源码获取:俺的博客首页 "资源" 里下载!
项目介绍
项目主要包括管理员与用户两种角色;
管理员角色包含以下功能:管理员登录,主页面,分类查看,修改个人信息,上传小说,查看小说,删除小说,评论顶收藏小说等功能。
用户角色包含以下功能:用户注册,用户登录,查看销售,查看小说,发布小说,搜索小说,查看个人信息等功能。
环境需要
1.运行环境:最好是java jdk 1.8,我们在这个平台上运行的。其他版本理论上也可以。
2.IDE环境:IDEA,Eclipse,Myeclipse都可以。推荐IDEA;
3.tomcat环境:Tomcat 7.x,8.x,9.x版本均可
4.硬件环境:windows 7/8/10 1G内存以上;或者 Mac OS;
5.数据库:MySql 5.7版本;
6.是否Maven项目:否
技术栈
1. 后端:servlet
2. 前端:JSP+css+javascript+bootstrap+jQuery
使用说明
1. 使用Navicat或者其它工具,在mysql中创建对应名称的数据库,并导入项目的sql文件;
2. 使用IDEA/Eclipse/MyEclipse导入项目,Eclipse/MyEclipse导入时,若为maven项目请选择maven;
若为maven项目,导入成功后请执行maven clean;maven install命令,然后运行;
3. 将项目中util/DBUtil.java配置文件中的数据库配置改为自己的配置;
4. 运行项目,输入localhost:8080/jsp_xiaoshuo_site
登录管理控制层:
@SuppressWarnings("serial")
public class LoginServlet extends HttpServlet{
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
resp.setContentType("text/plain;charset=utf-8");
req.setCharacterEncoding("utf-8");
PrintWriter out=resp.getWriter();
String username=req.getParameter("username");
String password=req.getParameter("password");
HttpSession session=req.getSession();
if(username==null||password==null){
return;
}
UserDao ud=new UserDao();
User user=ud.getUser(username);
if(user!=null){
if(user.getPassword().equals(password)){
out.print("{\"state\":0}");
session.setAttribute("user", user);
out.close();
}else{
out.print("{\"state\":2}");
out.close();
}
}else{
out.print("{\"state\":1}");
out.close();
}
}
}
用户信息管理控制层:
@SuppressWarnings("serial")
public class GetUserDetailServlet extends HttpServlet{
@SuppressWarnings("unused")
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setCharacterEncoding("utf-8");
resp.setContentType("text/plain;charset=utf-8");
String type=req.getParameter("type");
String uid=req.getParameter("uid");
int user_id=0;
if(uid!=null){
user_id=Integer.parseInt(uid);
}
PrintWriter out=resp.getWriter();
if(type==null)return;
HttpSession session=req.getSession();
User user=(User)session.getAttribute("user");
if(user==null){
return;
}
boolean isSmall=false;
if(user_id==user.getUser_id())
isSmall=true;
if(type.equals("getuserpost")){
UserDao ud=new UserDao();
User u=ud.getUser(user_id);//当前浏览的用户
if(u==null)return;
PostDao pd=new PostDao();
List<Post> ps=pd.getPosts(u.getUser_id());//用户发表过的帖子
JSONArray jsonArray=new JSONArray();
/*time
* pid
* title
* isSamll*/
for(Post p:ps){
JSONObject json=new JSONObject();
json.put("time", p.getPost_time());
json.put("pid", p.getPost_id());
json.put("title", p.getTitle());
if(isSmall){
json.put("isSmall", "true");
}else{
json.put("isSmall", "false");
}
jsonArray.put(json);
}
out.print(jsonArray.toString());
}else if(type.equals("delpost")){
String pid=req.getParameter("pid");
if(pid==null)return;
int post_id=Integer.parseInt(pid);
PostDao pd=new PostDao();
Post p=pd.getPost(post_id);
if(p==null)return;
pd.delPost(post_id);
CollectionDao cld=new CollectionDao();
cld.delCollectionByPostId(post_id);
CommentDao cd=new CommentDao();
out.print("ok");
}else if(type.equals("delcollection")){
String clid=req.getParameter("clid");
if(clid==null)return;
int cl_id=Integer.parseInt(clid);
CollectionDao cld=new CollectionDao();
cld.delCollectionById(cl_id);
out.print("ok");
return;
}else if(type.equals("getusercollection")){
