末获取源码
开发语言:Java
开发工具:IDEA /Eclipse
数据库:MYSQL5.7
应用服务:Tomcat7/Tomcat8
JDK版本:jdk1.8
目录
前言介绍
对于本考研信息查询系统的设计来说,它主要是采用java技术。在整个系统的设计当中它是应用mysql数据库来完成的,具体根据网上考研资讯平台的现状来进行开发的,具体根据学生需求实现网上考研资讯平台网络化的管理,各类信息有序地进行存储,进入考研信息查询系统页面之后,方可开始操作主控界面,系统功能包括学生前台:首页、导师信息、学校介绍、专业信息、项目信息、论坛、个人中心、后台管理、登陆、在线客服,管理员:首页、个人中心、考研资讯管理、学校介绍管理、导师信息管理、考生信息管理、专业信息管理、设施申请管理、项目信息管理、论坛管理,系统管理。导师后台:前页,个人中心,设施申请管理。考生后台:首页、个人中心、设施申请管理
前台首页功能模块
首页
导师信息
学校介绍
专业信息
管理员功能模块
考研资讯管理
学校介绍管理
导师信息管理
考生信息管理
专业信息管理
设施申请管理
项目信息管理
论坛管理
系统管理
导师功能模块
个人中心
设施申请管理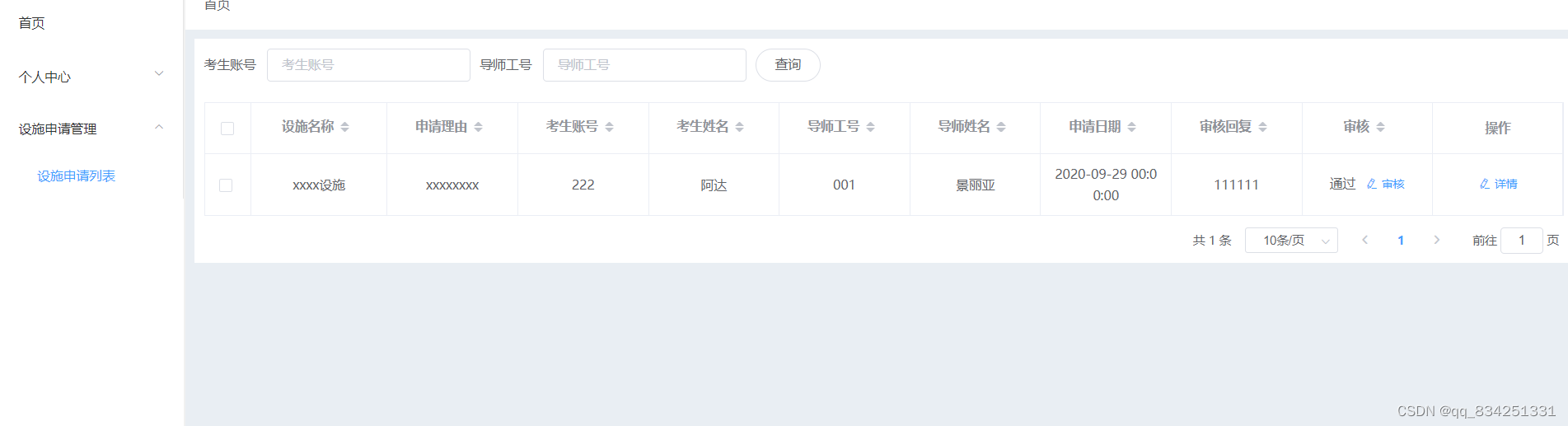
考生功能模块
个人中心
设施申请管理
部分核心代码:
controller层
/**
* 通用接口
*/
@RestController
public class CommonController{
@Autowired
private CommonService commonService;
@Autowired
private ConfigService configService;
private static AipFace client = null;
private static String BAIDU_DITU_AK = null;
@RequestMapping("/location")
public R location(String lng,String lat) {
if(BAIDU_DITU_AK==null) {
BAIDU_DITU_AK = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "baidu_ditu_ak")).getValue();
if(BAIDU_DITU_AK==null) {
return R.error("请在配置管理中正确配置baidu_ditu_ak");
}
}
Map<String, String> map = BaiduUtil.getCityByLonLat(BAIDU_DITU_AK, lng, lat);
return R.ok().put("data", map);
}
/**
* 人脸比对
*
* @param face1 人脸1
* @param face2 人脸2
* @return
*/
@RequestMapping("/matchFace")
public R matchFace(String face1, String face2,HttpServletRequest request) {
if(client==null) {
/*String AppID = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "AppID")).getValue();*/
String APIKey = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "APIKey")).getValue();
String SecretKey = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "SecretKey")).getValue();
String token = BaiduUtil.getAuth(APIKey, SecretKey);
if(token==null) {
return R.error("请在配置管理中正确配置APIKey和SecretKey");
}
client = new AipFace(null, APIKey, SecretKey);
client.setConnectionTimeoutInMillis(2000);
client.setSocketTimeoutInMillis(60000);
}
JSONObject res = null;
try {
File file1 = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+face1);
File file2 = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+face2);
String img1 = Base64Util.encode(FileUtil.FileToByte(file1));
String img2 = Base64Util.encode(FileUtil.FileToByte(file2));
MatchRequest req1 = new MatchRequest(img1, "BASE64");
MatchRequest req2 = new MatchRequest(img2, "BASE64");
ArrayList<MatchRequest> requests = new ArrayList<MatchRequest>();
requests.add(req1);
requests.add(req2);
res = client.match(requests);
System.out.println(res.get("result"));
} catch (FileNotFoundException e) {
e.printStackTrace();
return R.error("文件不存在");
} catch (IOException e) {
e.printStackTrace();
}
return R.ok().put("data", com.alibaba.fastjson.JSONObject.parse(res.get("result").toString()));
}
/**
* 获取table表中的column列表(联动接口)
* @param table
* @param column
* @return
*/
@IgnoreAuth
@RequestMapping("/option/{tableName}/{columnName}")
public R getOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,String level,String parent) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
if(StringUtils.isNotBlank(level)) {
params.put("level", level);
}
if(StringUtils.isNotBlank(parent)) {
params.put("parent", parent);
}
List<String> data = commonService.getOption(params);
return R.ok().put("data", data);
}
/**
* 根据table中的column获取单条记录
* @param table
* @param column
* @return
*/
@IgnoreAuth
@RequestMapping("/follow/{tableName}/{columnName}")
