作者:明明如月学长, CSDN 博客专家,蚂蚁集团高级 Java 工程师,《性能优化方法论》作者、《解锁大厂思维:剖析《阿里巴巴Java开发手册》》、《再学经典:《EffectiveJava》独家解析》专栏作者。
热门文章推荐:
一、背景
今天有个朋友在技术群里请教,“Java有啥比较好用的语言检测的工具吗,只要检测出非英文就行,目前试了language detector或者字符检测效果都不理想”
可以看到该同学耗费了很多时间。技术群里展开了大讨论,有些朋友说用是否包含英语字母来判断,该同学说德语、意大利语容易误判。
那单纯靠字符误判率较高,还有什么更好地方法吗?
人工智能时代,遇事不决, GPT…
接下来重点展示如何通过 New Bing 帮助我们更快、更好地解决问题。
二、过程
2.1 准备提示词
我们可以尝试用中文提问,如果中文得不到很好的答案可以,将我们的需求描述使用 New Bing 或者其他翻译软件转为英文:
请用 Java语言编写了一个程序来判断一段文本是否为英语,除英语以外的任何语言都会返回 false。
If you write a program in the Java language that determines whether a piece of text is English, any language other than English returns false.
2.2 提问
2.2.1 如何实现
Bing 给了我们如下的答案:
There are different ways to write a program in Java that determines whether a piece of text is English. One possible way is to use a library called Apache Tika that can detect the language of a given text. Here is an example of how to use it:
// import the library
import org.apache.tika.language.LanguageIdentifier;
// create a string with some text
String text = "This is an example of English text";
// create a language identifier object
LanguageIdentifier identifier = new LanguageIdentifier(text);
// get the language code of the text
String language = identifier.getLanguage();
// check if the language code is "en" for English
if (language.equals("en")) {
System.out.println("The text is English");
} else {
System.out.println("The text is not English");
}
This program will print “The text is English” as the output. If you change the text to another language, such as 中文, it will print “The text is not English”.
根据我们的经验 apache 的库一般质量都相当高,如果这个回答是靠谱的应该可以解决我们的问题。
2.2.2 寻找依赖
代码有了,我们也知道了哪个库的哪个类,我们直接让 New Bing 帮我找到 maven 依赖。
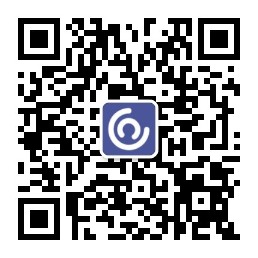
如果您想在Maven项目中使用 org.apache.tika.language.LanguageIdentifier
类,您需要添加 tika-langdetect 模块的依赖到您的 pom.xml 文件中。
这个模块提供了语言检测的功能,可以根据文本内容来判断语言。以下是 tika-langdetect 模块的Maven依赖:
<dependency>
<groupId>org.apache.tika</groupId>
<artifactId>tika-langdetect</artifactId>
<version>2.7.0</version>
</dependency>
New Bing 的“了解详情”部分给出了 maven 仓库的链接,我们可以点进去核实, New Bing 确实给了我们 maven 仓库的最新版:
但是经过验证,发现有两个问题:
(1) New Bing 给出的 maven 依赖,缺少 type 因为 2.7.0 版本为 pom 类型
(2)虽然版本最新,但是这个版本并不存在这个类
我们问 New Bing 这个版本里压根就没有 org.apache.tika.language.LanguageIdentifier
这个类,让它给我们其他版本:
no org.apache.tika.language.LanguageIdentifier in tika-langdetect 2.7.0, can you give me another versioin?
