一、控件的简单介绍
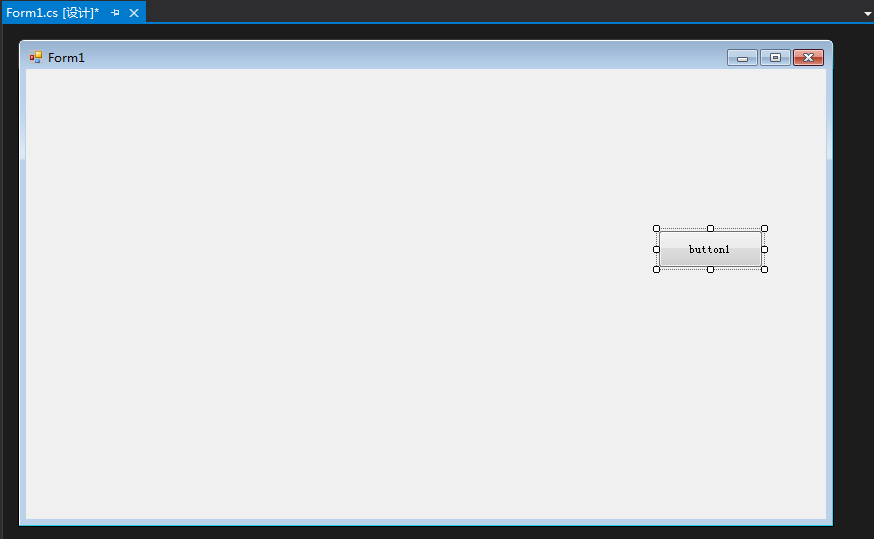
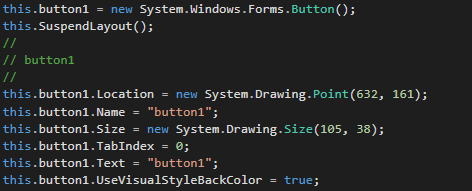
这里我可以按F11查看一下窗体代码执行的顺序:
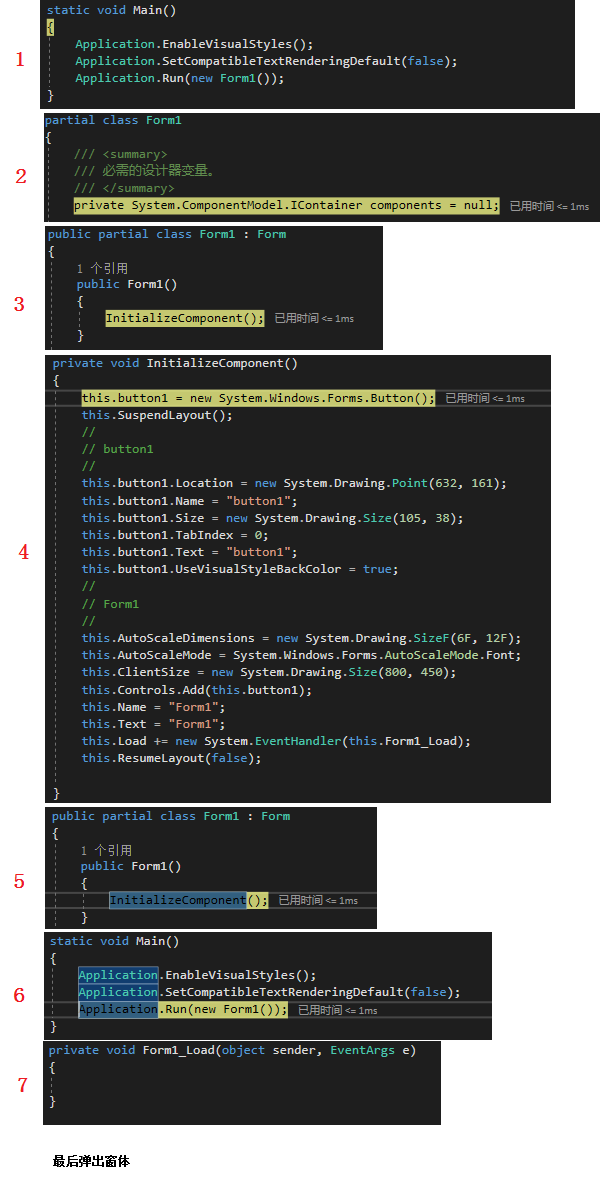
选中控件按F4,可以快速打开此控件都属性(注意:不要和其他控件或者主窗体都属性混淆了)
属性—外观—Text 中可以改变与控件中的显示文字。
属性—设计—(Name) 这里的是代码中用来标识该对象的名称。
属性—布局—Anchor 定义某个空间绑定到容器都边缘。当控件锚定到某个方向时,则该控件与此方向都边缘距离将保持不变。
属性—外观—BackColor 控件都背景颜色。
属性—外观—BackgroundImage 该控件都背景图像。
属性—外观—BackgroundImageLayout 该控件都背景图像在控件中的布局方式。None、Tile、Center、Stretch、Zoom
属性—行为—ContextMenuStrip 当用户在该控件范围内时点击右键显示快捷菜单。步骤如下:
将ContextMenuStrip拖入窗体中, 同时会在Form1.cs中生成以下代码: 在属性中选中此菜单与控件完成绑定 |
属性—外观—Cursor 鼠标指针移到该控件上方时显示都指针图形。
属性—行为—Visible 确定该控件是可见的还是隐藏的。
属性—行为—Enabled 指示是否已启动该控件。True是表示已启用,Flase表示未启用。
属性—外观—FlatStyle 鼠标移动到该控件并单击时,该控件都外观。备注:Popup有点3d的感觉。
属性—外观—FrontColor 控件的前景色,用于显示文本。
备注:,这里可以选择按首字母顺序排列,方便查找。
2、 TextBox
WordWrap:是否自动换行
ScrollBars:是否添加滚动条,Both标识上下左右都可以有滚动条。
PasswordChar:输入任何内容,都会变成你这里定义的字符。
3、label
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace _20220724_3
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void textBox1_TextChanged(object sender, EventArgs e)
{
label2.Text = textBox1.Text;
}
}
}
二、事件
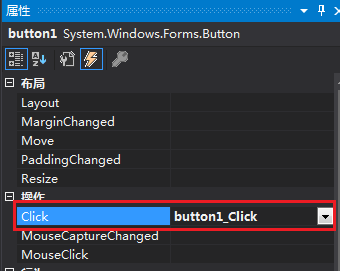
修改此处代码为:
private void button1_Click(object sender, EventArgs e)
{
MessageBox.Show("Hello World");
}
三、案例1
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
namespace _20220724
{
static class Program
{
/// <summary>
/// 应用程序的主入口点。
/// </summary>
[STAThread]
static void Main()
{
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new Form1());
}
}
}
//Form1.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace _20220724
