1、使用递归计算阶乘
递归函数有一个最大的缺陷,就是增加了系统的开销。因为,每当调用一个函数时,系统需要为函数准备堆栈空间存储参数信息,如果频繁的进行递归调用,系统需要为其开辟大量的堆栈空间。
#include "stdafx.h"
#include "iostream.h"
int factorial(const int nNumber) //定义递归函数
{
if (nNumber == 0 || nNumber == 1) //递归结束套件
return 1;
else
{
return nNumber * factorial(nNumber-1); //直接调用本身
}
}
int main(int argc, char* argv[])
{
factorial(5);
return 0;
}
2、函数指针
在c++语言中,函数名实际上是指向函数的指针。
#include "stdafx.h"
#include "iostream.h"
void OutputLong(long nData) //定义一个long类型参数的函数
{
cout << nData << endl;
}
int OutputInt(int nData) //定义一个返回值为int类型的函数
{
cout << nData << endl;
return 0;
}
void OutputNumber(int nData) //定义一个无返回值的函数
{
cout << nData << endl;
}
void (*funOutput)(int); //定义一个函数指针
int main(int argc, char* argv[])
{
//funOutput = &OutputLong; //错误的语句,不能够进行赋值
//funOutput = &OutputInt; //错误的语句,不能够进行赋值
funOutput = &OutputNumber; //正确,可以进行赋值
(*funOutput)(10); //通过函数指针调用OutputNumber函数
return 0;
}
3、模板函数
其中,template是关键字用于定义模板(可以是函数模板,也可以是类模板),尖括号<>表示模板参数。模板参数主要有两种,一种是模板类型参数,另一种是模板非类型参数。
下面的代码定义了一个函数模板Max,其中模板类型参数type和模板非类型参数nLen。
#include "stdafx.h"
template <class type,int nLen> //定义一个模板类型
type Max(type Array[nLen]) //定义函数模板
{
type tRet = Array[0]; //定义一个变量
for(int i=1; i<nLen; i++) //遍历数组元素
{
tRet = (tRet > Array[i])? tRet : Array[i]; //比较数组元素大小
}
return tRet; //返回最大值
}
int main(int argc, char* argv[])
{
int nArray[5] = {1,2,3,4,5}; //定义一个整型数组
int nRet = Max<int,5>(nArray); //调用函数模板Max
double dbList[3] = {10.5,11.2,9.8}; //定义实数数组
double dbRet = Max<double,3>(dbList); //调用函数模板Max
return 0;
}
4、重载模板参数
#include "stdafx.h"
#include "iostream.h"
template <class type> //定义一个模板类型
type Add(type Plus,type Summand) //定义一个重载的函数模板
{
return Plus + Summand; //返回两个数之和
}
template <class type> //定义一个模板类型
type Add(type Array[], int nLen) //定义一个重载的函数模板
{
type tRet = 0; //定义一个变量
for(int i=0; i<nLen; i++) //利用循环累计求和
{
tRet += Array[i];
}
return tRet; //返回结果
}
int main(int argc, char* argv[]) //主函数
{
int nRet = Add(100, 200); //调用第一个重载的函数模板,实现两个数的求和运算
cout << "整数之和: " << nRet << endl; //输出结果
int nArray[5]= {1, 2, 3, 4, 5}; //定义一个整型数组
int nSum = Add(nArray, 5); //调用第2个重载的函数模板,实现数组元素的求和运算
cout << "数组元素之和:" << nSum << endl; //输出结果
return 0;
}
5、局部/全局作用域
局部作用域示例1
#include "stdafx.h"
#include "iostream.h"
int main(int argc, char* argv[])
{
{ //复合语句,有其自己的作用域
int nLen = 10; //定义一个局部变量,处于复合语句的作用域中
cout << nLen << endl; //nLen等于10
}
cout << nLen << endl; //访问错误
return 0;
}
局部作用域示例2
#include "stdafx.h"
#include "iostream.h"
int main(int argc, char* argv[])
{
int nLen = 5;
{ //复合语句,有其自己的作用域
int nLen = 10; //定义一个局部变量,处于复合语句的作用域中
cout << nLen << endl; //nLen等于10
}
cout << nLen << endl; //nLen等于5
