Given an array of integers nums
sorted in ascending order, find the starting and ending position of a given target
value.
Your algorithm's runtime complexity must be in the order of O(log n).
If the target is not found in the array, return [-1, -1]
.
Input: nums = [5,7,7,8,8,10], target = 8
Output: [3,4]
Input: nums = [5,7,7,8,8,10], target = 6
Output: [-1,-1]
题意:
给定一个有序数组,找出某个值的起始和终止区间。
思路:
二分查找
扫描二维码关注公众号,回复:
1466623 查看本文章
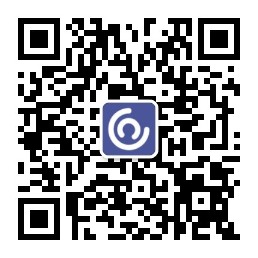
代码:
1 class Solution { 2 public int[] searchRange(int[] nums, int target) { 3 if(nums == null || nums.length == 0) return new int[]{-1,-1}; 4 5 int left = lower_bound(nums, 0, nums.length-1, target); 6 if(left == -1) return new int[]{-1,-1}; 7 int right = upper_bound(nums, 0, nums.length-1, target); 8 return new int[]{left, right}; 9 10 } 11 12 int lower_bound (int[] nums, int left, int right, int target) { 13 while(left + 1 < right){ 14 int mid = left + (right - left)/2; 15 if(nums[mid] < target){ 16 left = mid; 17 }else{ 18 right = mid; 19 } 20 } 21 if (nums[left] == target) return left; 22 if(nums[right] == target) return right; 23 return -1; 24 } 25 26 int upper_bound (int[] nums, int left, int right, int target) { 27 while(left + 1 < right){ 28 int mid = left + (right - left)/2; 29 if(nums[mid] > target){ 30 right = mid; 31 }else{ 32 left = mid; 33 } 34 } 35 if(nums[right] == target) return right; 36 if (nums[left] == target) return left; 37 return -1; 38 } 39 }