synchronized
public class test {
public static void main(String[] args){
MyRunnable mr = new MyRunnable();
Thread t_01 = new Thread(mr);
Thread t_02 = new Thread(mr);
t_01.start();
t_02.start();
}
}
class MyRunnable implements Runnable {
private int ticket = 10;
private Object obj = new Object();
public void run(){
for(int i=0;i<300;i++){
if(ticket>0){
synchronized(obj){
ticket--;
try {
Thread.sleep(1000);
}catch(InterruptedException e){
System.out.println(e);
}
System.out.println("您购买的票剩余:"+ticket+"张");
}
}
}
}
}
同步方法
public class test {
public static void main(String[] args){
MyRunnable mr = new MyRunnable();
Thread t_01 = new Thread(mr);
Thread t_02 = new Thread(mr);
t_01.start();
t_02.start();
}
}
class MyRunnable implements Runnable {
private int ticket = 10;
private Object obj = new Object();
public void run(){
for(int i=0;i<300;i++){
method();
}
}
private synchronized void method(){
if(ticket>0){
ticket--;
try {
Thread.sleep(100);
}catch(InterruptedException e){
System.out.println(e);
}
System.out.println("您购买的票剩余:"+ticket+"张");
}
}
}
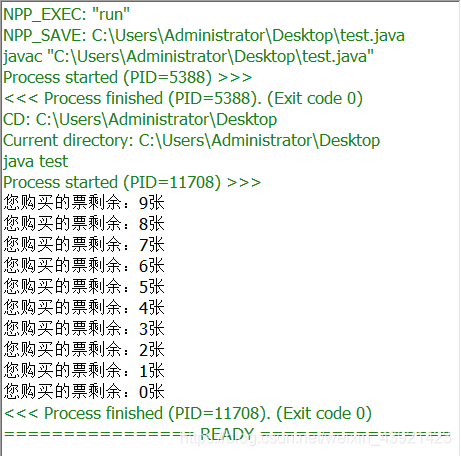
Lock 接口
import java.util.concurrent.locks.ReentrantLock;
public class test {
public static void main(String[] args){
MyRunnable mr = new MyRunnable();
Thread t_01 = new Thread(mr);
Thread t_02 = new Thread(mr);
t_01.start();
t_02.start();
}
}
class MyRunnable implements Runnable {
private int ticket = 10;
private Object obj = new Object();
public void run(){
for(int i=0;i<300;i++){
method();
}
}
ReentrantLock lock = new ReentrantLock();
private void method(){
lock.lock();
try{
if(ticket>0){
ticket--;
try {
Thread.sleep(100);
}catch(InterruptedException e){
System.out.println(e);
}
System.out.println("您购买的票剩余:"+ticket+"张");
}
}finally{
lock.unlock();
}
}
}