截图
首先引入recyclerview、cardview、和刷新库refresh-layout-kernel、refresh-header-classics
implementation 'androidx.recyclerview:recyclerview:1.2.0'//添加recyclerView
implementation 'androidx.cardview:cardview:1.0.0'//添加cardView
implementation 'com.scwang.smart:refresh-layout-kernel:2.0.3'//添加刷新库
implementation 'com.scwang.smart:refresh-header-classics:2.0.3'//添加经典刷新头
页面布局
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<com.scwang.smart.refresh.layout.SmartRefreshLayout
android:id="@+id/refreshLayout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/mRecycler"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</com.scwang.smart.refresh.layout.SmartRefreshLayout>
</LinearLayout>
向RecyclerView添加数据
private var page = 1
private var mList: ArrayList<String>? = null
mList = ArrayList()
initData()
//使用Recycler
val layoutManager = StaggeredGridLayoutManager(2,
StaggeredGridLayoutManager.VERTICAL)
mRecycler.layoutManager = layoutManager
val adapter = RecyclerAdapter(mList!!)
mRecycler.adapter = adapter
private fun initData() {
mList!!.add("我的关注")
mList!!.add("通知开关\n\n")
mList!!.add("我的徽章")
mList!!.add("意见反馈\n\n\n")
mList!!.add("我要投稿")
mList!!.add("我的关注")
mList!!.add("通知开关\n\n")
mList!!.add("我的徽章")
mList!!.add("意见反馈\n\n\n")
mList!!.add("我要投稿")
mList!!.add("我的关注")
mList!!.add("通知开关\n\n")
mList!!.add("我的徽章")
mList!!.add("意见反馈\n\n\n")
mList!!.add("我要投稿")
}
//防止item乱跳错位
layoutManager.gapStrategy = StaggeredGridLayoutManager.GAP_HANDLING_NONE
//防止第一页出现空白
mRecycler.addOnScrollListener(object : RecyclerView.OnScrollListener() {
override fun onScrollStateChanged(recyclerView: RecyclerView, newState: Int) {
super.onScrollStateChanged(recyclerView, newState)
layoutManager.invalidateSpanAssignments()
}
})
刷新和加载更多
//刷新
refreshLayout.setOnRefreshListener {
mList!!.clear()
initData()
adapter.notifyDataSetChanged()
refreshLayout.finishRefresh(true);//刷新完成
}
//加载更多
refreshLayout.setOnLoadMoreListener {
page++
initData()
adapter.notifyDataSetChanged()
refreshLayout.finishLoadMore(true)//加载完成
}
RecyclerAdapter代码
class RecyclerAdapter(private val textList: ArrayList<String>) :
RecyclerView.Adapter<RecyclerAdapter.MyViewHolder>() {
override fun onCreateViewHolder(
parent: ViewGroup,
viewType: Int
): MyViewHolder {
val view =
LayoutInflater.from(parent.context).inflate(R.layout.recycler_item, parent, false)
return MyViewHolder(view)
}
override fun getItemCount(): Int = textList.size ?: 0
override fun onBindViewHolder(holder: MyViewHolder, position: Int) {
val textpos = textList[position]
holder.title.text = textpos
holder.itemView.setOnClickListener {
Toast.makeText(holder.itemView.context, "${holder.title.text}", Toast.LENGTH_SHORT)
.show()
}
}
class MyViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val title: TextView = itemView.findViewById(R.id.mine_title)
}
}
recycler_item代码
<?xml version="1.0" encoding="utf-8"?>
<androidx.cardview.widget.CardView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="2.5dp"
android:layout_marginTop="5dp"
android:layout_marginRight="2.5dp"
app:cardBackgroundColor="@color/colorPrimaryDark"
app:cardCornerRadius="8dp"
app:cardElevation="0dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<ImageView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:scaleType="centerCrop"
android:src="@mipmap/ic_launcher" />
<TextView
android:id="@+id/mine_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:padding="5dp"
android:textColor="@android:color/white"
android:textSize="16dp"
tools:ignore="MissingConstraints"
tools:text="测试一下" />
</LinearLayout>
</androidx.cardview.widget.CardView>
App代码
public class App extends Application{
//static 代码段可以防止内存泄露
static {
//设置全局的Header构建器
SmartRefreshLayout.setDefaultRefreshHeaderCreator(new DefaultRefreshHeaderCreator() {
@Override
public RefreshHeader createRefreshHeader(Context context, RefreshLayout layout) {
layout.setPrimaryColorsId(R.color.colorPrimary, android.R.color.white);//全局设置主题颜色
return new ClassicsHeader(context);//.setTimeFormat(new DynamicTimeFormat("更新于 %s"));//指定为经典Header,默认是 贝塞尔雷达Header
}
});
//设置全局的Footer构建器
SmartRefreshLayout.setDefaultRefreshFooterCreator(new DefaultRefreshFooterCreator() {
@Override
public RefreshFooter createRefreshFooter(Context context, RefreshLayout layout) {
//指定为经典Footer,默认是 BallPulseFooter
return new ClassicsFooter(context).setDrawableSize(20);
}
});
}
}
配置文件AndroidManifest.xml中的application标签中引用
android:name=".App"
扫描二维码关注公众号,回复:
14814214 查看本文章
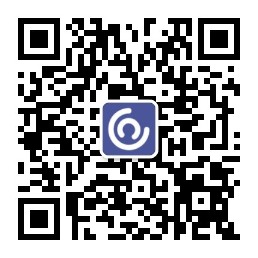