uni-app的基本使用
书接上回,课程介绍:
基础部分:
- uni-app中样式学习
- 在uni-app中使用字体图标和开启scss
- 条件注释跨端兼容
- uni中的事件
- 导航跳转
- 组件创建和通讯,及组件的生命周期
- uni-app中使用uni-ui库
uni.removeStorage
从本地缓存中异步移除指定 key。
代码演示
<template>
<view>
<button type="primary" @click="removeStorage">删除数据</button>
</view>
</template>
<script>
export default {
methods: {
removeStorage () {
uni.removeStorage({
key: 'id',
success: function () {
console.log('删除成功')
}
})
}
}
}
</script>
uni.removeStorageSync
从本地缓存中同步移除指定 key。
代码演示
<template>
<view>
<button type="primary" @click="removeStorage">删除数据</button>
</view>
</template>
<script>
export default {
methods: {
removeStorage () {
uni.removeStorageSync('id')
}
}
}
</script>
上传图片、预览图片
上传图片
uni.chooseImage方法从本地相册选择图片或使用相机拍照。
案例代码
<template>
<view>
<button @click="chooseImg" type="primary">上传图片</button>
<view>
<image v-for="item in imgArr" :src="item" :key="index"></image>
</view>
</view>
</template>
<script>
export default {
data () {
return {
imgArr: []
}
},
methods: {
chooseImg () {
uni.chooseImage({
count: 9,
success: res=>{
this.imgArr = res.tempFilePaths
}
})
}
}
}
</script>
预览图片
结构
<view>
<image v-for="item in imgArr" :src="item" @click="previewImg(item)" :key="item"></image>
</view>
预览图片的方法
previewImg (current) {
uni.previewImage({
urls: this.imgArr,
current
})
}
条件注释实现跨段兼容
条件编译是用特殊的注释作为标记,在编译时根据这些特殊的注释,将注释里面的代码编译到不同平台。
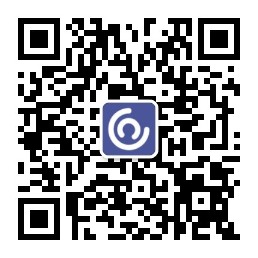
**写法:**以 #ifdef 加平台标识 开头,以 #endif 结尾。
平台标识
值 | 平台 | 参考文档 |
---|---|---|
APP-PLUS | 5+App | HTML5+ 规范 |
H5 | H5 | |
MP-WEIXIN | 微信小程序 | 微信小程序 |
MP-ALIPAY | 支付宝小程序 | 支付宝小程序 |
MP-BAIDU | 百度小程序 | 百度小程序 |
MP-TOUTIAO | 头条小程序 | 头条小程序 |
MP-QQ | QQ小程序 | (目前仅cli版支持) |
MP | 微信小程序/支付宝小程序/百度小程序/头条小程序/QQ小程序 |
组件的条件注释
代码演示
<!-- #ifdef H5 -->
<view>
h5页面会显示
</view>
<!-- #endif -->
<!-- #ifdef MP-WEIXIN -->
<view>
微信小程序会显示
</view>
<!-- #endif -->
<!-- #ifdef APP-PLUS -->
<view>
app会显示
</view>
<!-- #endif -->
api的条件注释
代码演示
onLoad () {
//#ifdef MP-WEIXIN
console.log('微信小程序')
//#endif
//#ifdef H5
console.log('h5页面')
//#endif
}
样式的条件注释
代码演示
/* #ifdef H5 */
view{
height: 100px;
line-height: 100px;
background: red;
}
/* #endif */
/* #ifdef MP-WEIXIN */
view{
height: 100px;
line-height: 100px;
background: green;
}
/* #endif */
uni中的导航跳转
利用navigator进行跳转
navigator详细文档:文档地址
跳转到普通页面
<navigator url="/pages/about/about" hover-class="navigator-hover">
<button type="default">跳转到关于页面</button>
</navigator>
跳转到tabbar页面
<navigator url="/pages/message/message" open-type="switchTab">
<button type="default">跳转到message页面</button>
</navigator>
利用编程式导航进行跳转
利用navigateTo进行导航跳转
保留当前页面,跳转到应用内的某个页面,使用uni.navigateBack
可以返回到原页面。
<button type="primary" @click="goAbout">跳转到关于页面</button>
通过navigateTo方法进行跳转到普通页面
goAbout () {
uni.navigateTo({
url: '/pages/about/about',
})
}
通过switchTab跳转到tabbar页面
跳转到tabbar页面
<button type="primary" @click="goMessage">跳转到message页面</button>
通过switchTab方法进行跳转
goMessage () {
