一直听说foreach会有gc产生,有说.net3.5之前有,.net4则修复,一直没有测试,今天测试记录一下
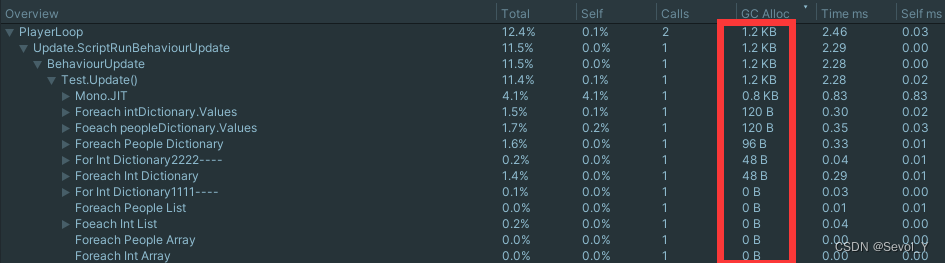
测试在Update中进行:
foreach遍历字典有GC,不过只有第一次有。例如:在update中,两句代码都用foreach遍历同一个字典,则只有第一个foreach有gc产生。
//第一种方法
foreach (var item in peopleDictionary)
{
}
//第二种方法
foreach (KeyValuePair<int, People> keyValuePair in peopleDictionary)
{
}
for循环遍历字典,同样的,如果两句代码遍历同一个字典。也只有第一次有GC产生。可以使用下面代码。
IEnumerator ee = peopleDictionary.GetEnumerator();
ee.MoveNext();
for (int i = 0; i < peopleDictionary.Count; ee.MoveNext(), i++)
{
}
使用foreach循环遍历其中的key或values,则会分别产生额外GC,同样只只产生一次。如下面代码
foreach (var item in peopleDictionary.Values)
{
}
foreach (var item in peopleDictionary.Keys)
{
}
再来一个,每帧都有GC产生的方法,哈哈。
//每帧都有GC产生
IEnumerator ee = peopleDictionary.GetEnumerator();
ee.MoveNext();
for (int i = 0; i < peopleDictionary.Count; ee.MoveNext(), i++)
{
}
//只有一次有GC产生,关键字var
var ee = peopleDictionary.GetEnumerator();
ee.MoveNext();
for (int i = 0; i < peopleDictionary.Count; ee.MoveNext(), i++)
{
}
GC产生的大小,与字典存的数据结构有关,存int的GC 就小于存Class GC。
总结:
遍历List、Array。for循环、 foreach循环、while循环都没有GC。
遍历Dictionary。for循环、 foreach循环,第一次都会产生GC。只有一次也可以放心使用。
最后来一段测试代码,大家可以自行测试
using UnityEngine;
using System.Collections;
using System.Collections.Generic;
using UnityEngine.Profiling;
//using System.Collections.Specialized;
public class People
{
public string Name;
public int Age;
}
public class Test : MonoBehaviour
{
int[] tempsInts = new[] { 1, 3, 4, 5, 6 };
private People[] peoples;
private List<People> peoplesList;
private List<int> intList;
private Dictionary<int, People> peopleDictionary;
private Dictionary<int, int> intDictionary;
private int tem;
// Use this for initialization
void Awake()
{
peoples = new People[5];
peoplesList = new List<People>();
intList = new List<int>();
peopleDictionary = new Dictionary<int, People>();
intDictionary = new Dictionary<int, int>();
for (int i = 0; i < 5; i++)
{
People people = new People();
people.Age = i;
people.Name = ((char)'A' + i).ToString();
peoples[i] = people;
peoplesList.Add(people);
peopleDictionary.Add(i, people);
intDictionary.Add(i, i);
intList.Add(i);
}
}
void Update()
{
Profiler.BeginSample("Foreach Int Dictionary PeoplePeoplePeople");
foreach (KeyValuePair<int, People> keyValuePair in peopleDictionary)
{
}
Profiler.EndSample();
Profiler.BeginSample("Foreach Int Dictionary-2-2-2");
foreach (KeyValuePair<int, People> keyValuePair in peopleDictionary)
{
}
Profiler.EndSample();
Profiler.BeginSample("Foreach Int Dictionary-5-5-5");
foreach (var item in peopleDictionary)
{
}
Profiler.EndSample();
Profiler.BeginSample("For Int Dictionary1111----");
var e = peopleDictionary.GetEnumerator();
e.MoveNext();
for (int i = 0; i < peopleDictionary.Count; e.MoveNext(), i++)
{
}
Profiler.EndSample();
Profiler.BeginSample("For Int Dictionary2222----");
IEnumerator ee = peopleDictionary.GetEnumerator();
ee.MoveNext();
for (int i = 0; i < peopleDictionary.Count; ee.MoveNext(), i++)
{
}
Profiler.EndSample();
Profiler.BeginSample("Foreach Int Dictionary-1-1-1");
foreach (var item in peopleDictionary.Keys)
{
}
Profiler.EndSample();
Profiler.BeginSample("Foreach Int Dictionary000----");
foreach (var item in peopleDictionary.Values)
{
}
Profiler.EndSample();
Profiler.BeginSample("Foreach Int Dictionary999----");
foreach (People item in peopleDictionary.Values)
{
}
Profiler.EndSample();
}
}