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
//当加载窗体时,将窗体对象放到Test类中的静态字段中。
{
Test._fr1Test = this;
}
private void button1_Click(object sender, EventArgs e)
{
Form2 frm2 = new Form2();//在内存中创建,不会显示出来
frm2.Show();
}
private void contextMenuStrip1_Opening(object sender, CancelEventArgs e)
{
}
private void Form1_Click(object sender, EventArgs e)
{
}
}
}
//Form2.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace _20220724
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
private void Form2_Load(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
Form3 frm3 = new Form3();//在内存中创建,不会显示出来
frm3.Show();
}
}
}
//Form3.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace _20220724
{
public partial class Form3 : Form
{
public Form3()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
Test._fr1Test.Close();
}
}
}
//Test.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace _20220724
{
public static class Test
{
public static Form1 _fr1Test;
}
}
四、案例2
属性—事件—MouseEnter —在鼠标进入控件的可见部分时发生。
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace _20220724_2
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
MessageBox.Show("我也一样");
this.Close();//关闭主窗体
}
private void button2_MouseEnter(object sender, EventArgs e)
{
//当鼠标进入按钮的可见部分的时候,给按钮一个新的坐标。
int x = this.ClientSize.Width-button2.Width;//获取或设置窗体工作区的大小。
int y = this.ClientSize.Height - button2.Height;
Random r = new Random();
//给按钮一个随机的新坐标。
button2.Location = new Point(r.Next(0,x+1),r.Next(0, y + 1));
}
private void button2_Click(object sender, EventArgs e)
{
MessageBox.Show("还是被你点到了");
}
private void Form1_Load(object sender, EventArgs e)
{
}
}
}