HKEY_CURRENT_USER
HKEY_CURRENT_USER不受权限限制,可直接使用。
可读、可写、可删除
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Microsoft.Win32; namespace RegeditControl { public class RegCurrentUser { public const string InitEnable = "0"; /// <summary> /// 设置键值 /// </summary> /// <param name="bFalse"></param> /// public static void PartEnable(bool bFalse) { try { //Registry.LocalMachine RegistryKey Part = Registry.CurrentUser.OpenSubKey("SOFTWARE\\APP\\Series\\Part", true); if (Part == null) { Create(); Part = Registry.CurrentUser.OpenSubKey("SOFTWARE\\APP\\Series\\Part", true); } if (Part == null) { return; } if (bFalse) Part.SetValue("Enable", "1"); else Part.SetValue("Enable", "0"); Part.Close(); } catch (Exception exp) { System.Windows.Forms.MessageBox.Show(exp.Message); return; } } /// <summary> /// 获取键值 /// </summary> /// <returns></returns> public static bool IsPartEnable() { string value = ""; try { RegistryKey Part = Registry.CurrentUser.OpenSubKey("SOFTWARE\\APP\\Series\\Part", true); if (Part == null) { Create(); Part = Registry.CurrentUser.OpenSubKey("SOFTWARE\\APP\\Series\\Part", true); } if (Part == null) { value = InitEnable; if (value == "0") return false; return true; } // if (Part != null) if (IsRegeditKeyExist(Part, "Enable")) { value = Part.GetValue("Enable").ToString(); } else { if (value == "") Part.SetValue("Enable", InitEnable); value = InitEnable; } Part.Close(); } catch (Exception exp) { System.Windows.Forms.MessageBox.Show(exp.Message); value = InitEnable; } if (value == "0") return false; return true; } /// <summary> /// 创建项与键值 /// </summary> private static void Create() { string value = ""; try { RegistryKey key = Registry.CurrentUser; RegistryKey software = Registry.CurrentUser.OpenSubKey("SOFTWARE", true); if (software == null) software = key.CreateSubKey("SOFTWARE"); RegistryKey app = software.OpenSubKey("APP", true); if (app == null) app = software.CreateSubKey("APP"); RegistryKey series = app.OpenSubKey("Series", true); if (series == null) series = app.CreateSubKey("Series"); RegistryKey part = series.OpenSubKey("Part", true); if (part == null) part = series.CreateSubKey("Part"); if (IsRegeditKeyExist(part, "Enable")) { value = part.GetValue("Enable").ToString(); } else { if (value == "") part.SetValue("Enable", InitEnable); value = InitEnable; } part.Close(); series.Close(); app.Close(); software.Close(); key.Close(); } catch (Exception exp) { System.Windows.Forms.MessageBox.Show(exp.Message); } } /// <summary> /// 判断键值是否存在 /// </summary> /// <param name="RegBoot"></param> /// <param name="RegKeyName"></param> /// <returns></returns> private static bool IsRegeditKeyExist(RegistryKey RegBoot, string RegKeyName) { string[] subkeyNames; subkeyNames = RegBoot.GetValueNames(); foreach (string keyName in subkeyNames) { if (keyName == RegKeyName) //判断键值的名称 { return true; } } return false; } /// <summary> /// 判断项是否存在 /// </summary> /// <param name="RegBoot"></param> /// <param name="ItemName"></param> /// <returns></returns> private static bool IsRegeditItemExist(RegistryKey RegBoot, string ItemName) { if (ItemName.IndexOf("\\") <= -1) { string[] subkeyNames; subkeyNames = RegBoot.GetValueNames(); foreach (string ikeyName in subkeyNames) //遍历整个数组 { if (ikeyName == ItemName) //判断子项的名称 { return true; } } return false; } else { string[] strkeyNames = ItemName.Split('\\'); RegistryKey _newsubRegKey = RegBoot.OpenSubKey(strkeyNames[0]); string _newRegKeyName = ""; int i; for (i = 1; i < strkeyNames.Length; i++) { _newRegKeyName = _newRegKeyName + strkeyNames[i]; if (i != strkeyNames.Length - 1) { _newRegKeyName = _newRegKeyName + "\\"; } } return IsRegeditItemExist(_newsubRegKey, _newRegKeyName); } } } }
