前言
- 今天因为工作需求需要清除一份PDF文件上的水印
- 经过查询发现这篇博客但是在使用过程中发现有些方法报错,查阅
PyMuPDF
包官方文档后发现,因版本更新有很多方法写法发生了变化,导致报错
- 这里我在上述博客基础上对函数进行改进,添加了文件夹新建模块、进度条模块。
去除水印
!pip install PyMuPDF
from PIL import Image
from tqdm.notebook import tqdm
from itertools import product
import fitz
import os
def remove_pdf(pdf_file, out_file):
if not os.path.exists(out_file):
os.mkdir(out_file)
page_num = 0
pdf = fitz.open(pdf_file);
for page in tqdm(pdf):
pixmap = page.get_pixmap()
for pos in product(range(pixmap.width), range(pixmap.height)):
rgb = pixmap.pixel(pos[0], pos[1])
if(sum(rgb) > 620):
pixmap.set_pixel(pos[0], pos[1], (255, 255, 255))
pixmap.pil_save(os.path.join(out_file, '%d.png'%page_num))
page_num = page_num + 1
def pic2pdf(pic_dir):
pdf = fitz.open()
img_files = sorted(os.listdir(pic_dir),key=lambda x:int(str(x).split('.')[0]))
for img in tqdm(img_files):
imgdoc = fitz.open(pic_dir + '/' + img)
pdfbytes = imgdoc.convert_to_pdf()
imgpdf = fitz.open("pdf", pdfbytes)
pdf.insert_pdf(imgpdf)
pdf.save("demo.pdf")
pdf.close()
pdf_file = '../input/pdfcheck/CET4.pdf'
out_file = './temp'
remove_pdf(pdf_file, out_file)
pic2pdf(out_file)
- 效果展示
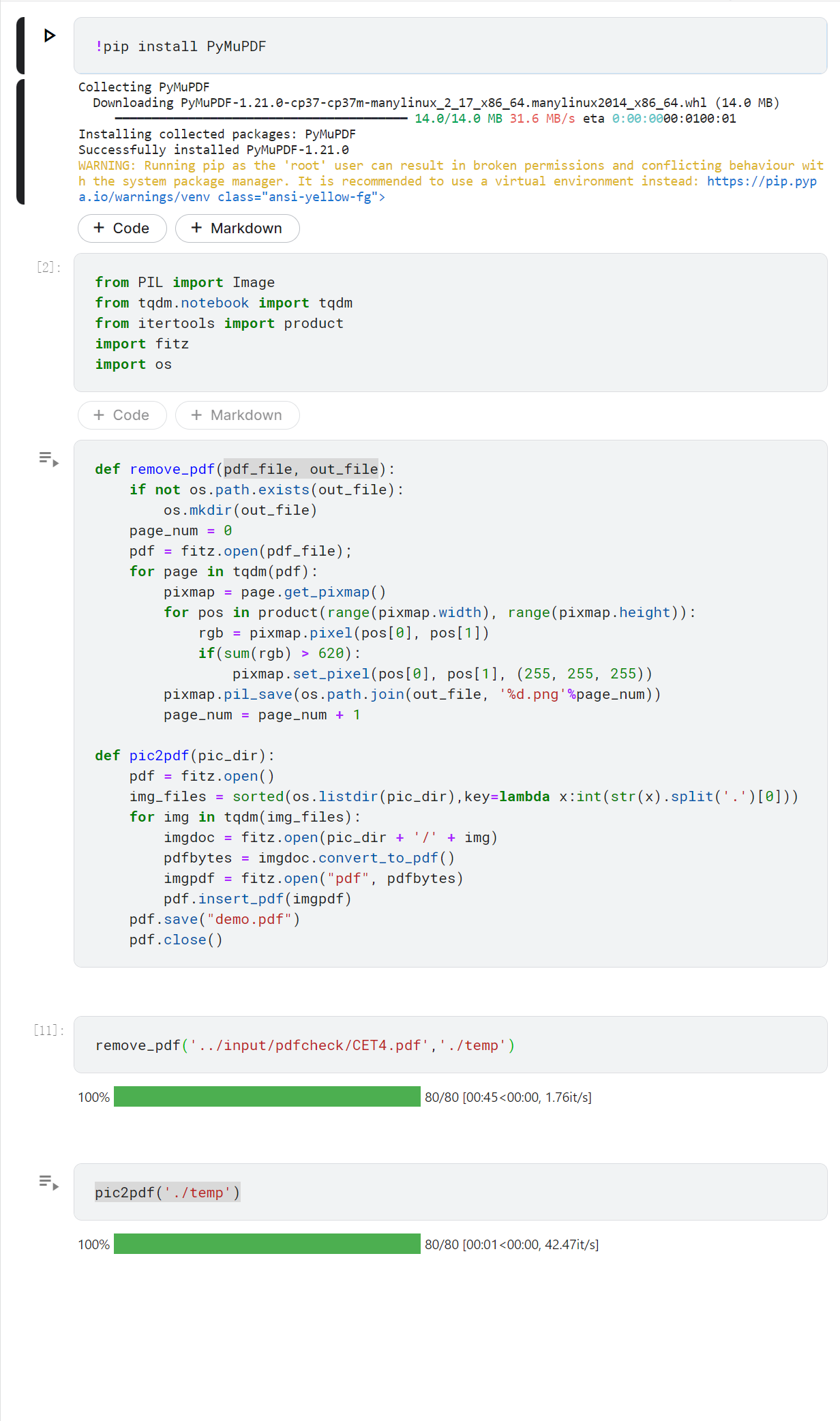
后记
- 有个小问题就是再对彩色PDF进行转换时,对图像质量可能会有影响,这个我也不清楚怎么调节一下,建议先用PDF软件把PDF转换为图片后再进行水印去除,对应的图片去水印函数如下:
def remove_img(in_file, out_file):
if not os.path.exists(out_file):
os.mkdir(out_file)
count = 0
img_files = sorted(os.listdir(in_file),key=lambda x:int((str(x).split('_')[1]).split('.')[0]))
for image_file in tqdm(img_files):
img = Image.open(os.path.join(in_file, image_file))
width, height = img.size
for pos in product(range(width), range(height)):
rgb = img.getpixel(pos)[:3]
if(sum(rgb) >= 620):
img.putpixel(pos, (255, 255, 255))
img.save(os.path.join(out_file, '%d.png'%count))
count = count + 1