1.文件操作需要包含头文件fstream
2.文件操作分两种
- 文本文件:ASCII码
- 二进制文件:一般人读不懂
3.操作三大类
ofstream
:写操作
ifstream
:读操作
fstream
:读写写操作
4. 打开方式:
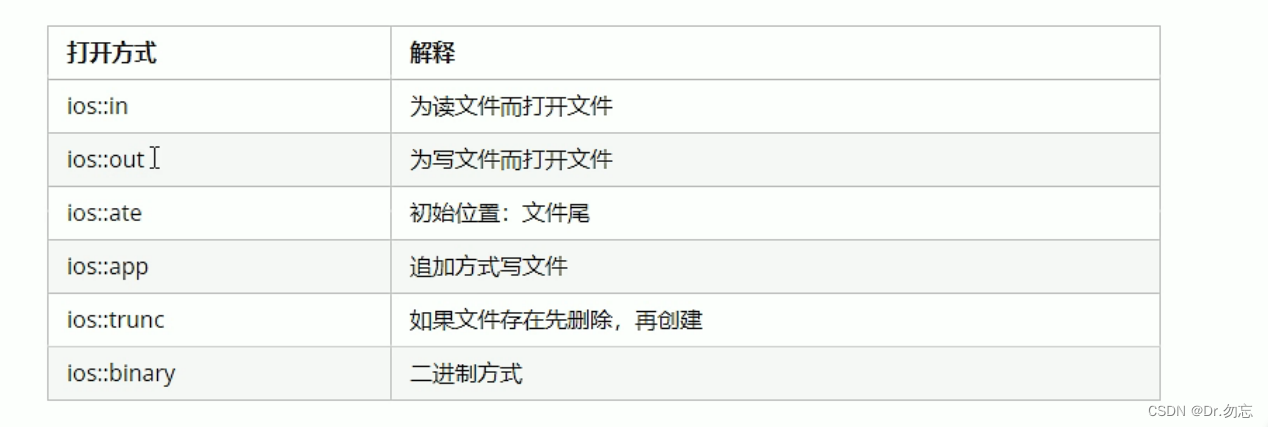
举例
1. 写文件举例
- 包含头文件
fstream
- 创建流对象
- 打开文件
- 写数据
- 关闭文件
#include<iostream>
using namespace std;
#include<string>
#include<fstream>
int main()
{
ofstream f;
f.open("D://1.txt", ios::out);
f << "这是文件";
f.close();
}
2. 读文件举例
- 包含头文件
fstream
- 创建流对象
- 打开文件
- 读数据
- 关闭文件
如果乱码:中文文档用 将文件另存为,编码方式选择ANSI,并覆盖原来的文件
#include<iostream>
using namespace std;
#include<string>
#include<fstream>
int main()
{
ifstream f;
f.open("D://1.txt", ios::in);
cout << "\n方式4" << endl;
char c;
while( (c = f.get()) != EOF )
{
cout << c;
}
f.close();
}
3. 二进制写
- 写binary
- 数据对象,write写入

#include<iostream>
using namespace std;
#include<string>
#include<fstream>
class Person
{
public:
char name[1024];
int age;
};
int main()
{
ofstream f;
f.open("D://1.txt", ios::out | ios::binary);
Person p{
"张三",19};
f.write( (const char *)&p, sizeof(Person) );
f.close();
}
4. 二进制读

#include<iostream>
using namespace std;
#include<string>
#include<fstream>
class Person
{
public:
char name[1024];
int age;
};
int main()
{
ifstream f;
f.open("D://1.txt", ios::in | ios::binary);
Person p;
f.read( (char *)&p, sizeof(Person) );
cout << p.age << ' ' << p.name <<endl;
f.close();
}