一起养成写作习惯!这是我参与「掘金日新计划 · 4 月更文挑战」的第3天,点击查看活动详情。
迈向全栈的第三步
Model 数据模型
从官网上对于 mongoose 的使用是基于模块化引入,我们使用 nestjs-typegoose
依赖来实现模块引入
npm i nestjs-typegoose --save
复制代码
修改 main.ts
文件,在入口文件中去掉数据库连接,改为在 app.module.ts
模块中引入的方式
async function bootstrap() {
// mongoose.connect('mongodb://localhost/nestjs-test-api', {}, (err: any) => {
// if (err) {
// console.log(err, '数据库连接错误!');
// }
// console.log('数据库连接成功!');
// });
...
}
bootstrap();
复制代码
// app.module.ts
import { TypegooseModule } from 'nestjs-typegoose';
@Module({
imports: [
TypegooseModule.forRoot('mongodb://localhost/nestjs-test-api', {}),
PostsModule,
],
controllers: [AppController],
providers: [AppService],
})
export class AppModule { }
复制代码
使用 TypegooseModule
链接数据库,再在 Post
模块下引入对应的 Post
数据模型后,可以在控制器内注入模型使用。
由上图简单的关系我们可以大致修改下对应的代码,就可以实现模块化注入模型。
先修改模型文件 post.model.ts
import { prop } from '@typegoose/typegoose';
export class Post {
@prop()
title: string
@prop()
content: string
}
// export const PostModel = getModelForClass(Post);
复制代码
可以直接导出数据模型,而不用使用原来的导出包裹方式。接着在 posts.module.ts
模块中引入对于的数据模型。
import { TypegooseModule } from 'nestjs-typegoose';
import { Post } from './post.model';
@Module({
imports: [
TypegooseModule.forFeature([Post]),
],
controllers: [PostsController],
})
复制代码
在控制器中注入模型 posts.controller.ts
import { ModelType } from '@typegoose/typegoose/lib/types';
import { InjectModel } from 'nestjs-typegoose';
import { Post as PostSchema } from './post.model';
export class PostsController {
constructor(
@InjectModel(PostSchema)
private readonly postModel: ModelType<PostSchema>
) { }
...
}
复制代码
利用 @InjectModel
装饰器后,可以在接口中通过 this.postModel
访问模型。
扫描二维码关注公众号,回复:
13785088 查看本文章
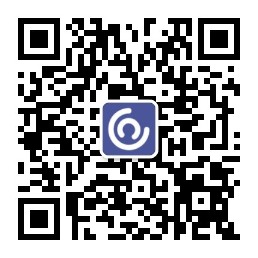
@Controller('posts')
@ApiTags('Posts')
export class PostsController {
constructor(
@InjectModel(PostSchema)
private readonly postModel: ModelType<PostSchema>
) { }
@Get()
@ApiOperation({
summary: '获取文章列表数据',
})
async index() {
return await this.postModel.find();
}
...
}
复制代码
这样解耦出模型,可以在任意模块注入使用。
CRUD 生成器
Nest CLI
自动生成模块所需要的代码文件。让我们的开发变得简单且高效
nest g resource
复制代码
cmd
运行效果
-
运行生成的模板文件
在项目根目录下执行命令后,可以生成所有的 Nest
构建文件,包括模块、服务、控制器,也生成了实体类,DTO
类和测试文件。只关心模型定义实现业务即可。
总结
到此,对于 `Nest` 我们熟悉了基本的使用规则,后续接着实现如何在项目内管理多个子项目。
复制代码