if(uid==null){return;}
CollectionDao cld=new CollectionDao();
List<Collection> cls=cld.getCollectionByUserId(user_id);
if(cls.size()==0)return;
PostDao pd=new PostDao();
UserDao ud=new UserDao();
JSONArray jsonArray=new JSONArray();
for(Collection c:cls){
Post p=pd.getPost(c.getPost_id());
int uuid=pd.getUserIdByPostId(p.getPost_id());
User u=ud.getUser(uuid);
JSONObject json=new JSONObject();
/*time
* cl_id
* pid
* title
* isSamll
* uid
* unickname
* pid time tile isSmall
*/
json.put("cl_id",c.getCollection_id());
json.put("pid", p.getPost_id());
json.put("title", p.getTitle());
json.put("time", c.getTime());
json.put("uid",u.getUser_id());
json.put("unickname", u.getNickname());
if(isSmall){
json.put("isSmall", "true");
}else{
json.put("isSmall", "false");
}
jsonArray.put(json);
}
out.print(jsonArray.toString());
}else if(type.equals("getusercomment")){
if(uid==null){return;}
CommentDao cd=new CommentDao();
List<Comment> cs=cd.getCommentByUserId(user_id);
JSONArray jsonArray=new JSONArray();
for(Comment c:cs){
PostDao pd=new PostDao();
String isExist="false";
Post p=pd.getPost(c.getPost_id()); //发表的帖子
JSONObject json=new JSONObject();
if(p!=null){
isExist="true";
UserDao ud=new UserDao();
User u=ud.getUser(p.getUser_id()); //创建人
json.put("uid", u.getUser_id());
json.put("title",p.getTitle());
json.put("unickname",u.getNickname());
json.put("pid",p.getPost_id());
}
json.put("cid", c.getComment_id());
json.put("time",c.getTime());
if(isSmall){
json.put("isSmall", "true");
}else{
json.put("isSmall", "false");
}
json.put("content", c.getContent());
json.put("isExist", isExist);
jsonArray.put(json);
}
out.print(jsonArray.toString());
/*cid time isSmall content uid title unickname pid isExist*/
}else if(type.equals("delcomment")){
String cid=req.getParameter("cid");
if(cid==null)return;
int comment_id=Integer.parseInt(cid);
CommentDao cd=new CommentDao();
cd.delCommentById(comment_id);
out.print("ok");
}
}
}
评论管理控制层:
//评论相关
@SuppressWarnings("serial")
public class CommentServlet extends HttpServlet{
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
req.setCharacterEncoding("utf-8");
resp.setContentType("text/html;charset=utf-8");
String type=req.getParameter("type");
String content=req.getParameter("content");
String pid=req.getParameter("pid");
PrintWriter out=resp.getWriter();
if(type==null||content==null||pid==null){
return;
}
HttpSession session=req.getSession();
User user=(User)session.getAttribute("user");
if(user==null){
out.print("<script type='text/javascript'>"
+ "window.location.href='/jsp_xiaoshuo_site/index.jsp'"
+ "</script>");
return;
}
int post_id=Integer.parseInt(pid);
Comment com=new Comment();
com.setContent(content);
com.setPost_id(post_id);
com.setUser_id(user.getUser_id());
Date now=new Date();
SimpleDateFormat sdf=new SimpleDateFormat("yyyy-MM-dd HH:mm");
String time=sdf.format(now);
com.setTime(time);
CommentDao cd=new CommentDao();
cd.insert(com);
out.print("<script type='text/javascript'>"
+ "window.location.href='/jsp_xiaoshuo_site/jsp/postdetail.jsp?pid="+pid+"'"
+ "</script>");
}
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
}
}
扫描二维码关注公众号,回复:
14322538 查看本文章
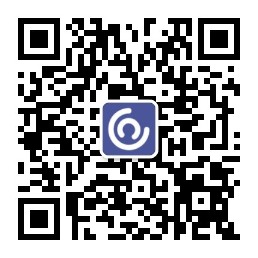
源码获取:俺的博客首页 "资源" 里下载!