return 0;
}
全局作用域示例1
#include "stdafx.h"
#include "iostream.h"
int VAR = 10; //定义全局变量
int main(int argc, char* argv[])
{
int VAR = 5; //定义局部变量
cout << "局部变量: " << VAR << endl; //输出局部变量
cout << "全局变量" << ::VAR << endl; //访问全局变量
return 0;
}
6、命名空间
开发时经常会出现多个文件中对象同名的情况,会导致程序的错误,为了解决冲突,c++引入了命名空间。
另外对于同一个命名空间,可以在多个文件中定义,此命名空间的内容就是多个文件的“和”。
还可以定义嵌套的命名空间。也可以不指定命名空间的名称。
#include "stdafx.h"
#include "iostream.h"
namespace Output //定义一个命名空间Output
{
const int MAXLEN = 128; //定义一个常量
int nLen = 10; //定义一个整型变量
void PutoutText(const char* pchData) //定义一个输出函数
{
if (pchData != NULL) //判断指针是否为空
{
cout << "PutoutText命名空间: " << pchData << endl; //输出数据
}
}
}
namespace Windows //定义一个命名空间Windows
{
typedef unsigned int UINT; //自定义一个类型
void PutoutText(const char* pchData) //定义一个输出函数
{
if (pchData != NULL) //判断指针是否为空
{
cout << "PutoutText命名空间: " << pchData << endl; //输出数据
}
}
}
int main(int argc, char* argv[])
{
int nLen = 5; //定义一个局部变量
using namespace Output; //使用Output命名空间
PutoutText("Welcome to CHINA!"); //访问命名空间中的PutoutText函数
cout << nLen << endl; //nLen的值为5
cout << Output::nLen << endl; //nLen的值为10
return 0;
}
7、遍历磁盘目录
#include "stdafx.h"
#include <io.h>
#include <string.h>
#include <iostream.h>
const int MAXLEN = 1024; //定义最大目录长度
unsigned long FILECOUNT = 0; //记录文件数量
void ListDir(const char* pchData)
{
_finddata_t fdata; //定义文件查找结构对象
long done;
char tempdir[MAXLEN]={0}; //定义一个临时字符数组,存储目录
strcat(tempdir, pchData); //连接字符串
strcat(tempdir, "\\*.*"); //连接字符串
done = _findfirst(tempdir, &fdata); //开始查找文件
if (done != -1) //是否查找成功
{
int ret = 0;
while (ret != -1) //定义一个循环
{
if (fdata.attrib != _A_SUBDIR) //判断文件属性
{
if (strcmp(fdata.name,"...") != 0 &&
strcmp(fdata.name,"..") != 0 &&
strcmp(fdata.name,".") != 0) //过滤.
{
char dir[MAXLEN]={0}; //定义字符数组
strcat(dir,pchData); //连接字符串
strcat(dir,"\\"); //连接字符串
strcat(dir,fdata.name); //连接字符串
cout << dir << endl; //输出查找的文件
FILECOUNT++; //累加文件
}
}
ret = _findnext(done, &fdata); //查找下一个文件
if (fdata.attrib == _A_SUBDIR && ret != -1) //判断文件属性,如果是目录,则递归调用
{
if (strcmp(fdata.name,"...") != 0 &&
strcmp(fdata.name,"..") != 0 &&
strcmp(fdata.name,".") != 0) //过滤.
{
char pdir[MAXLEN]= {0}; //定义字符数组
strcat(pdir,pchData); //连接字符串
strcat(pdir , "\\"); //连接字符串
strcat(pdir,fdata.name); //连接字符串
ListDir(pdir); //递归调用
}
}
}
}
}
int main(void)
{
while (true) //设计一个循环
{
FILECOUNT = 0;
char szFileDir[128] = {0}; //定义一个字符数组,存储目录
cin >> szFileDir;
if (strcmp(szFileDir, "e") == 0) //退出系统
{
break;
}
ListDir(szFileDir); //调用ListDir函数遍历目录
cout << "共计" << FILECOUNT << "个文件" << endl; //统计文件数量
}
return 0;
}
扫描二维码关注公众号,回复:
13802200 查看本文章
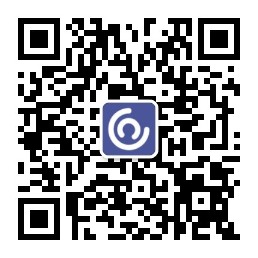