公司的核心业务流程,譬如CRM系统、交易系统、监控报警系统等等。通过钉钉的自定义机器人,可以将这些系统事件同步到钉钉的聊天群。
说明
当前机器人尚不支持应答机制,该机制指的是群里成员在聊天@机器人的时候,钉钉回调指定的服务地址,即Outgoing机器人。
调用频率限制
由于消息发送太频繁会严重影响群成员的使用体验,因此钉钉开放平台对自定义机器人发送消息的频率作出以下限制:
每个机器人每分钟最多发送20条消息到群里,如果超过20条,会限流10分钟。
注意 如果你有大量发消息的场景(譬如系统监控报警)可以将这些信息进行整合,通过markdown消息以摘要的形式发送到群里。
二、创建 Ding 群
首先在钉钉软件里创建一个群聊,这个比较简单就不多说啥了,主要注意的地方是一个群聊至少三个人,但是如何做到 1 个人创建一个群呢?也很简单,只要一开始拉 2 个人进来,然后创建成功后把他们踢出去就行,这样就实现 1 个人在一个群里,就可以和机器人愉快地玩耍啦~
三、添加自定义机器人
添加机器人
添加-选择加签,保存这个secret
保存这个webhook地址
四、编码
1、引入包
<dependency>
<groupId>com.aliyun</groupId>
<artifactId>alibaba-dingtalk-service-sdk</artifactId>
<version>2.0.0</version>
</dependency>
2、封装公共方法
private static final String SECRET = "SEC2c885aef51xxxxxx4703fc94de28c6b";
private static final String URL = "https://oapi.dingtalk.com/robot/send?access_token=0e82c5326dd33b4xxxxx026ed53eb47b47";
/**
* 组装签名url
* @return url
*/
public static String getURL()throws NoSuchAlgorithmException, UnsupportedEncodingException, InvalidKeyException {
Long timestamp = System.currentTimeMillis();
String stringToSign = timestamp + "\n" + SECRET;
Mac mac = Mac.getInstance("HmacSHA256");
mac.init(new SecretKeySpec(SECRET.getBytes(StandardCharsets.UTF_8), "HmacSHA256"));
byte[] signData = mac.doFinal(stringToSign.getBytes(StandardCharsets.UTF_8));
String sign = URLEncoder.encode(new String(Base64.encodeBase64(signData)), "UTF-8");
String signResult = "×tamp=" + timestamp + "&sign=" + sign;
// 得到拼接后的 URL
return URL + signResult;
}
/**
* 获取客户端
* @return
*/
public static DingTalkClient getClient()throws NoSuchAlgorithmException, UnsupportedEncodingException, InvalidKeyException{
return new DefaultDingTalkClient(getURL());
}
3、发送文本消息
如何设置at.setIsAtAll(true);则消息会@所有人
public static void sendText(String content) throws ApiException, NoSuchAlgorithmException, UnsupportedEncodingException, InvalidKeyException {
DingTalkClient client = getClient();
OapiRobotSendRequest request = new OapiRobotSendRequest();
request.setMsgtype("text");
OapiRobotSendRequest.Text text = new OapiRobotSendRequest.Text();
text.setContent(content);
