1.Digit count
计算位数
Given a number n, you are required to output how many digits are there.
There are several test cases for each test group.
Input: Test case count T following by each test case. Example:
2
12
120
Output:
2
3
import java.util.Scanner;
public class Main{
public static void main(String[] args){
Scanner s = new Scanner(System.in);
int a = s.nextInt();
for(int i=0; i<a; i++){
String str = s.next();
int count = str.length();
for(int j=0; j<count; j++){
if(str.charAt(j)=='0'||str.charAt(j)=='-') count--;
else break;
}
if(count==0) count++;
if(count==5) count=1;
System.out.println(count);
}
s.close();
}
}
2.Unique Digits
读取出现的数字,唯一输出
Given a number n, you are required to output its unique digits in appearing order. There are several test cases for each test group.
Input: Test case count T following by each test case, preceding zeros may exist. Example:
5
1212
120
0121
0000
-23
Output:
12
120
012
0
23
import java.util.*;
public class Main{
public static void main(String[] args){
Scanner s = new Scanner(System.in);
int a = s.nextInt();
for(int i=0; i<a; i++){
String str = s.next();
char arr[] = str.toCharArray();
int j = 0;
if(arr[0] == '-') j = 1;
for( ; j<arr.length; j++){
int m = 0;
for(int k=0; k<j; k++){
if(arr[k] == arr[j]){
m = 1;
break;
}
}
if(m == 0) System.out.print(arr[j]);
}
System.out.print("\n");
}
s.close();
}
}
3.Sum of digits
计算所有数字的和
Given a number n, you are required to output the sum of its digits. There are several test cases for each test group.
Input: Test case count T following by each test case. Example:
5
1211
1234
012
1111
-23
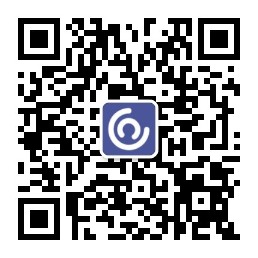
Output:
5
10
3
4
5
import java.util.*;
public class Main{
public static void main(String[] args){
Scanner s = new Scanner(System.in);
int a = s.nextInt();
for(int i=0; i<a; i++){
String str = s.next();
char arr[] = str.toCharArray();
int j = 0;
if(arr[0] == '-') j = 1;
int sum = 0;
for( ; j<arr.length; j++){
String stringj = String.valueOf(arr[j]);
int m = Integer.parseInt(stringj);
sum += m;
}
System.out.println(sum);
}
s.close();
}
}
4.String Length
Your are to output the length of a string. There are several test cases for each test group.
Input: Test case count T following by each test case. Example:
4
asdf
avc asd as
00100
Output:
4
8
10
5
利用scanner的nextLine直接读取下一行为字符串,然后求取长度
import java.util.Scanner;
public class Main {
public static void main(String args[]) {
Scanner s = new Scanner(System.in);
int n = s.nextInt();
s.nextLine();
for(int i = 0; i < n; i++) {
String str = s.nextLine();
System.out.println(str.length());
}
}
}
5.foo bar baz
You are given two numbers "from"(inclusive) and "to"(inclusive). And you are expected to create an application that loops from "from" to "to" and prints out each value on a separate line, except print out "foo" for every multiple of 3, "bar" for every multiple of 5, and "baz" for every multiple of 7.
If "From" is greater than "to", then output a blank line.
For example: if from=1 and to=16, then it will give the following output:
1
2
3 foo
4
5 bar
6 foo
7 baz
8
9 foo
10 bar
11
12 foo
13
14 baz
15 foo bar
16
Input: Total test case count T following by T cases of test instances. Example:
3
4 5
6 10
3 -1
output:
4
5 bar
6 foo
7 baz
8
9 foo
10 bar
这个问题比较难理解,第二行开始输入的是一个一个正向的数据区间,输出需要打印这些区间的数,并且3的倍数输出foo,5的倍数输出bar,7的倍数输出baz,如果遇到左边的数大于右边的数,则这个区间无效不输出,利用nextInt判断接下来是否有输入
import java.util.*;
public class Main{
public static void main(String[] args){
Scanner s = new Scanner(System.in);
int a = s.nextInt();
for(int i=0; i<a; i++){
int m = s.nextInt();
int n = s.nextInt();
if(n<m){
System.out.println();
continue;
}
for(int j = m; j <= n; j++){
System.out.print(j);
if(j%3 == 0) System.out.print(" foo");
if(j%5 == 0) System.out.print(" bar");
if(j%7 == 0) System.out.print(" baz");
System.out.println();
}
}
s.close();
}
}
6.Version 1: No Information Hiding
In this version of the Vehicle class, you will leave the attributes public so that the test program TestVehicle1 will have direct access to them.
- Create a class Vehicle that implements the above UML diagram.