uni.switchTab({
url: '/pages/message/message'
})
}
redirectTo进行跳转
关闭当前页面,跳转到应用内的某个页面。
<!-- template -->
<button type="primary" @click="goMessage">跳转到message页面</button>
<!-- js -->
goMessage () {
uni.switchTab({
url: '/pages/message/message'
})
}
通过onUnload测试当前组件确实卸载
onUnload () {
console.log('组件卸载了')
}
导航跳转传递参数
在导航进行跳转到下一个页面的同时,可以给下一个页面传递相应的参数,接收参数的页面可以通过onLoad生命周期进行接收
传递参数的页面
goAbout () {
uni.navigateTo({
url: '/pages/about/about?id=80',
});
}
接收参数的页面
<script>
export default {
onLoad (options) {
console.log(options)
}
}
</script>
uni-app中组件的创建
在uni-app中,可以通过创建一个后缀名为vue的文件,即创建一个组件成功,其他组件可以将该组件通过impot的方式导入,在通过components进行注册即可
-
创建login组件,在component中创建login目录,然后新建login.vue文件
<template> <view> 这是一个自定义组件 </view> </template> <script> </script> <style> </style>
-
在其他组件中导入该组件并注册
import login from "@/components/test/test.vue"
-
注册组件
components: { test}
-
使用组件
<test></test>
组件的生命周期函数
beforeCreate | 在实例初始化之后被调用。详见 | ||
---|---|---|---|
created | 在实例创建完成后被立即调用。详见 | ||
beforeMount | 在挂载开始之前被调用。详见 | ||
mounted | 挂载到实例上去之后调用。详见 注意:此处并不能确定子组件被全部挂载,如果需要子组件完全挂载之后在执行操作可以使用$nextTick Vue官方文档 |
||
beforeUpdate | 数据更新时调用,发生在虚拟 DOM 打补丁之前。详见 | 仅H5平台支持 | |
updated | 由于数据更改导致的虚拟 DOM 重新渲染和打补丁,在这之后会调用该钩子。详见 | 仅H5平台支持 | |
beforeDestroy | 实例销毁之前调用。在这一步,实例仍然完全可用。详见 | ||
destroyed | Vue 实例销毁后调用。调用后,Vue 实例指示的所有东西都会解绑定,所有的事件监听器会被移除,所有的子实例也会被销毁。详见 |
组件的通讯
父组件给子组件传值
通过props来接受外界传递到组件内部的值
<template>
<view>
这是一个自定义组件 {
{msg}}
</view>
</template>
<script>
export default {
props: ['msg']
}
</script>
<style>
</style>
其他组件在使用login组件的时候传递值
<template>
<view>
<test :msg="msg"></test>
</view>
</template>
<script>
import test from "@/components/test/test.vue"
export default {
data () {
return {
msg: 'hello'
}
},
components: {
test}
}
</script>
子组件给父组件传值
通过$emit触发事件进行传递参数
<template>
<view>
这是一个自定义组件 {
{msg}}
<button type="primary" @click="sendMsg">给父组件传值</button>
</view>
</template>
<script>
export default {
data () {
return {
status: '打篮球'
}
},
props: {
msg: {
type: String,
value: ''
}
},
methods: {
sendMsg () {
this.$emit('myEvent',this.status)
}
}
}
</script>
父组件定义自定义事件并接收参数
<template>
<view>
<test :msg="msg" @myEvent="getMsg"></test>
</view>
</template>
<script>
import test from "@/components/test/test.vue"
export default {
data () {
return {
msg: 'hello'
}
},
methods: {
getMsg (res) {
console.log(res)
}
},
components: {
test}
}
</script>
兄弟组件通讯
uni-ui的使用
1、进入Grid宫格组件
2、使用HBuilderX导入该组件
3、导入该组件
import uniGrid from "@/components/uni-grid/uni-grid.vue"
import uniGridItem from "@/components/uni-grid-item/uni-grid-item.vue"
4、注册组件
components: {uniGrid,uniGridItem}
5、使用组件
<uni-grid :column="3">
<uni-grid-item>
<text class="text">文本</text>
</uni-grid-item>
<uni-grid-item>
<text class="text">文本</text>
</uni-grid-item>
<uni-grid-item>
<text class="text">文本</text>
</uni-grid-item>
</uni-grid>
总结
以上就是本篇文章的全部内容,希望能对大家的学习有所帮助,评论区留下“管用",记得三联哦。 还有其他知识分享,欢迎拜访链接: 首页