1、功能测试
1、阶乘go代码实现。factorial.go
package factorial
func factorial(n int) int {
if n == 0 {
return 1
}
if n == 1 {
return 1
}
return n * factorial(n-1)
}
2、功能测试代码,go测试文件以 _test.go结尾,测试函数以Test开头且Test后面跟大写字母或数子。factorial_test.go
package factorial
import "testing"
func TestFactorial(t *testing.T) {
if factorial(0) != 1 {
t.Error(`factorial(0) != 1`)
}
if factorial(1) != 1 {
t.Error(`factorial(1) != 1`)
}
if factorial(10) != 3628800 {
t.Error(`factorial(10) != 3628800`)
}
}
3、进入到factorial目录,执行 go test 命令
go test
PASS
ok fa.com/wms/test/factorial 0.207s
4、单元测试覆盖率
go test -cover
PASS
coverage: 100.0% of statements
ok fa.com/ms/test/factorial 0.195s
2、性能测试
1、性能测试函数以Benchmark开头。
func BenchmarkFactorial(b *testing.B) {
for i := 0; i < b.N; i++ {
factorial(50)
}
}
2、执行测试函数,结果如下:执行次数13175143,耗时84.47 ns/op,内存消耗0 B/op。性能测试默认测试最小时间1秒。 可以使用-benchtime设置最小执行时间。
go test -bench="." -benchmem
goos: windows
goarch: amd64
pkg: fa.com/ms/test/factorial
cpu: Intel(R) Core(TM) i7-8565U CPU @ 1.80GHz
BenchmarkFactorial-8 13222440 84.99 ns/op 0 B/op 0 allocs/op
PASS
ok fa.com/ms/test/factorial 1.430s
3、性能分析分析工具 go tool pprof与系统性能统计函数pprof.StartCPUProfile( ) pprof.StopCPUProfile(),代码示例如下:
package main
import (
"log"
"os"
"runtime/pprof"
"fa.com/ms/test/factorial"
)
func main() {
c, err := os.Create("D:/cpu_profile.prof")
if err != nil {
log.Fatal(err)
}
defer c.Close()
m, err := os.Create("D:/mem_profile.prof")
if err != nil {
log.Fatal(err)
}
defer m.Close()
// 开始cpu信息采集,并把采集信息写入D:/cpu_profile.prof
pprof.StartCPUProfile(c)
defer pprof.StopCPUProfile()
// 需要分析的功能函数
i := factorial.Factorial(5)
println(i)
// 堆内存分析 写入D:/mem_profile.prof
pprof.Lookup("heap").WriteTo(m, 0)
}
D:/cpu_profile.prof、D:/mem_profile.prof为二进制文件不能直接打开。
需要使用命令打开 go tool pprof D:\cpu_profile.prof:
Type: cpu
Time: Oct 5, 2022 at 9:52am (CST)
Duration: 209.92ms, Total samples = 0
No samples were found with the default sample value type.
Try "sample_index" command to analyze different sample values.
Entering interactive mode (type "help" for commands, "o" for options)
(pprof)
继续输入 top命令:
扫描二维码关注公众号,回复:
14697709 查看本文章
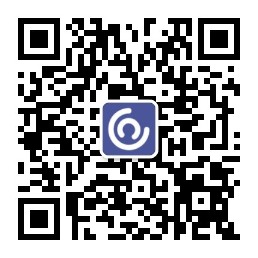
(pprof) top
Showing nodes accounting for 0, 0% of 0 total
flat flat% sum% cum cum%
使用命令打开 go tool pprof D:\cpu_profile.prof
o tool pprof D:\mem_profile.prof
Type: inuse_space
Time: Oct 5, 2022 at 9:52am (CST)
Entering interactive mode (type "help" for commands, "o" for options)
(pprof)
继续输入命令:list main.main
(pprof) list main.main
Total: 2.72MB
ROUTINE ======================== main.main in D:\workspace\main.go
0 1.72MB (flat, cum) 63.24% of Total
. . 19: if err != nil {
. . 20: log.Fatal(err)
. . 21: }
. . 22: defer m.Close()
. . 23:
. 1.72MB 24: pprof.StartCPUProfile(c)
. . 25: defer pprof.StopCPUProfile()
. . 26:
. . 27: i := factorial.Factorial(5)
. . 28: println(i)
. . 29:
(pprof)
更多命令可使用 go tool pprof -help 查看
3、示例测试
示例测试是对文档中的示例代码的测试。示例测试函数以Example开头。
package factorial
import (
"fmt"
)
func ExampleFactorial() {
fmt.Println(Factorial(5))
// Output:
// 120
}