加载BMP
1.使用SDL_init初始化SDL库
2.使用SDL_CreateWindow创建一个窗口
3.使用SDL_GetWindowSurface获取创建窗口的surface
4.使用SDL_LoadBMP加载一张BMP图片
5.使用SDL_BlitSurface将加载的bmp surface拷贝到窗口的surface
6.使用SDL_UpdateWindowSurface更新到窗口
7.使用SDL_FreeSurface释放申请的空间
8.使用SDL_DestroyWindow销毁窗口
9.使用SDL_Quit释放SDL库
以下时完整代码
#include <iostream>
#include <sdl.h>
const int SCREEN_WIDTH = 640;
const int SCREEN_HEIGHT = 480;
SDL_Window* gWindow = nullptr;
SDL_Surface* gScreenSurface = nullptr;
SDL_Surface* gHelloWorld = nullptr;
bool init()
{
bool success = true;
if (SDL_Init(SDL_INIT_VIDEO) < 0)
{
printf("SDL could not initialize!SDL Error:%s\n", SDL_GetError());
success = false;
}
else
{
//create window
gWindow = SDL_CreateWindow("SDL tutorial", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, SCREEN_WIDTH, SCREEN_HEIGHT, SDL_WINDOW_SHOWN);
if (gWindow == nullptr)
{
printf("window could not created!SDL_Error:%s\n", SDL_GetError());
success = false;
}
else
{
//get window surface
gScreenSurface = SDL_GetWindowSurface(gWindow);
}
}
return success;
}
bool loadMedia()
{
//loading success flag
bool success = true;
//load splash image
gHelloWorld = SDL_LoadBMP("../BMP2.bmp");
if (gWindow == nullptr)
{
printf("unable to load image!SDL_ERROR%s\n",SDL_GetError());
success = false;
}
return success;
}
void close()
{
SDL_FreeSurface(gHelloWorld);
gHelloWorld = NULL;
SDL_DestroyWindow(gWindow);
gWindow = NULL;
SDL_Quit();
}
int main(int argc, char* args[])
{
if (!init())
{
printf("failed to initialize!\n");
}
else
{
//load media
if (!loadMedia())
{
printf("failed to load media!\n");
}
else
{
SDL_BlitSurface(gHelloWorld, nullptr, gScreenSurface, nullptr);
//update the surface
SDL_UpdateWindowSurface(gWindow);
SDL_Event e;
bool quit = false;
while (quit == false)
{
while (SDL_PollEvent(&e))
{
if (e.type == SDL_QUIT)
quit = true;
}
}
}
}
close();
return 0;
}
扫描二维码关注公众号,回复:
14893259 查看本文章
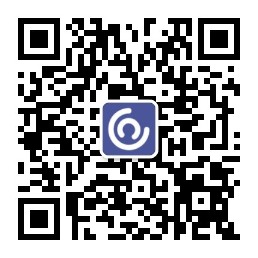
加载PNG
由于默认的SDL库只能加载BMP格式的图片,如果需要加载png等其他格式的图片,需要下载一个扩展库SDL_image
这里我们下载SDL2_image-devel-2.6.3-VC.zipSDL_image
加载步骤与加载BMP步骤差不多,这里需要注意以下几点
1.需要使用IMG_Init初始化iamge库
2. 使用IMG_Load加载png图片(之前使用SDL_LoadBMP加载BMP)
3.使用IMG_Quit释放image库
以下是完整代码
#include <iostream>
#include <sdl.h>
#include <sdl_image.h>
#include <string>
using namespace std;
const int SCREEN_WIDTH = 640;
const int SCREEN_HEIGHT = 480;
SDL_Surface* loadSurface(std::string path);
SDL_Window* gWindow = nullptr;
SDL_Surface* gScreenSurface = nullptr;
SDL_Surface* gPNGSurface = nullptr;
bool init()
{
bool success = true;
//initialize SDL
if (SDL_Init(SDL_INIT_VIDEO) < 0)
{
printf("SDL_ERROR:%s\n", SDL_GetError());
success = false;
}
else
{
//create window
gWindow = SDL_CreateWindow("SDL load PNG", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, SCREEN_WIDTH, SCREEN_HEIGHT, SDL_WINDOW_SHOWN );
if (gWindow == nullptr)
{
printf("SDL_ERROR:%s\n", SDL_GetError());
success = false;
}
else
{
//initialize PNG loading
int imgFlag = IMG_INIT_PNG;
if (!(IMG_Init(imgFlag) & imgFlag))
{
printf("SDL_IMAGE could not initialize!SDL_IMAGE ERROR: %s\n", IMG_GetError());
success = false;
}
else
{
//get window surface
gScreenSurface = SDL_GetWindowSurface(gWindow);
}
}
}
return success;
}
SDL_Surface* loadSurface(string path)
{
SDL_Surface* optimizedSurface = nullptr;
//load image at specified path
SDL_Surface* loadSurface = IMG_Load(path.c_str());
if (loadSurface == nullptr)
{
printf("load image error: %s\n", IMG_GetError());
}
else
{
//convert surface to screen format
optimizedSurface = SDL_ConvertSurface(loadSurface, gScreenSurface->format, 0);
if (optimizedSurface == nullptr)
{
printf("unable to optimize image: %s\n", SDL_GetError());
}
//get rid of old loaded surface
SDL_FreeSurface(loadSurface);
}
return optimizedSurface;
}
bool loadMedia()
{
//Loading success flag
bool success = true;
//Load PNG surface
gPNGSurface = loadSurface("../picture/loaded.png");
if (gPNGSurface == NULL)
{
printf("Failed to load PNG image!\n");
success = false;
}
return success;
}
void close()
{
SDL_FreeSurface(gPNGSurface);
gPNGSurface = nullptr;
SDL_DestroyWindow(gWindow);
gWindow = nullptr;
IMG_Quit();
SDL_Quit();
}
int main(int argc, char* argv[])
{
if (!init())
{
printf("Failed to initialize!\n");
}
else
{
//Load media
if (!loadMedia())
{
printf("Failed to load media!\n");
}
else
{
//Main loop flag
bool quit = false;
//Event handler
SDL_Event e;
//While application is running
while (!quit)
{
//Handle events on queue
while (SDL_PollEvent(&e) != 0)
{
//User requests quit
if (e.type == SDL_QUIT)
{
quit = true;
}
}
//Apply the PNG image
SDL_BlitSurface(gPNGSurface, NULL, gScreenSurface, NULL);
//Update the surface
SDL_UpdateWindowSurface(gWindow);
}
}
}
//Free resources and close SDL
close();
return 0;
}