描述
给定一个单链表的头结点pHead(该头节点是有值的,比如在下图,它的val是1),长度为n,反转该链表后,返回新链表的表头。
数据范围: 0≤n≤10000
要求:空间复杂度 O(1) ,时间复杂度O(n) 。
如当输入链表{1,2,3}时,
经反转后,原链表变为{3,2,1},所以对应的输出为{3,2,1}。
以上转换过程如下图所示:
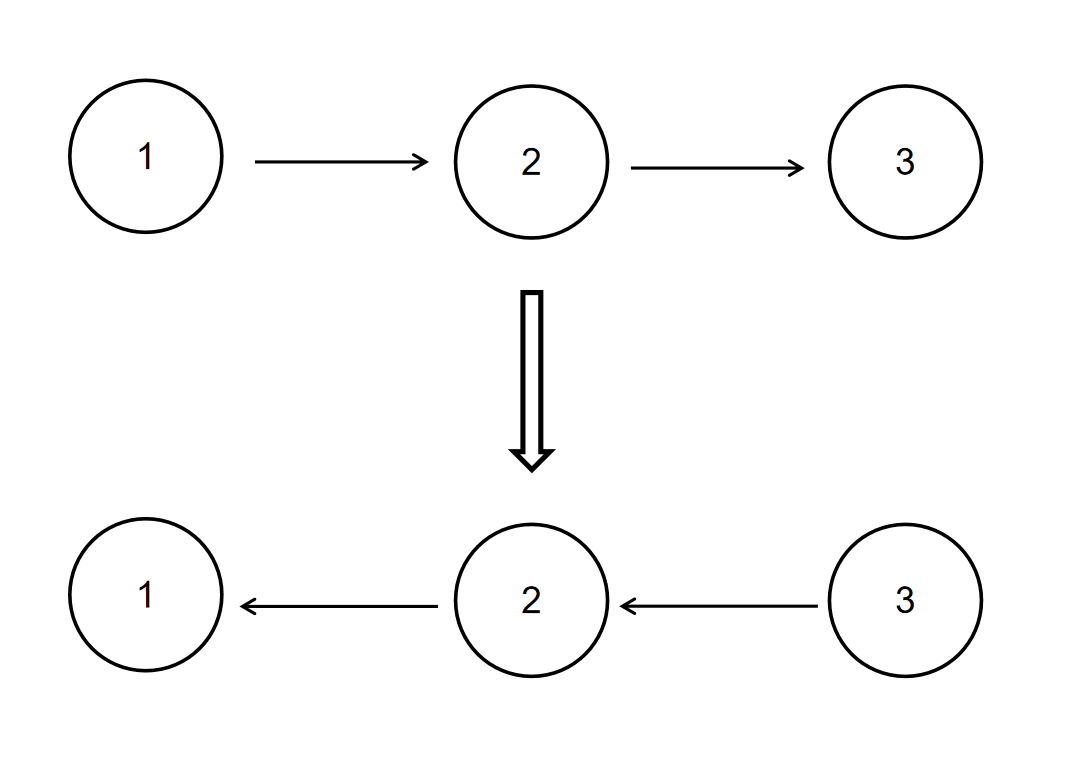
示例1
输入:
{1,2,3}
复制
返回值:
{3,2,1}
复制
示例2
输入:
{}
复制
返回值:
{}
复制
说明:
空链表则输出空
代码实现:
1.正确示例
#include<iostream>
using namespace std;
struct ListNode {
int val;
struct ListNode* next;
ListNode(int x) :
val(x), next(NULL) {
}
};
class Solution {
public:
ListNode* ReverseList(ListNode* pHead) {
//新链表
ListNode* newHead = NULL;
ListNode* temp = NULL;
while (pHead != NULL) {
//反转操作
temp = pHead;
pHead = pHead->next;//pHead指向原链表的下一个单元
temp->next = newHead;
//*important!!!:使用指针之后,两个不同名的指针指向的是同一块地址空间,所以两者的改变都会对该结构体变量产生作用*
newHead = temp;//temp作为新的表头
//更新操作
}
//返回新链表
return newHead;
}
};
int main() {
//创建链表
ListNode* head = new ListNode(1);
head->next = new ListNode(2);
head->next->next = new ListNode(3);
head->next->next->next = new ListNode(4);
head->next->next->next->next = new ListNode(5);
//打印原始链表
ListNode* cur = head;
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
//调用函数进行翻转
Solution s;
ListNode* newHead = s.ReverseList(head);
//打印翻转链表
while (newHead != NULL) {
cout << newHead->val << " ";
newHead = newHead->next;
}
cout << endl;
return 0;
}
2.错误示例:
*important!!!:使用指针之后,两个不同名的指针指向的是同一块地址空间,所以两者的改变都会对该结构体变量产生作用*
#include<iostream>
using namespace std;
struct ListNode {
int val;
struct ListNode* next;
ListNode(int x) :
val(x), next(NULL) {
}
};
class Solution {
public:
ListNode* ReverseList(ListNode* pHead) {
//新链表
ListNode *temp = NULL;
ListNode *newHead = NULL;
while (pHead->next != NULL) {
//反转操作
newHead = pHead;
newHead->next = temp; /*注意:此时,newHead和pHead同时指向同一块地址空间,newHead->next赋值等同于同时对Phead->next进行赋值*/
//*important!!!:使用指针之后,两个不同名的指针指向的是同一块地址空间,所以两者的改变都会对该结构体变量产生作用*
//更新操作
temp = newHead;//更新temp(每次更新为新链表的头部)
// cout<<"Phead->next->val:"<<pHead->next->val;
pHead = pHead->next;//原始链表后移
// cout<<"Phead:"<<pHead->val;
}
//返回新链表
return newHead;
}
};
int main() {
//创建链表
ListNode* head = new ListNode(1);
head->next = new ListNode(2);
head->next->next = new ListNode(3);
head->next->next->next = new ListNode(4);
head->next->next->next->next = new ListNode(5);
//打印原始链表
ListNode* cur = head;
while (cur != NULL) {
cout << cur->val << " ";
cur = cur->next;
}
cout << endl;
//调用函数进行翻转
Solution s;
ListNode* newHead = s.ReverseList(head);
//打印翻转链表
while (newHead != NULL) {
cout << newHead->val << " ";
newHead = newHead->next;
}
cout << endl;
return 0;
}