参考内容
回调函数
- 回调函数就是一个通过函数指针调用的函数。
- 如果你把函数的指针(地址)作为参数传递给另一个函数,当这个指针被用来调用其所指向的函数时,我们就说这是回调函数
- 把一段可执行的代码像参数传递那样传给其他代码,而这段代码会在某个时刻被调用执行,这就叫做回调。如果代码立即被执行就称为同步回调,如果在之后晚点的某个时间再执行,则称之为异步回调
- C 语言回调函数详解 | 菜鸟教程
#include<stdio.h>
int Callback_1() // Callback Function 1
{
printf("Hello, this is Callback_1 \n");
return 0;
}
int Callback_2() // Callback Function 2
{
printf("Hello, this is Callback_2 \n");
return 0;
}
int Callback_3() // Callback Function 3
{
printf("Hello, this is Callback_3 \n");
return 0;
}
int Handle(int (*Callback)()) //函数指针
{
printf("Entering Handle Function. \n");
Callback();
printf("Leaving Handle Function. \n");
}
int main()
{
printf("Entering Main Function. \n");
Handle(Callback_1);
Handle(Callback_2);
Handle(Callback_3);
printf("Leaving Main Function. \n");
return 0;
}
/tmp/tmp.BI8jCkdUAx/cmake-build-debug/openssl-demo
Entering Main Function.
Entering Handle Function.
Hello, this is Callback_1
Leaving Handle Function.
Entering Handle Function.
Hello, this is Callback_2
Leaving Handle Function.
Entering Handle Function.
Hello, this is Callback_3
Leaving Handle Function.
Leaving Main Function.
进程已结束,退出代码0
例子
- Openssl 中大量用到了回调函数。回调函数一般定义在数据结构中,是一个函数指针。 通过回调函数,客户可以自行编写函数,让 openssl 函数来调用它,即用户调用 openssl 提供 的函数,openssl 函数再回调用户提供的函数。这样方便了用户对 openssl函数操作的控制。 在 openssl 实现函数中,它一般会实现一个默认的函数来进行处理,如果用户不设置回调函数,则采用它默认的函数。
- 本例子用来生产简单的随机数,如果用户提供了生成随机数回调函数,则生成随机数采用用户的方法,否则采用默认的方法
openssl-test.h
#ifndef OPENSSL_DEMO_OPENSSL_TEST_H
#define OPENSSL_DEMO_OPENSSL_TEST_H