public R getFollowByOption(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName, @RequestParam String columnValue) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
params.put("columnValue", columnValue);
Map<String, Object> result = commonService.getFollowByOption(params);
return R.ok().put("data", result);
}
/**
* 修改table表的sfsh状态
* @param table
* @param map
* @return
*/
@RequestMapping("/sh/{tableName}")
public R sh(@PathVariable("tableName") String tableName, @RequestBody Map<String, Object> map) {
map.put("table", tableName);
commonService.sh(map);
return R.ok();
}
/**
* 获取需要提醒的记录数
* @param tableName
* @param columnName
* @param type 1:数字 2:日期
* @param map
* @return
*/
@IgnoreAuth
@RequestMapping("/remind/{tableName}/{columnName}/{type}")
public R remindCount(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("table", tableName);
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindStart);
remindStartDate = c.getTime();
map.put("remindstart", sdf.format(remindStartDate));
}
if(map.get("remindend")!=null) {
Integer remindEnd = Integer.parseInt(map.get("remindend").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindEnd);
remindEndDate = c.getTime();
map.put("remindend", sdf.format(remindEndDate));
}
}
int count = commonService.remindCount(map);
return R.ok().put("count", count);
}
/**
* 单列求和
*/
@IgnoreAuth
@RequestMapping("/cal/{tableName}/{columnName}")
public R cal(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
Map<String, Object> result = commonService.selectCal(params);
return R.ok().put("data", result);
}
/**
* 分组统计
*/
@IgnoreAuth
@RequestMapping("/group/{tableName}/{columnName}")
public R group(@PathVariable("tableName") String tableName, @PathVariable("columnName") String columnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("column", columnName);
List<Map<String, Object>> result = commonService.selectGroup(params);
return R.ok().put("data", result);
}
/**
* (按值统计)
*/
@IgnoreAuth
@RequestMapping("/value/{tableName}/{xColumnName}/{yColumnName}")
public R value(@PathVariable("tableName") String tableName, @PathVariable("yColumnName") String yColumnName, @PathVariable("xColumnName") String xColumnName) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("table", tableName);
params.put("xColumn", xColumnName);
params.put("yColumn", yColumnName);
List<Map<String, Object>> result = commonService.selectValue(params);
return R.ok().put("data", result);
}
}
/**
* 导师信息
* 后端接口
* @author
* @email
* @date 2020-09-29 15:12:22
*/
@RestController
@RequestMapping("/daoshixinxi")
public class DaoshixinxiController {
@Autowired
private DaoshixinxiService daoshixinxiService;
@Autowired
private TokenService tokenService;
/**
* 登录
*/
@IgnoreAuth
@RequestMapping(value = "/login")
public R login(String username, String password, String captcha, HttpServletRequest request) {
DaoshixinxiEntity user = daoshixinxiService.selectOne(new EntityWrapper<DaoshixinxiEntity>().eq("daoshigonghao", username));
if(user==null || !user.getMima().equals(password)) {
return R.error("账号或密码不正确");
}
String token = tokenService.generateToken(user.getId(), username,"daoshixinxi", "导师信息" );
return R.ok().put("token", token);
}
/**
* 注册
*/
@IgnoreAuth
@RequestMapping("/register")
public R register(@RequestBody DaoshixinxiEntity daoshixinxi){
//ValidatorUtils.validateEntity(daoshixinxi);
DaoshixinxiEntity user = daoshixinxiService.selectOne(new EntityWrapper<DaoshixinxiEntity>().eq("daoshigonghao", daoshixinxi.getDaoshigonghao()));