HKEY_LOCAL_MACHINE
HKEY_LOCAL_MACHINE在Win7及以上系统受权限限制,需要管理员权限才能实现读、写、删除等操作。
1、只可读
注:
①获取项的读权限:
[assembly: RegistryPermissionAttribute(SecurityAction.RequestMinimum, Read = @"HKEY_LOCAL_MACHINE\SOFTWARE")]
②第二个参数不能填true-写,只能填false:
RegistryKey software = Registry.LocalMachine.OpenSubKey("SOFTWARE\\", false);
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Microsoft.Win32; using System.Security.Permissions; [assembly: RegistryPermissionAttribute(SecurityAction.RequestMinimum, Read = @"HKEY_LOCAL_MACHINE\SOFTWARE")] namespace RegeditControl { /// <summary> /// 只能读取 /// </summary> public class RegLocalMachineOnlyRead { public const string InitEnable = "0"; // [assembly: IsolatedStorageFilePermission(SecurityAction.RequestMinimum, UsageAllowed = IsolatedStorageContainment.AssemblyIsolationByUser)] public static bool IsPartEnable() { try { // RegistryKey key = Registry.LocalMachine; RegistryKey software = Registry.LocalMachine.OpenSubKey("SOFTWARE\\", false); if (software != null) { software.CreateSubKey("APP"); } software.Close(); } catch(Exception exp) { System.Windows.Forms.MessageBox.Show(exp.Message); } return true; } } }
2、可读、可写、可删除
第一步、添加“应用程序清单文件”,并将“app.manifest”文件中的“asInvoker”替换成“requireAdministrator”;
app.manifest
<?xml version="1.0" encoding="utf-8"?> <asmv1:assembly manifestVersion="1.0" xmlns="urn:schemas-microsoft-com:asm.v1" xmlns:asmv1="urn:schemas-microsoft-com:asm.v1" xmlns:asmv2="urn:schemas-microsoft-com:asm.v2" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"> <assemblyIdentity version="1.0.0.0" name="MyApplication.app"/> <trustInfo xmlns="urn:schemas-microsoft-com:asm.v2"> <security> <requestedPrivileges xmlns="urn:schemas-microsoft-com:asm.v3"> <requestedExecutionLevel level="requireAdministrator" uiAccess="false" /> </requestedPrivileges> </security> </trustInfo> <compatibility xmlns="urn:schemas-microsoft-com:compatibility.v1"> <application> </application> </compatibility> </asmv1:assembly>
第二步、将程序改为以管理员方式启动
static void Main() { //获得当前登录的Windows用户标示 System.Security.Principal.WindowsIdentity identity = System.Security.Principal.WindowsIdentity.GetCurrent(); System.Security.Principal.WindowsPrincipal principal = new System.Security.Principal.WindowsPrincipal(identity); //判断当前登录用户是否为管理员 if (principal.IsInRole(System.Security.Principal.WindowsBuiltInRole.Administrator)) { // 修改注册表 //bool benable = RegeditControl.RegLocalMachine.IsPartEnable(); //if (benable) { Application.EnableVisualStyles(); Application.SetCompatibleTextRenderingDefault(false); Application.Run(new Form1()); } } else { //创建启动对象 System.Diagnostics.ProcessStartInfo startInfo = new System.Diagnostics.ProcessStartInfo(); startInfo.UseShellExecute = true; startInfo.WorkingDirectory = Environment.CurrentDirectory; startInfo.FileName = Application.ExecutablePath; //设置启动动作,确保以管理员身份运行 startInfo.Verb = "runas"; try { System.Diagnostics.Process.Start(startInfo); } catch(Exception exp) { System.Windows.Forms.MessageBox.Show(exp.Message); return; } //退出 Application.Exit(); } }
第三步、添加注册表操作接口
*.cs
扫描二维码关注公众号,回复:
1483044 查看本文章
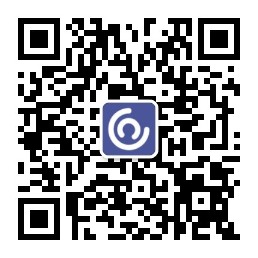