- Include two public attributes: load "the current weight of the vehicle's cargo" and maxLoad "the vehicle's maximum cargo weight limit".
- Include one public constructor to set the maxLoad attribute.
- Include two public access methods: getLoad to retrieve the load attribute and getMaxLoad to retrieve the maxLoad attribute.
Note that all of the data are assumed to be in kilograms.
- The test program Main.java is preset. So just submit your Vehicle class. If everything is ok, The output will be as expected. You can read the test program. Notice that the program gets into trouble when the last box is added to the vehicle's load because the code does not check if adding this box will exceed the maxLoad.
- You can get the test code and run the Main class locally. The output generated should be:
Creating a vehicle with a 10,000kg maximum load. Add box #1 (500kg) Add box #2 (250kg) Add box #3 (5000kg) Add box #4 (4000kg) Add box #5 (300kg) Vehicle load is 10050.0 kg
- 前置代码
/* PRESET CODE BEGIN - NEVER TOUCH CODE BELOW */ public class Main { public static void main(String[] args) { // Create a vehicle that can handle 10,000 kilograms weight System.out.println("Creating a vehicle with a 10,000kg maximum load."); Vehicle vehicle = new Vehicle(10000.0); // Add a few boxes System.out.println("Add box #1 (500kg)"); vehicle.load = vehicle.load + 500.0; System.out.println("Add box #2 (250kg)"); vehicle.load = vehicle.load + 250.0; System.out.println("Add box #3 (5000kg)"); vehicle.load = vehicle.load + 5000.0; System.out.println("Add box #4 (4000kg)"); vehicle.load = vehicle.load + 4000.0; System.out.println("Add box #5 (300kg)"); vehicle.load = vehicle.load + 300.0; // Print out the final vehicle load System.out.println("Vehicle load is " + vehicle.getLoad() + " kg"); } } /* PRESET CODE END - NEVER TOUCH CODE ABOVE */
读取题目可知,只需编写一个名叫Vehicle的class部分即可,分别定义公共的load和maxload,然后按照要求构造函数或则是方法获取调用他们
class Vehicle { double load; double maxLoad; public double getLoad(){ return load; } public double getMaxLoad() { return maxLoad; } public Vehicle(double max_load){ maxLoad = max_load; } }
Version 2: Basic Information Hiding
To solve the problem from the first version, you will hide the internal classdata (load and maxLoad) and provide a method, addBox, to perform the proper checking that the vehicle is not being overloaded.
- Create a class Vehicle that implements the above UML diagram.
You may wish to copy the Vehicle.java file you created in version #1.
- Modify the load and maxLoad attributes to be private.
- Add the addBox method. This method takes a single argument, which is the weight of the box in kilograms. The method must verify that adding the box will not violate the maximum load. If a violation occurs the box is rejected by returning the value of false; otherwise the weight of the box is added to the vehicle load and the method returns true.
Hint: you will need to use an "if" statement. Here is the basic form of the conditional form:
if ( <boolean_expression> ) { <statement>* } else { <statement>* }
Note that all of the data are assumed to be in kilograms.
- Read the Main.java code. Notice that the code can not modify the load attribute directly, but now must use the addBox method. This method returns a true or false value which is printed to the screen.
- Compile the Vehicle and Main classes locally.
- Run the Main class. The output generated should be something like these:
Creating a vehicle with a 10,000kg maximum load. Add box #1 (500kg) : true Add box #2 (250kg) : true Add box #3 (5000kg) : true Add box #4 (4000kg) : true Add box #5 (300kg) : false Vehicle load is 9750.0 kg
类似上一个问题,注意公共和私有变化,增加一个是否满载的判断函数
class Vehicle {
private double load;
private double maxLoad;
public double getLoad(){
return load;
}
public double getMaxLoad() {
return maxLoad;
}
public Vehicle(double max_load){
maxLoad = max_load;
}
public boolean addBox(double weight){
if((load+weight)>getMaxLoad()) return false;
else{
load += weight;
return true;
}
}
}
Version 3: Change Internal Representation of Weight to Newtons
Now suppose that you were going to write some calculations that determine the wear on the vehicle's engine and frame. These calculations are easier if the weight of the load is measured in newtons.
- Create a class Vehicle that implements the above UML diagram.
You may wish to copy the Vehicle.java file you created in version #2.
- Modify the constructor, getLoad, getMaxLoad, and addBox methods to use a conversion from kilograms (the parameter weight measurement) to newtons (the instance variable measurement). You might want to use the following private methods:
private double kiloToNewts(double weight) { return (weight * 9.8); } private double newtsToKilo(double weight) { return (weight / 9.8); }
Note that now the internal data of the vehicle objects is in newtons and the external data (passed between methods) is still in kilograms.
