2springboot配置文件解析
2.1application.properties配置文件
使用application.properties全局配置文件(位置为src/main/resources目录下或类路径的config下),提供自定义属性支持。
2.1.1自定义属性配置及使用
1)在application.properties文件中添加:
com.dudu.name="test"
com.dudu.want="祝大家鸡年大吉"
2)通过注解使用@Value(value=”${config.name}”)即可绑定
@RestController
public class UserController {
@Value("${com.dudu.name}")
private String name;
@Value("${com.dudu.want}")
private String want;
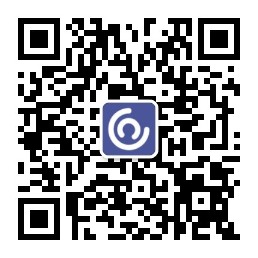
@RequestMapping("/")
public String hexo(){
return name+","+want;
}
}
2.1.2属性过多时绑定对象
1)创建一个ConfigBean.java类,使用注解@ConfigurationProperties(prefix=”com.dudu”)指明使用哪个
@ConfigurationProperties(prefix = "com.dudu")
public class ConfigBean {
private String name;
private String want;
// 省略getter和setter
}
2)还需配置spring boot入口类:
添加@EnableConfigurationProperties并指明加载哪个bean
@SpringBootApplication
@EnableConfigurationProperties({ConfigBean.class})
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
3)最后在controller类中引入configbean使用:
@RestController
public class UserController {
@Autowired
ConfigBean configBean;
@RequestMapping("/")
public String hexo(){
return configBean.getName()+configBean.getWant();
}
}
2.1.3参数间引用
application.properties中的各个参数之间也可以直接引用来使用:
com.dudu.name="test"
com.dudu.want="祝大家鸡年大吉"
com.dudu.yearhope=${com.dudu.name}在此${com.dudu.want}
2.2自定义配置文件
自定义配置文件,避免全部放在application.properties中
1) 新建文件test.properties(路径如src/main/resources),添加配置
com.md.name="哟西~"
com.md.want="祝大家鸡年,大吉吧"
2) 新建bean类
@Configuration
@ConfigurationProperties(prefix = "com.md")
@PropertySource("classpath:test.properties")
public class ConfigTestBean {
private String name;
private String want;
// 省略getter和setter
}
1.5之前的配置可为:
@ConfigurationProperties(prefix = "config2",locations="classpath:test.properties")
2.3随机值配置
配置文件中${random} 可以用来生成各种不同类型的随机值,从而简化了代码生成的麻烦。如生成int 值、long 值或者 string 字符串
dudu.secret=${random.value}
dudu.number=${random.int}
dudu.bignumber=${random.long}
dudu.uuid=${random.uuid}
dudu.number.less.than.ten=${random.int(10)}
dudu.number.in.range=${random.int[1024,65536]}
2.4外部配置(命令行参数配置)
1)Spring boot是基于jar包运行的,可打成jar包并用命令行运行。
Java –jar xx.jar
或修改tomcat端口号:
Java –jar xx.jar –server.port=9090
其中—即对application.properties中属性进行赋值标识。相当于在配置文件中添加server.port=9090.
2)可通过SpringApplication.setAddCommandLineProperties(false)禁用命令行。
3) 配置文件优先级
application.properties和application.yml文件可以放在以下四个位置(按照优先级排序):
外置,在相对于应用程序运行目录的/congfig子目录里。
外置,在应用程序运行的目录里
内置,在config包内
内置,在Classpath根目录
3.1)src/main/resources/config下application.properties覆盖src/main/resources下application.properties中相同的属性
3.2) 在相同优先级位置同时有application.properties和application.yml,那么application.properties里的属性里面的属性就会覆盖application.yml。
2.5多环境配置
2.5.1 profile多环境配置
程序部署到不同运行环境时,配置细节不同(如日志级别,数据源配置)。以往做法是每次发布时替换配置文件(繁琐),而springboot的profile很方便解决该问题。
1) 多环境文件名以application-{profile}.properties的格式命名,{profile}对应你的环境标识,如:
application-dev.properties:开发环境
application-prod.properties:生产环境
2) 使用对应环境,仅需在application.properties中使用spring.profile.active属性设置,值为对应的{profile}标识。
当然也可以使用命令行执行:
java -jar xxx.jar --spring.profiles.active=dev
2.5.2 注解@Profile多环境配置
除了使用profile配置文件分区配置,可注解@Profile配置,如数据库配置:
1)先定义一个接口:
public interface DBConnector { public void configure(); }
2)定义2个实现类:
/**
* 测试数据库
*/
@Component
@Profile("testdb")
public class TestDBConnector implements DBConnector {
@Override
public void configure() {
System.out.println("testdb");
}
}
/**
* 生产数据库
*/
@Component
@Profile("devdb")
public class DevDBConnector implements DBConnector {
@Override
public void configure() {
System.out.println("devdb");
}
}
3)配置文件中配置
spring.profiles.active=testdb
4)使用
@RestController
@RequestMapping("/task")
public class TaskController {
@Autowired DBConnector connector ;
@RequestMapping(value = {"/",""})
public String hellTask(){
connector.configure(); //最终打印testdb
return "hello task !! myage is " + myage;
}
}
除了spring.profiles.active来激活一个或者多个profile之外,还可以用spring.profiles.include来叠加profile:
spring.profiles.active: testdb
spring.profiles.include: proddb,prodmq