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using Microsoft.Win32; using System.Security.Permissions; namespace RegeditControl { /// <summary> /// 只能读取 /// </summary> public class RegLocalMachine { public const string InitEnable = "0"; /// <summary> /// 设置键值 /// </summary> /// <param name="bFalse"></param> /// public static void PartEnable(bool bFalse) { try { //Registry.LocalMachine RegistryKey Part = Registry.LocalMachine.OpenSubKey("SOFTWARE\\WOW6432Node\\APP\\Series\\Part", true); if (Part == null) { Create(); Part = Registry.LocalMachine.OpenSubKey("SOFTWARE\\WOW6432Node\\APP\\Series\\Part", true); } if (Part == null) { return; } if (bFalse) Part.SetValue("Enable", "1"); else Part.SetValue("Enable", "0"); Part.Close(); } catch (Exception exp) { System.Windows.Forms.MessageBox.Show(exp.Message); return; } } /// <summary> /// 获取键值 /// </summary> /// <returns></returns> public static bool IsPartEnable() { string value = ""; try { RegistryKey Part = Registry.LocalMachine.OpenSubKey("SOFTWARE\\WOW6432Node\\APP\\Series\\Part", true); if (Part == null) { Create(); Part = Registry.LocalMachine.OpenSubKey("SOFTWARE\\WOW6432Node\\APP\\Series\\Part", true); } if (Part == null) { value = InitEnable; if (value == "0") return false; return true; } // if (Part != null) if (IsRegeditKeyExist(Part, "Enable")) { value = Part.GetValue("Enable").ToString(); } else { if (value == "") Part.SetValue("Enable", InitEnable); value = InitEnable; } Part.Close(); } catch (Exception exp) { System.Windows.Forms.MessageBox.Show(exp.Message); value = InitEnable; } if (value == "0") return false; return true; } /// <summary> /// 创建项与键值 /// </summary> private static void Create() { string value = ""; try { RegistryKey key = Registry.LocalMachine; RegistryKey software = Registry.LocalMachine.OpenSubKey("SOFTWARE\\WOW6432Node", true); if (software == null) software = key.CreateSubKey("SOFTWARE"); RegistryKey app = software.OpenSubKey("APP", true); if (app == null) app = software.CreateSubKey("APP"); RegistryKey series = app.OpenSubKey("Series", true); if (series == null) series = app.CreateSubKey("Series"); RegistryKey part = series.OpenSubKey("Part", true); if (part == null) part = series.CreateSubKey("Part"); if (IsRegeditKeyExist(part, "Enable")) { value = part.GetValue("Enable").ToString(); } else { if (value == "") part.SetValue("Enable", InitEnable); value = InitEnable; } part.Close(); series.Close(); app.Close(); software.Close(); key.Close(); } catch (Exception exp) { System.Windows.Forms.MessageBox.Show(exp.Message); } } /// <summary> /// 判断键值是否存在 /// </summary> /// <param name="RegBoot"></param> /// <param name="RegKeyName"></param> /// <returns></returns> private static bool IsRegeditKeyExist(RegistryKey RegBoot, string RegKeyName) { string[] subkeyNames; subkeyNames = RegBoot.GetValueNames(); foreach (string keyName in subkeyNames) { if (keyName == RegKeyName) //判断键值的名称 { return true; } } return false; } /// <summary> /// 判断项是否存在 /// </summary> /// <param name="RegBoot"></param> /// <param name="ItemName"></param> /// <returns></returns> private static bool IsRegeditItemExist(RegistryKey RegBoot, string ItemName) { if (ItemName.IndexOf("\\") <= -1) { string[] subkeyNames; subkeyNames = RegBoot.GetValueNames(); foreach (string ikeyName in subkeyNames) //遍历整个数组 { if (ikeyName == ItemName) //判断子项的名称 { return true; } } return false; } else { string[] strkeyNames = ItemName.Split('\\'); RegistryKey _newsubRegKey = RegBoot.OpenSubKey(strkeyNames[0]); string _newRegKeyName = ""; int i; for (i = 1; i < strkeyNames.Length; i++) { _newRegKeyName = _newRegKeyName + strkeyNames[i]; if (i != strkeyNames.Length - 1) { _newRegKeyName = _newRegKeyName + "\\"; } } return IsRegeditItemExist(_newsubRegKey, _newRegKeyName); } } } }