栈区与堆区同为内存中的分区,其中栈区是一种先进后出的结构,由编译器自动分配释放;而堆区容量远大于栈,用于动态内存分配,可有程序员手动分配与释放。
二者在使用过程中有以下注意事项。
1.栈区注意事项:
首先我们做一个实验,写一个函数myFunc() 返回局部变量的地址 定义一个指针变量p接收
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int* myFunc() {
int a = 10;
return &a;
}
void test01() {
int* p = myFunc();
printf("*p=%d\n", *p);
printf("*p=%d\n", *p);
}
int main() {
test01();
system("pause");
return EXIT_SUCCESS;
}
实际上我们不能通过*p获得局部变量a的值,因为局部变量a分配在栈区,会随着函数调用结束而自动释放掉。至于为什么第一次打印可以正确获得a的值,是因为编译器为我们保留了一次,后面再用就不对了。
总结:不要返回局部变量的地址,局部变量在函数执行之后就被释放了,释放的内存就没有权限去操作,如果操作,结果未知!
2. 堆区注意事项
如果函数想要返回局部变量地址,我们可以把局部变量开辟在堆区,但要注意手动释放。
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int* getSpace() {
int * p = (int *)malloc(sizeof(int) * 5); // 堆区开辟4*5个字节 等同于int类型的数组int arr[5]
if (p == NULL) {
printf("分配内存失败");
return;
}
for (int i = 0; i < 5; i++) {
p[i] = i + 100;
}
return p;
}
void test01() {
int* p = getSpace();
for (int i = 0; i < 5; i++) {
printf("%d\n", p[i]);
}
free(p); //释放堆区数据
p = NULL; // 防止出现野指针
}
int main() {
test01();
system("pause");
return EXIT_SUCCESS;
}
程序可以正确执行。
扫描二维码关注公众号,回复:
14671622 查看本文章
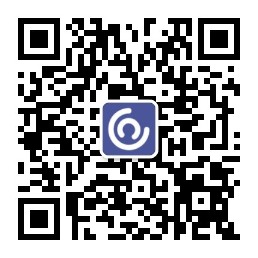
给指针开辟内存:
给定一个开辟内存的函数allocateSpace(),定义一个空指针p,作为函数allocateSpace()的参数
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
void allocateSpace(char *pp) {
char* tmp = malloc(100);//堆区分配100字节
memset(tmp, 0, 100); // 内存置空
strcpy(tmp, "hello world");
pp = tmp;
}
void test02() {
char* p = NULL;
allocateSpace(p);
printf("%s\n", p);
}
int main() {
test02();
system("pause");
return EXIT_SUCCESS;
}
程序运行结果如上。实际上在allocateSpace()函数中,没有改变指针p所对应的内存空间。
注意事项:给指针进行内存开辟时,传入函数中不要用同级的指针,需要传入指针的地址,函数中接收这个地址后在进行内存开辟。
实验代码更改:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
// 注意事项
// 参数为一个二级指针 接收一级指针的地址
void allocateSpace(char **pp) {
char* tmp = malloc(100);//堆区分配100字节
memset(tmp, 0, 100); // 内存置空
strcpy(tmp, "hello world");
*pp = tmp;
}
void test02() {
char* p = NULL;
allocateSpace(&p);
printf("%s\n", p);
}
int main() {
test02();
system("pause");
return EXIT_SUCCESS;
}
程序就可以正确执行了。