1. Bean的配置
Spring 可以被看作是一个大型工厂,这个工厂的作用就是生产和管理 Spring 容器中的Bean。如果想要在项目中使用这个工厂,就需要开发者对Spring 的配置文件进行配置。
Spring 容器支持XML 和 Properties 两种格式的配置文件,在实际开发中,最常使用的就是 对应XML 格式的配置方式。这种配置方式通过XML 文件来注册并管理Bean 之间的依赖关系。接下来本小节将使用XML 文件的形式对Bean的属性和定义进行详细的讲解。
在Spring 中,XML 配置文件的根元素是,中包含了多个子元素,每一个子元素定义了一个Bean,并描述了该Bean 如何被装配到 Spring 容器中。元素中同样包含了多个属性以及子元素,其常用属性及子元素如表所示。
在实际配置中,通常一个普通的Bean只需要定义id和class两个属性即可
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--
bean:配置一个bean对象,将对象交给IOC容器管理
属性:
id:bean的唯一标识,不能重复
class:设置bean对象所对应的类型
-->
<bean id="bean1" class="com.atguigu.spring.Bean1"/>
<bean id="bean2" class="com.atguigu.spring.Bean2"/>
</beans>
如果在Bean中未指定id和name,则Spring会将class当作id来使用
2.Bean的实例化
实例化Bean通常有三种方式,分别为构造器实例化,静态工厂实例化和实例工厂实例化
2.1 构造器实例化
构造器实例化是指Spring容器通过Bean对应类中默认的无参构造方法来实例化Bean。
实体类(Bean)
package com.atguigu.spring.pojo;
public class HelloWorld {
public void sayHello(){
System.out.println("hello,spring");
}
}
对应xml配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<!--
bean:配置一个bean对象,将对象交给IOC容器管理
属性:
id:bean的唯一标识,不能重复
class:设置bean对象所对应的类型
-->
<bean id="helloworld" class="com.atguigu.spring.pojo.HelloWorld"></bean>
</beans>
实际调用
扫描二维码关注公众号,回复:
14703724 查看本文章
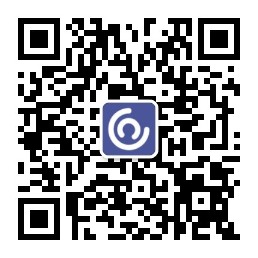
@Test
public void test(){
//获取IOC容器
ApplicationContext ioc = new ClassPathXmlApplicationContext("applicationContext.xml");
//获取IOC容器中的bean
HelloWorld helloworld = (HelloWorld) ioc.getBean("helloworld");
helloworld.sayHello();
}
2.2 静态工厂方式实例化
使用静态工厂是实例化Bean的另一种方式,该方式要求开发者创建一个静态工厂的方法来创建Bean的实例,注意其配置中的class不再是Bean实例的实现类,而是静态工厂类
实体类
public class UserFactoryBean implements FactoryBean<User> {
@Override
public User getObject() throws Exception {
return new User();
}
@Override
public Class<?> getObjectType() {
return User.class;
}
ApplicationContext applicationContext = new FileSystemXmlApplicationContext(String config)
}
xml配置
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean class="com.atguigu.spring.factory.UserFactoryBean"></bean>
</beans>
测试方法
@Test
public void testFactoryBean(){
ApplicationContext ioc = new ClassPathXmlApplicationContext("spring-factory.xml");
User user = ioc.getBean(User.class);
System.out.println(user);
}
2.3 实例工厂方式实例化
该方式与静态工厂方式类似,区别在于不再使用静态方法创建Bean实例,而是采用直接创建的方式,这里不再举例。