相信大家在开发的过程中都会遇到在线预览功能,有没有想过如何通过java来实现excel、word、txt、ppt等办公文件在线预览功能?今天我们就来解决这一疑问!
其实,网上还是有些公司对这一功能提供了收费服务。那么,如何实现免费的功能呢?接下来,我们就来免费实现一版本吧。
我们要实现免费,用到的就是openoffice。
openoffice的原理是:通过openoffice将word、ppt、excel、txt这些文件先转化成pdf文件流;
另外,浏览器支持pdf文件预览的话,并且装了Adobe Reader XI,将pdf拖拽到浏览器中是可以直接打来浏览的。
Apache OpenOffice的下载地址:Apache OpenOffice - Official Download
安装包下载完成后,傻瓜式安装运行就可以了。Linux的则百度一下即可。
pom文件中引入依赖
<dependency>
<groupId>com.artofsolving</groupId>
<artifactId>jodconverter</artifactId>
<version>2.2.1</version>
</dependency>
<dependency>
<groupId>org.jodconverter</groupId>
<artifactId>jodconverter-core</artifactId>
<version>4.4.2</version>
</dependency>
<dependency>
<groupId>org.openoffice</groupId>
<artifactId>jurt</artifactId>
<version>3.0.1</version>
</dependency>
<dependency>
<groupId>org.openoffice</groupId>
<artifactId>ridl</artifactId>
<version>3.0.1</version>
</dependency>
<dependency>
<groupId>org.openoffice</groupId>
<artifactId>juh</artifactId>
<version>3.0.1</version>
</dependency>
<dependency>
<groupId>org.openoffice</groupId>
<artifactId>unoil</artifactId>
<version>3.0.1</version>
</dependency>
代码实现
工具类:
/**
* 文件格式转换工具类
*/
public class FileConvertUtil {
/**
* 默认转换后文件后缀
**/
private static final String DEFAULT_SUFFIX = "pdf";
/**
* openoffice_port
*/
private static final Integer OPENOFFICE_PORT = 8100;
/**
* 方法描述 office文档转换为PDF(处理本地文件)
* @param sourcePath 源文件路径
* @param suffix 源文件后缀
* @param ip 链接ip地址
* @return InputStream 转换后文件输入流
*/
public static InputStream convertLocaleFile(String sourcePath, String suffix,String ip) throws Exception {
File inputFile = new File(sourcePath);
InputStream inputStream = new FileInputStream(inputFile);
return covertCommonByStream(inputStream, suffix,ip);
}
/**
* 方法描述 office文档转换为PDF(处理网络文件)
* @param netFileUrl 网络文件路径
* @param suffix 文件后缀
* @param ip 链接ip地址
* @return InputStream 转换后文件输入流
**/
public static InputStream convertNetFile(String netFileUrl, String suffix,String ip) throws Exception {
// 创建URL
URL url = new URL(netFileUrl);
// 试图连接并取得返回状态码
URLConnection urlconn = url.openConnection();
urlconn.connect();
HttpURLConnection httpconn = (HttpURLConnection) urlconn;
int httpResult = httpconn.getResponseCode();
if (httpResult == HttpURLConnection.HTTP_OK) {
InputStream inputStream = urlconn.getInputStream();
return covertCommonByStream(inputStream, suffix,ip);
}
return null;
}
/**
* 方法描述 将文件以流的形式转换
* @param inputStream 源文件输入流
* @param suffix 源文件后缀
* @param ip 链接ip地址
* @return InputStream 转换后文件输入流
*/
public static InputStream covertCommonByStream(InputStream inputStream, String suffix,String ip) throws Exception {
ByteArrayOutputStream out = new ByteArrayOutputStream();
OpenOfficeConnection connection = new SocketOpenOfficeConnection(ip,OPENOFFICE_PORT);
connection.connect();
DocumentConverter converter = new StreamOpenOfficeDocumentConverter(connection);
DefaultDocumentFormatRegistry formatReg = new DefaultDocumentFormatRegistry();
DocumentFormat targetFormat = formatReg.getFormatByFileExtension(DEFAULT_SUFFIX);
DocumentFormat sourceFormat = formatReg.getFormatByFileExtension(suffix);
converter.convert(inputStream, sourceFormat, out, targetFormat);
connection.disconnect();
return outputStreamConvertInputStream(out);
}
/**
* 方法描述 outputStream转inputStream
*/
public static ByteArrayInputStream outputStreamConvertInputStream(final OutputStream out) throws Exception {
ByteArrayOutputStream baos=(ByteArrayOutputStream) out;
return new ByteArrayInputStream(baos.toByteArray());
}
/**
* @Description:系统文件在线预览接口
*/
public void onlinePreview(String url,String ip, HttpServletResponse response) throws BaseException {
if(StringUtils.isEmpty(url)){
throw new ParamException("文件路径不能为空");
}
//获取文件类型
String[] str = url.split("\\.");
if(str.length==0){
throw new ParamException("文件格式不正确");
}
String suffix = str[str.length-1];
if(!suffix.equals("txt") && !suffix.equals("doc") && !suffix.equals("docx") && !suffix.equals("xls")
&& !suffix.equals("xlsx") && !suffix.equals("ppt") && !suffix.equals("pptx")){
throw new ParamException("文件格式不支持预览");
}
InputStream in= null;
OutputStream outputStream = null;
try {
in = convertNetFile(url,suffix,ip);
outputStream = response.getOutputStream();
//创建存放文件内容的数组
byte[] buff =new byte[1024];
//所读取的内容使用n来接收
int n;
//当没有读取完时,继续读取,循环
while((n=in.read(buff))!=-1){
//将字节数组的数据全部写入到输出流中
outputStream.write(buff,0,n);
}
//强制将缓存区的数据进行输出
outputStream.flush();
//关流
outputStream.close();
in.close();
} catch (Exception e) {
e.printStackTrace();
if (outputStream != null) {
//关流
try {
outputStream.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
if(in != null){
try {
in.close();
} catch (IOException e1) {
e1.printStackTrace();
}
}
}
}
在Controller类的接口中调用onlinePreview即可完成在线预览功能,无需任何返回参数,通过输入输出流来实现在线预览。
扫描二维码关注公众号,回复:
14883586 查看本文章
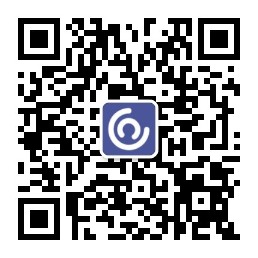
效果图:
注意:如果文件是docx、xlsx、pptx会不支持的!
后续会更文,告诉大家如何才能够支持以上三种文件的预览
欢迎点击下方卡片,关注《coder练习生》