typedef int *callback_random(char* random,int len);
void set_callback(callback_random *cb);
int generate_random(char* random,int len);
#endif //OPENSSL_DEMO_OPENSSL_TEST_H
openssl-test.cpp
#include "openssl-test.h"
#include <cstdio>
#include <cstring>
callback_random *cb_rand = nullptr;
static int default_random(char* random,int len){
memset(random,0x01,len);
}
void set_callback(callback_random *cb){
cb_rand = cb;
}
int generate_random(char* random,int len){
if (cb_rand == nullptr){
return default_random(random,len);
} else{
return *cb_rand(random,len);
}
}
main.cpp
#include <cstring>
#include <cstdio>
#include "openssl-test.h"
static int * my_rand(char* rand, int len){
memset(rand,0x02,len);
}
int main()
{
char random[10];
int ret = 0;
/*
* 如果注释掉 set_callback ,打印输出的数据均为 1,即没有使用自定义的函数进行回调
* 如果用户自定义随机数函数,就会使用用户自定义的函数,而不是默认的函数
*/
// set_callback(my_rand);
ret = generate_random(random,10);
for (int i = 0; i < 10; ++i) {
printf("%d ",random[i]);
}
return 0;
}
CMakeLists.txt
cmake_minimum_required(VERSION 3.15)
project(openssl-demo)
set(CMAKE_CXX_STANDARD 11)
# 忽略警告
set(CMAKE_CXX_FLAGS "-Wno-error=deprecated-declarations -Wno-deprecated-declarations ")
# 指定lib目录
link_directories(/usr/local/gmssl/lib)
# 指定头文件搜索策略
include_directories(/usr/local/gmssl/include)
# 使用指定的源文件来生成目标可执行文件
add_executable(${PROJECT_NAME} main.cpp openssl-test.h openssl-test.cpp)
# 将库链接到项目中
target_link_libraries(${PROJECT_NAME} ssl crypto pthread dl)
执行结果
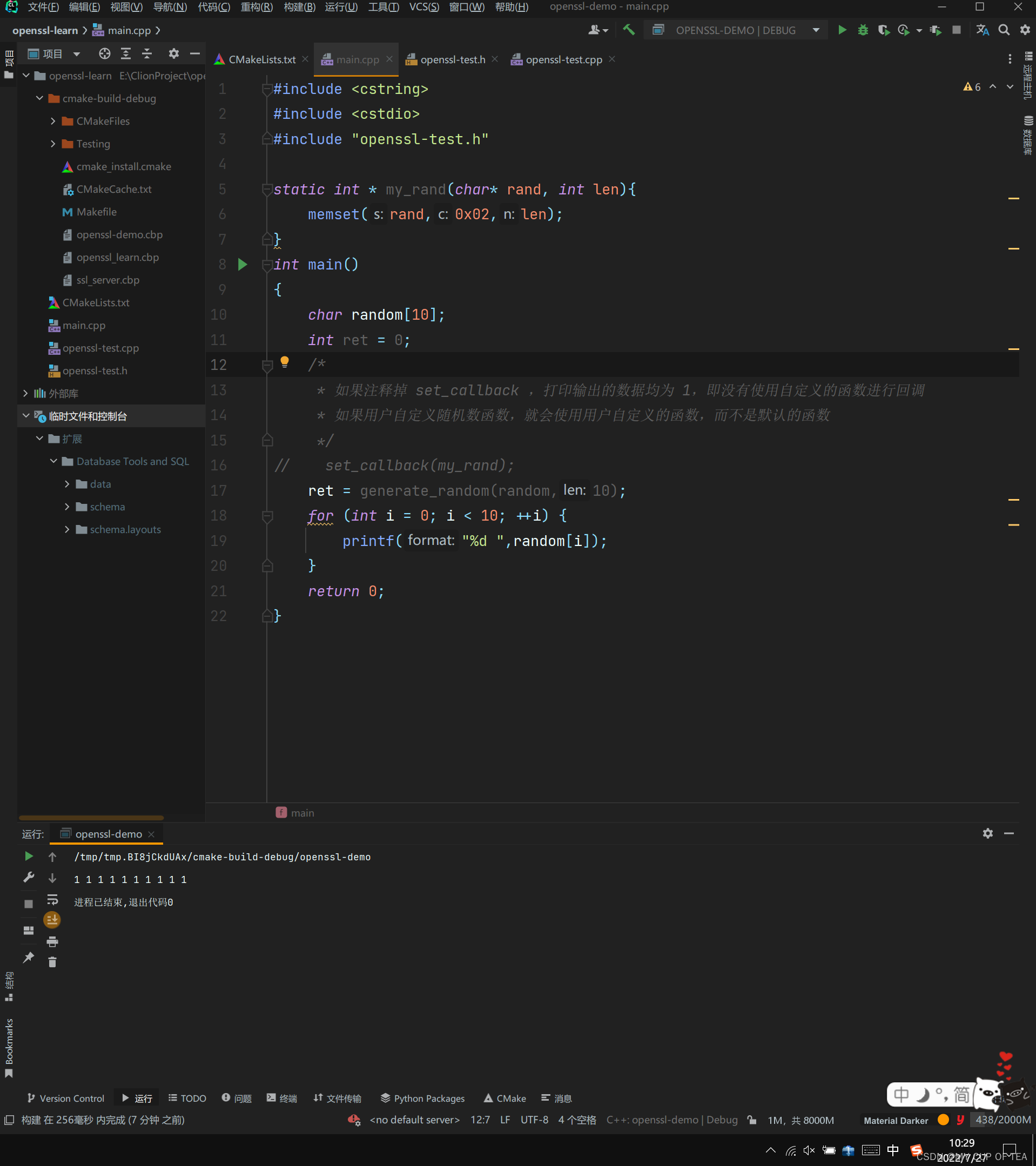