- Modify the constructor, getLoad, getMaxLoad, and addBox methods to use a conversion from kilograms (the parameter weight measurement) to newtons (the instance variable measurement). You might want to use the following private methods:
- Read the preset Main.java code. Notice that it is identical to the test code in version #2.
- Compile the Vehicle and Main classes locally.
- Run the Main class. The output generated should be:
Creating a vehicle with a 10,000kg maximum load. Add box #1 (500kg) : true Add box #2 (250kg) : true Add box #3 (5000kg) : true Add box #4 (4000kg) : true Add box #5 (300kg) : false Vehicle load is 9750.0 kg
根据题意增加单位转换函数即可
class Vehicle { private double load; private double maxLoad; public double getLoad(){ return load; } public double getMaxLoad() { return maxLoad; } public Vehicle(double max_load){ maxLoad = max_load; } public boolean addBox(double weight){ if((load+weight)>getMaxLoad()) return false; else{ load += weight; return true; } } private double kiloToNewts(double weight){ return (weight * 9.8); } private double newtsToKilo(double weight){ return (weight / 9.8); } }
7.Create a Simple Banking Package
与前面的三个version很相似,观察图像和描述,理解题意,编写class Account即可, 设置方法读取每一个balance,构造getBalance读取初值,deposit函数存入(加入),withdraw取出(减去)
- Create the banking directory. Use a command such as:
mkdir banking
under the command line prompt. - Create the Account class in the file Account.java under the banking directory. This class must implement the model in the above UML diagram.
- declare one private object attribute: balance; this attribute will hold the current (or "running") balance of the bank account
- declare a public constructor that takes one parameter (init_balance); that populates the balance attribute
- declare a public method getBalance that retrieves the current balance
- declare a public method deposit that adds the amount parameter to the current balance
- declare a public method withdraw that removes the amount parameter from the current balance
- In the main test directory(where the Main class lives), compile the Main.java file. This has a cascading effect of compiling all of the classes used in the program; thus compiling the Customer.java and Account.java files under the banking directory.
javac -d . Main.java - Run the Main class. You shoud see the following output:
Creating an account with a 500.00 balance. Withdraw 150.00 Deposit 22.50 Withdraw 47.62 The account has a balance of 324.88
- 前置代码
/* PRESET CODE BEGIN - NEVER TOUCH CODE BELOW */ /* * This class creates the program to test the banking classes. * It creates a new Bank, sets the Customer (with an initial balance), * and performs a series of transactions with the Account object. */ // Note that this import statement is commented // import banking.*; public class Main{ public static void main(String[] args) { Account account; // Create an account that can has a 500.00 balance. System.out.println("Creating an account with a 500.00 balance."); account = new Account(500.00); System.out.println("Withdraw 150.00"); account.withdraw(150.00); System.out.println("Deposit 22.50"); account.deposit(22.50); System.out.println("Withdraw 47.62"); account.withdraw(47.62); // Print out the final account balance System.out.println("The account has a balance of " + account.getBalance()); } } /* PRESET CODE END - NEVER TOUCH CODE ABOVE */
需提交代码
class Account{ public double balance; public Account(double inti_balance){ balance = inti_balance; } public double getBalance() { return balance; } public double deposit(double amount) { balance += amount; return amount; } public double withdraw(double amount) { balance -= amount; return amount; } }
8.Solve quadratic equations
The two roots of a quadratic equation ax^2 + bx + c = 0 can be obtained using the following formula:
r1 = (-b + sqrt(b^2 - 4ac))/2a
and
r2 = (-b - sqrt(b^2 - 4ac))/2a
b^2 - 4ac is called the discriminant of the quadratic equation. If it is positive, the equation has two real roots. If it is zero, the equation has one root. If it is negative, the equation has no real roots.
Write a program that gets the user input values for a, b, and c and displays the result based on the discriminant. If the discriminant is positive, display two roots. If the discriminant is 0 , display one root. Otherwise, display “The equation has no real roots”.
Input:
Three numbers of a, b and c separated by the blank character. Example:
1.0 3 1
Output: the result or r1 and r2, please keep 6 digits after the decimal point.
-0.381966 -2.618034
Hints:
You can use Math.pow(x, 0.5) to compute sqrt(x).
解二次方程,引入Java.lang.Math,然后调用Math.sqrt开根号
import java.lang.Math;
import java.util.*;
public class Main{
public static void main(String[] args){
Scanner s = new Scanner(System.in);
double a = s.nextDouble();
double b = s.nextDouble();
double c = s.nextDouble();
double d = b*b - 4*a*c;
if (d == 0){
double r = (-b+Math.sqrt(d))/(2*a);
System.out.printf("%f\n",r);
}
if (d > 0 ){
double r1 = (-b+Math.sqrt(d))/(2*a);
double r2 = (-b-Math.sqrt(d))/(2*a);
System.out.printf("%f %f\n", r1, r2);
}
else System.out.println("The equation has no real roots");
s.close();
}
}