request.setText(text);
OapiRobotSendRequest.At at = new OapiRobotSendRequest.At();
// isAtAll类型如果不为Boolean,请升级至最新SDK
at.setIsAtAll(false);
at.setAtMobiles(Arrays.asList("1392xxxxx","155xxxx"));
request.setAt(at);
OapiRobotSendResponse response = client.execute(request);
log.info("success:{}, code:{}, errorCode:{}, errorMsg:{}",response.isSuccess(),response.getCode(),response.getErrcode(),response.getErrmsg());
}
4、发送Link消息
如何设置at.setIsAtAll(true);则消息会@所有人
@SneakyThrows
public static void sendLink(){
DingTalkClient client = getClient();
OapiRobotSendRequest request = new OapiRobotSendRequest();
request.setMsgtype("link");
OapiRobotSendRequest.Link link = new OapiRobotSendRequest.Link();
link.setMessageUrl("https://www.dingtalk.com/");
link.setPicUrl("https://gw.alicdn.com/tfs/TB1ut3xxbsrBKNjSZFpXXcXhFXa-846-786.png");
link.setTitle("时代的火车向前开");
link.setText("这个即将发布的新版本,创始人xx称它为红树林。而在此之前,每当面临重大升级,产品经理们都会取一个应景的代号,这一次,为什么是红树林");
request.setLink(link);
OapiRobotSendResponse response = client.execute(request);
}
5、发送MarkDown消息
@SneakyThrows
public static void sendMarkDown(){
DingTalkClient client = getClient();
OapiRobotSendRequest request = new OapiRobotSendRequest();
OapiRobotSendRequest.Markdown markdown = new OapiRobotSendRequest.Markdown();
request.setMsgtype("markdown");
markdown.setTitle("杭州天气");
markdown.setText("#### 杭州天气 \n" +
"> 9度,西北风1级,空气良89,相对温度73%\n\n" +
"> 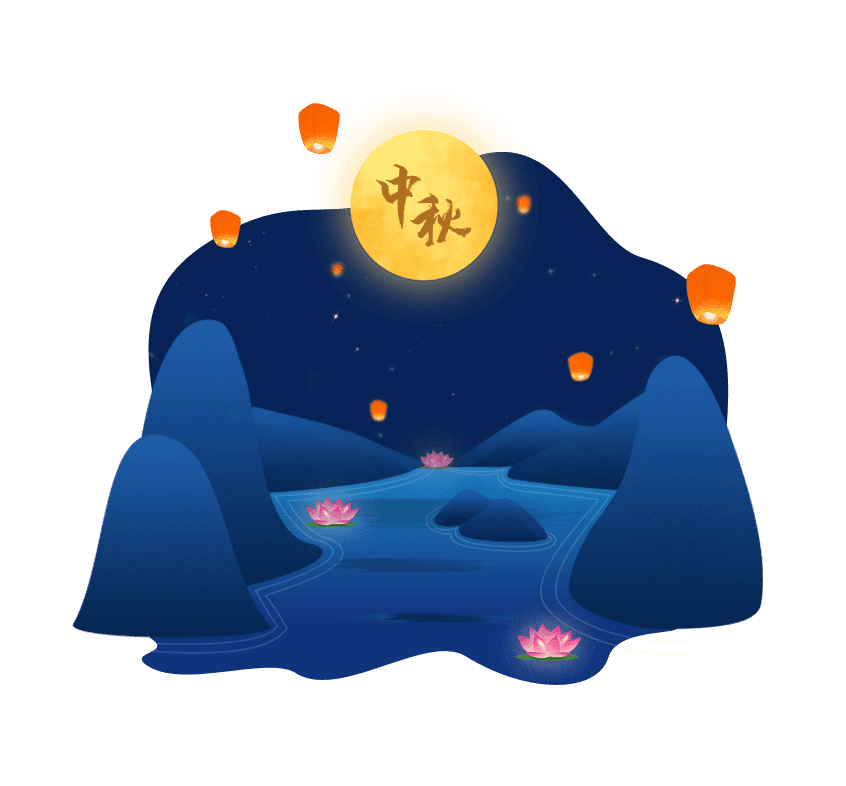\n" +
"> ###### 10点20分发布 [天气](http://www.thinkpage.cn/) \n");
request.setMarkdown(markdown);
OapiRobotSendRequest.At at = new OapiRobotSendRequest.At();
at.setAtMobiles(Arrays.asList("1392xxxxx","155xxxx"));
request.setAt(at);
OapiRobotSendResponse response = client.execute(request);
System.out.printf("success:%s, code:%s, errorCode:%s, errorMsg:%s%n",response.isSuccess(),response.getCode(),response.getErrcode(),response.getErrmsg());
}
6、发送ActionCard消息
@SneakyThrows
public static void sendActionCard(){
DingTalkClient client = getClient();
OapiRobotSendRequest request = new OapiRobotSendRequest();