if(user!=null) {
return R.error("注册用户已存在");
}
Long uId = new Date().getTime();
daoshixinxi.setId(uId);
daoshixinxiService.insert(daoshixinxi);
return R.ok();
}
/**
* 退出
*/
@RequestMapping("/logout")
public R logout(HttpServletRequest request) {
request.getSession().invalidate();
return R.ok("退出成功");
}
/**
* 获取用户的session用户信息
*/
@RequestMapping("/session")
public R getCurrUser(HttpServletRequest request){
Long id = (Long)request.getSession().getAttribute("userId");
DaoshixinxiEntity user = daoshixinxiService.selectById(id);
return R.ok().put("data", user);
}
/**
* 密码重置
*/
@IgnoreAuth
@RequestMapping(value = "/resetPass")
public R resetPass(String username, HttpServletRequest request){
DaoshixinxiEntity user = daoshixinxiService.selectOne(new EntityWrapper<DaoshixinxiEntity>().eq("daoshigonghao", username));
if(user==null) {
return R.error("账号不存在");
}
user.setMima("123456");
daoshixinxiService.updateById(user);
return R.ok("密码已重置为:123456");
}
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,DaoshixinxiEntity daoshixinxi, HttpServletRequest request){
EntityWrapper<DaoshixinxiEntity> ew = new EntityWrapper<DaoshixinxiEntity>();
PageUtils page = daoshixinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, daoshixinxi), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,DaoshixinxiEntity daoshixinxi, HttpServletRequest request){
EntityWrapper<DaoshixinxiEntity> ew = new EntityWrapper<DaoshixinxiEntity>();
PageUtils page = daoshixinxiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, daoshixinxi), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( DaoshixinxiEntity daoshixinxi){
EntityWrapper<DaoshixinxiEntity> ew = new EntityWrapper<DaoshixinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( daoshixinxi, "daoshixinxi"));
return R.ok().put("data", daoshixinxiService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(DaoshixinxiEntity daoshixinxi){
EntityWrapper< DaoshixinxiEntity> ew = new EntityWrapper< DaoshixinxiEntity>();
ew.allEq(MPUtil.allEQMapPre( daoshixinxi, "daoshixinxi"));
DaoshixinxiView daoshixinxiView = daoshixinxiService.selectView(ew);
return R.ok("查询导师信息成功").put("data", daoshixinxiView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") String id){
DaoshixinxiEntity daoshixinxi = daoshixinxiService.selectById(id);
return R.ok().put("data", daoshixinxi);
}
/**
* 前端详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") String id){
DaoshixinxiEntity daoshixinxi = daoshixinxiService.selectById(id);
return R.ok().put("data", daoshixinxi);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody DaoshixinxiEntity daoshixinxi, HttpServletRequest request){
daoshixinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(daoshixinxi);
DaoshixinxiEntity user = daoshixinxiService.selectOne(new EntityWrapper<DaoshixinxiEntity>().eq("daoshigonghao", daoshixinxi.getDaoshigonghao()));
if(user!=null) {
return R.error("用户已存在");
}
daoshixinxi.setId(new Date().getTime());
daoshixinxiService.insert(daoshixinxi);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody DaoshixinxiEntity daoshixinxi, HttpServletRequest request){
daoshixinxi.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(daoshixinxi);
DaoshixinxiEntity user = daoshixinxiService.selectOne(new EntityWrapper<DaoshixinxiEntity>().eq("daoshigonghao", daoshixinxi.getDaoshigonghao()));
if(user!=null) {
return R.error("用户已存在");
}
daoshixinxi.setId(new Date().getTime());
