一、MD5加密
在python中实现MD5加密是主要是通过hashlib完成的,加密的代码是固定的。是一种不可逆运算,对不同的数据加密的结果是定长的32位和16位字符 python代码的实现如下:
import hashlib
def hasmd5(arg):
# 将数据转换成UTF-8格式
se = hashlib.md5(arg.encode('utf-8'))
# 将hash中的数据转换成只包含十六进制的数字
result = se.hexdigest()
return result
if __name__ == '__main__':
print(hasmd5('123abc'))
>> a906449d5769fa7361d7ecc6aa3f6d28
二、 AES对称加密
在数字加密算法中,通过可划分为对称加密和非对称加密。
在对称加密算法中,加密和解密使用的是同一把钥匙,即:使用相同的密匙对同一密码进行加密和解
密;
加密过程如下:
加密:原文 + 密匙 = 密文
解密:密文 - 密匙 = 原文
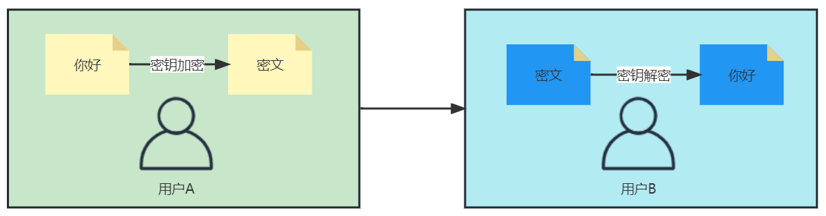
在线加密工具:https://www.lddgo.net/encrypt/aes
pip安装:
pip install pycryptodome
代码实现:
# -*- coding: utf-8 -*-
import base64
from Crypto.Cipher import AES
class EncryptDate:
def __init__(self, key):
self.key = key.encode('utf-8') # 初始化密钥
self.length = AES.block_size # 初始化数据块大小,为16位
self.aes = AES.new(self.key, AES.MODE_ECB) # 初始化AES,ECB模式的实例
self.unpad = lambda date: date[0:-ord(date[-1])] # 截断函数,去除填充的字符
def pad(self, text):
'''
填充函数,使被加密数据的字节码长度是block_size的整数倍
'''
count = len(text.encode('utf-8'))
add = self.length - (count % self.length)
entext = text + (chr(add) * add)
return entext
# 加密函数
def encrypt(self, encrData):
res = self.aes.encrypt(self.pad(encrData).encode('utf-8'))
# Base64是网络上最常见的用于传输8Bit字节码的编码方式之一
msg = str(base64.b64encode(res), encoding='utf-8')
return msg
# 解密函数
def decrypt(self, decrData):
res = base64.decodebytes(decrData.encode('utf-8')) # 转为二进制字节流
msg = self.aes.decrypt(res).decode('utf-8')
return self.unpad(msg) # 把之前为了填充为16位多余的那部分截掉
if __name__ == '__main__':
print("============加密==================")
key = "1234567812345678" # key 密码,服务器指定
data = "tony" # 数据
eg = EncryptDate(key) # 这里密钥的长度必须是16的倍数
res1 = eg.encrypt(data)
print(res1)
print("============解密==================")
res2 = eg.decrypt(res1)
print(res2)
>> ============加密==================
>> XbXHJrNLwoTVcyfqM9eTgQ==
>> ============解密==================
>> tony
三、rsa非对称加密
对称加密是使用的 同一把密匙进行加密和解密。那么,非对称加密自然是使用不同的密钥进行加密和解密啦。 非对称加密有两个钥匙,及公钥(Public Key)和私钥(Private Key)。公钥和私钥是成对的存在,如 果对原文使用公钥加密,则只能使用对应的私钥才能解密;因为加密和解密使用的不是同一把密钥,所 以这种算法称之为非对称加密算法。 非对称加密算法的密匙是通过一系列算法获取到的一长串随机数,通常随机数的长度越长,加密信息越 安全。通过私钥经过一系列算法是可以推导出公钥的,也就是说,公钥是基于私钥而存在的。但是无法 通过公钥反向推倒出私钥,这个过程是单向的。
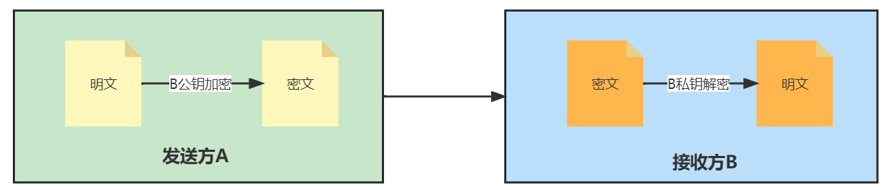
在线加密工具:http://rsa.bchrt.com/
python代码实现:
# -*- coding: utf-8 -*-
import base64
import rsa
# 秘钥的位数, 可以自定义指定, 例如: 128、256、512、1024、2048等
(pubkey, privkey) = rsa.newkeys(512)
# 生成公钥
pub = pubkey.save_pkcs1()
# 将公钥保存到文件中
# with open('public.pem', 'wb') as f:
# f.write(pub)
# 生成私钥
pri = privkey.save_pkcs1()
# 将私钥保存到文件中
# with open('private.pem', 'wb') as f:
# f.write(pri)
class Rsa():
def __init__(self):
self.pub_key = rsa.PublicKey.load_pkcs1(pub)
self.priv_key = rsa.PrivateKey.load_pkcs1(pri)
def encrypt(self, text):
# rsa加密 最后把加密字符串转为base64
text = text.encode("utf-8")
cryto_info = rsa.encrypt(text, self.pub_key)
cipher_base64 = base64.b64encode(cryto_info) # 输出的为byte类型的base64
cipher_base64 = cipher_base64.decode() # 转为str类型的base64
return cipher_base64
def decrypt(self, text):
# rsa解密 返回解密结果
cryto_info = base64.b64decode(text) # 将base64转为二进制byte字节
talk_real = rsa.decrypt(cryto_info, self.priv_key)
res = talk_real.decode("utf-8")
return res
if __name__ == "__main__":
rsaer = Rsa()
info = rsaer.encrypt('测试非对称加密')
print('加密:', info)
print('解密:', rsaer.decrypt(info))
>> 加密: N0sl/zKIM9b3e282XX/7wTDwqnjgTj9yrVwpCgndhw30Z5y7r2RcL1zsajzUt9opznEdb3p9aDe7UVrSGtmy7w==
>> 解密: 测试非对称加密