OapiRobotSendRequest.Actioncard card = new OapiRobotSendRequest.Actioncard();
request.setMsgtype("actionCard");
card.setTitle("乔布斯 20 年前想打造一间苹果咖啡厅,而它正是 Apple Store 的前身");
card.setText("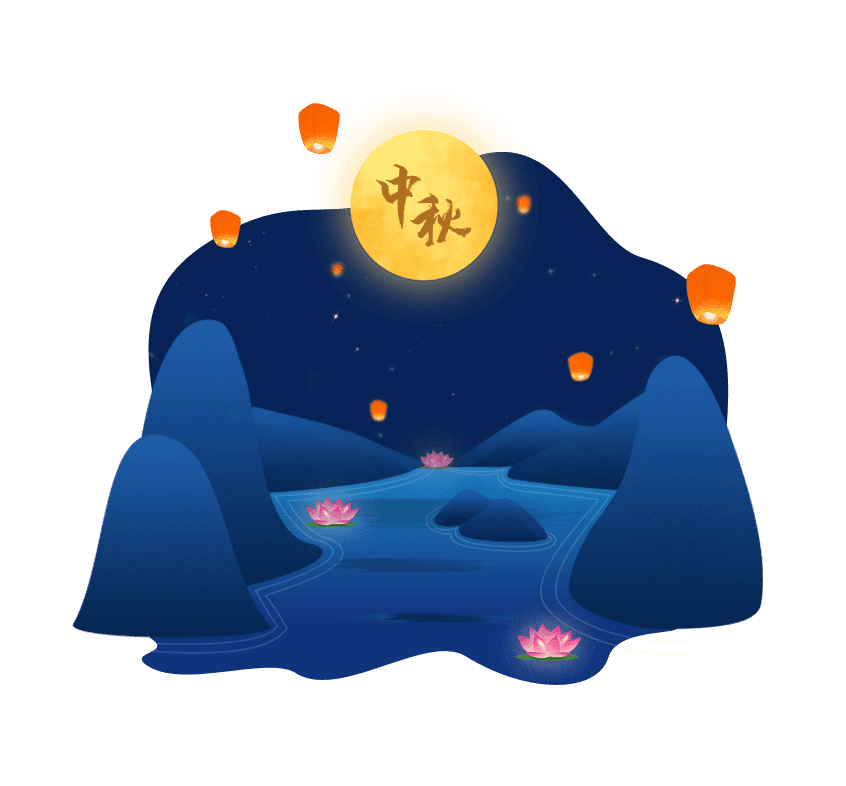 \n" +
" ### 乔布斯 20 年前想打造的苹果咖啡厅 \n" +
" Apple Store 的设计正从原来满满的科技感走向生活化,而其生活化的走向其实可以追溯到 20 年前苹果一个建立咖啡馆的计");
// 0:按钮竖直排列, 1:按钮横向排列
card.setBtnOrientation("0");
card.setSingleTitle("阅读全文");
card.setSingleURL("https://www.dingtalk.com/");
request.setActionCard(card);
OapiRobotSendRequest.At at = new OapiRobotSendRequest.At();
at.setAtMobiles(Arrays.asList("1392xxxxx","155xxxx"));
request.setAt(at);
OapiRobotSendResponse response = client.execute(request);
}
7、发送FeedCard消息
@SneakyThrows
@SneakyThrows
public static void sendFreeCard(){
DingTalkClient client = getClient();
OapiRobotSendRequest request = new OapiRobotSendRequest();
OapiRobotSendRequest.Feedcard card = new OapiRobotSendRequest.Feedcard();
request.setMsgtype("feedCard");
List<OapiRobotSendRequest.Links> data = new ArrayList<>();
OapiRobotSendRequest.Links links1 = new OapiRobotSendRequest.Links();
OapiRobotSendRequest.Links links2 = new OapiRobotSendRequest.Links();
OapiRobotSendRequest.Link link1 = new OapiRobotSendRequest.Link();
link1.setText("标题1");
link1.setTitle("时代的火车向前开1");
link1.setPicUrl("https://img.alicdn.com/tfs/TB1NwmBEL9TBuNjy1zbXXXpepXa-2400-1218.png");
link1.setMessageUrl("https://www.dingtalk.com/");
links1.setTitle("时代的火车向前开1");
links1.setPicURL("https://img.alicdn.com/tfs/TB1NwmBEL9TBuNjy1zbXXXpepXa-2400-1218.png");
links1.setMessageURL("https://www.dingtalk.com/");
data.add(links1);
links2.setTitle("时代的火车向前开2");
links2.setPicURL("https://img.alicdn.com/tfs/TB1NwmBEL9TBuNjy1zbXXXpepXa-2400-1218.png");
links2.setMessageURL("https://www.dingtalk.com/");
data.add(links2);
card.setLinks(data);
request.setFeedCard(card);
OapiRobotSendRequest.At at = new OapiRobotSendRequest.At();
at.setAtMobiles(Arrays.asList("1392xxxxx","155xxxx"));
request.setAt(at);
OapiRobotSendResponse response = client.execute(request);
}
8、发送交互消息
参考官网文档:ActionCard