根据搜索结果,org.apache.tika.language.LanguageIdentifier
类已经在 Tika 2.0.0 版本中被移除,取而代之的是org.apache.tika.language.detect.LanguageDetector
接口和其实现类。如果您想继续使用 LanguageIdentifier
类,您需要使用Tika 1.x 版本的 tika-langdetect 模块,如以下的Maven依赖:
<dependency>
<groupId>org.apache.tika</groupId>
<artifactId>tika-langdetect</artifactId>
<version>1.28</version>
</dependency>
这是目前最新的Tika 1.x版本的 tika-langdetect 模块。如果您想使用 Tika 2.x版本的 tika-langdetect模块,您需要修改您的代码,使用 LanguageDetector
接口和其实现类,如 OptimaizeLangDetector
或 CommonsLang3LangDetector
。
2.2.3 寻找优化方法
既然最初的代码示例中的类已经废弃,我们提问如何使用 OptimaizeLangDetector
实现一样的功能
How do you use OptimaizeLangDetector to implement the same functionality?
根据搜索结果,OptimaizeLangDetector
是Tika 2.x版本中提供的一个语言检测的实现类,它使用了一个开源的语言检测库。要使用 OptimaizeLangDetector
来实现和 LanguageIdentifier
类相同的功能,您可以参考以下的示例代码:
// import the library
import org.apache.tika.language.detect.LanguageDetector;
import org.apache.tika.language.detect.LanguageResult;
import org.apache.tika.langdetect.OptimaizeLangDetector;
// create a string with some text
String text = "This is an example of English text";
// create a language detector object
LanguageDetector detector = new OptimaizeLangDetector().loadModels();
// add the text to the detector
detector.addText(text);
// get the language result of the text
LanguageResult result = detector.detect();
// get the language code of the text
String language = result.getLanguage();
// check if the language code is "en" for English
if (language.equals("en")) {
System.out.println("The text is English");
} else {
System.out.println("The text is not English");
}
这个程序也会打印 “The text is English” 作为输出。如果您改变文本为其他语言,如中文,它会打印"The text is not English"。
但是我们发现给的代码还不够完整,我希望它直接给我放到 isEnglish 方法里,让它继续优化:
i want you put this codes in a function like public static boolean isEnglish(String text)
如果您想把这些代码放在一个函数中,如 public static boolean isEnglish(String text)
,您可以参考以下的示例代码:
// import the library
import org.apache.tika.language.detect.LanguageDetector;
import org.apache.tika.language.detect.LanguageResult;
import org.apache.tika.langdetect.OptimaizeLangDetector;
// define a function that takes a string as input and returns a boolean value
public static boolean isEnglish(String text) {
// create a language detector object
LanguageDetector detector = new OptimaizeLangDetector().loadModels();
// add the text to the detector
detector.addText(text);
// get the language result of the text
LanguageResult result = detector.detect();
// get the language code of the text
String language = result.getLanguage();
// check if the language code is "en" for English and return true or false accordingly
return language.equals("en");
}
// test the function with some examples
System.out.println(isEnglish("This is an example of English text")); // true
System.out.println(isEnglish("这是一段中文文本的例子")); // false
System.out.println(isEnglish("Ceci est un exemple de texte français")); // false
发现 New Bing 已经帮我们写好了,甚至把一些用例都写好了,点赞!!
如果想让它再加一些其他语言在测试代码中或者有其他要求,也可以继续告诉它,让它修改。
我们将代码放在 IDE 里面运行,发现也符合预期。
当然,如果上述方案还不满意,你也可以尝试问 New Bing 有没有可以实现类似功能的其他类库等。
三、启发
人工智能时代,利用好 AI 工具,编写代码、解决问题的效率将得到倍速提升。
现在人工智能飞速发展,能力也在不断提升,但是回答的准确度还依赖于我们是否可以更准确和具体的表达意图。
我们对 New Bing 和 ChatGPT 提问时,当我们得不到想要的答案时,可以考虑换一种说法。如果你认为描述很准确还是不能得到理想的答案,可以尝试将提问翻译成英文再提问,可能效果更好。
此外,人工智能给的答案可以当做一个重要的参考,这些答案也可能会存在一些错误,需要我们自己去核实。
总之,人工智能时代,我们学习和解决问题的方式需要改变,遇事不决可以优先请教 AI 。
创作不易,如果本文对你有帮助,欢迎点赞、收藏加关注,你的支持和鼓励,是我创作的最大动力。