daoshixinxiService.insert(daoshixinxi);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
public R update(@RequestBody DaoshixinxiEntity daoshixinxi, HttpServletRequest request){
//ValidatorUtils.validateEntity(daoshixinxi);
daoshixinxiService.updateById(daoshixinxi);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
daoshixinxiService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* 提醒接口
*/
@RequestMapping("/remind/{columnName}/{type}")
public R remindCount(@PathVariable("columnName") String columnName, HttpServletRequest request,
@PathVariable("type") String type,@RequestParam Map<String, Object> map) {
map.put("column", columnName);
map.put("type", type);
if(type.equals("2")) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
Calendar c = Calendar.getInstance();
Date remindStartDate = null;
Date remindEndDate = null;
if(map.get("remindstart")!=null) {
Integer remindStart = Integer.parseInt(map.get("remindstart").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindStart);
remindStartDate = c.getTime();
map.put("remindstart", sdf.format(remindStartDate));
}
if(map.get("remindend")!=null) {
Integer remindEnd = Integer.parseInt(map.get("remindend").toString());
c.setTime(new Date());
c.add(Calendar.DAY_OF_MONTH,remindEnd);
remindEndDate = c.getTime();
map.put("remindend", sdf.format(remindEndDate));
}
}
Wrapper<DaoshixinxiEntity> wrapper = new EntityWrapper<DaoshixinxiEntity>();
if(map.get("remindstart")!=null) {
wrapper.ge(columnName, map.get("remindstart"));
}
if(map.get("remindend")!=null) {
wrapper.le(columnName, map.get("remindend"));
}
int count = daoshixinxiService.selectCount(wrapper);
return R.ok().put("count", count);
}
}
/**
* 上传文件映射表
*/
@RestController
@RequestMapping("file")
@SuppressWarnings({"unchecked","rawtypes"})
public class FileController{
@Autowired
private ConfigService configService;
/**
* 上传文件
*/
@RequestMapping("/upload")
public R upload(@RequestParam("file") MultipartFile file, String type,HttpServletRequest request) throws Exception {
if (file.isEmpty()) {
throw new EIException("上传文件不能为空");
}
String fileExt = file.getOriginalFilename().substring(file.getOriginalFilename().lastIndexOf(".")+1);
String fileName = new Date().getTime()+"."+fileExt;
File dest = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+fileName);
file.transferTo(dest);
if(StringUtils.isNotBlank(type) && type.equals("1")) {
ConfigEntity configEntity = configService.selectOne(new EntityWrapper<ConfigEntity>().eq("name", "faceFile"));
if(configEntity==null) {
configEntity = new ConfigEntity();
configEntity.setName("faceFile");
configEntity.setValue(fileName);
} else {
configEntity.setValue(fileName);
}
configService.insertOrUpdate(configEntity);
}
return R.ok().put("file", fileName);
}
/**
* 下载文件
*/
@IgnoreAuth
@RequestMapping("/download")
public void download(@RequestParam String fileName, HttpServletRequest request, HttpServletResponse response) {
try {
File file = new File(request.getSession().getServletContext().getRealPath("/upload")+"/"+fileName);
if (file.exists()) {
response.reset();
response.setHeader("Content-Disposition", "attachment; filename=\"" + fileName+"\"");
response.setHeader("Cache-Control", "no-cache");
response.setHeader("Access-Control-Allow-Credentials", "true");
response.setContentType("application/octet-stream; charset=UTF-8");
IOUtils.write(FileUtils.readFileToByteArray(file), response.getOutputStream());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}