jfinal 由于其特性 快速 简单 易上手 被越来越多的程序员重视,beetl 其开发效率极高 维护简单 比jsp性能高2倍 比freemarker 高16倍(大师说的 不是我说的 勿喷)
行了 废话少说 给大家截个图
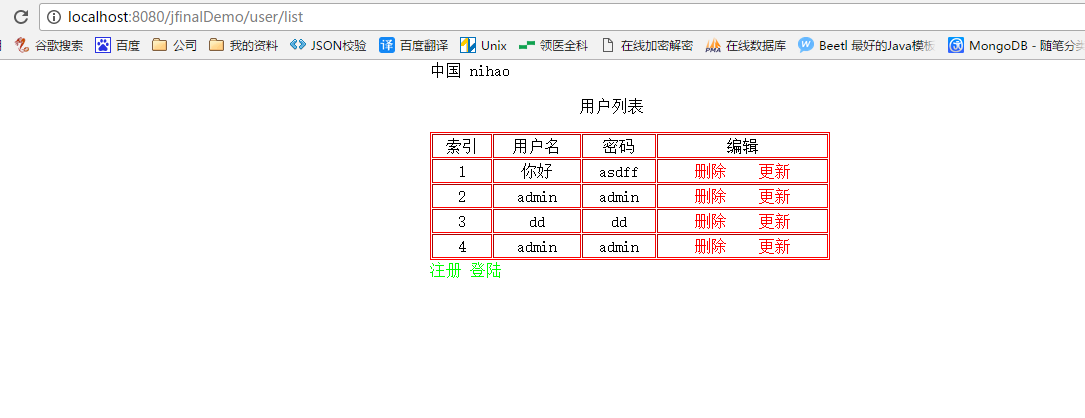
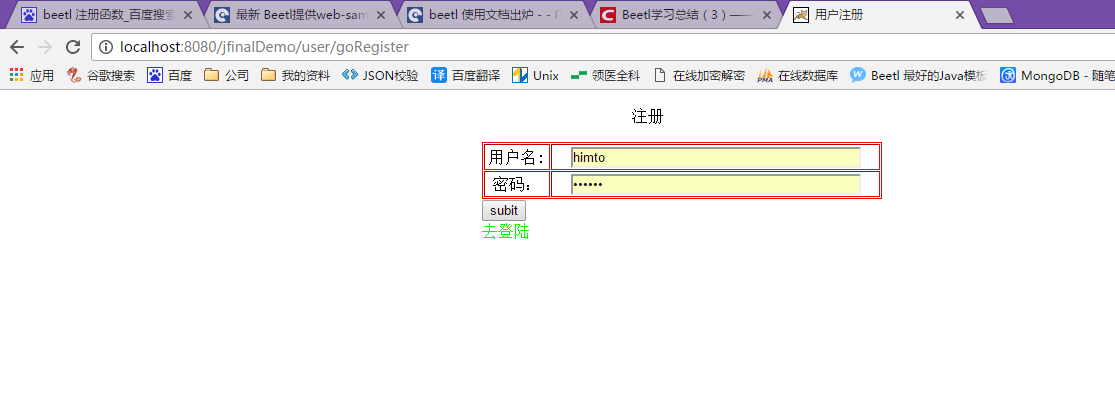
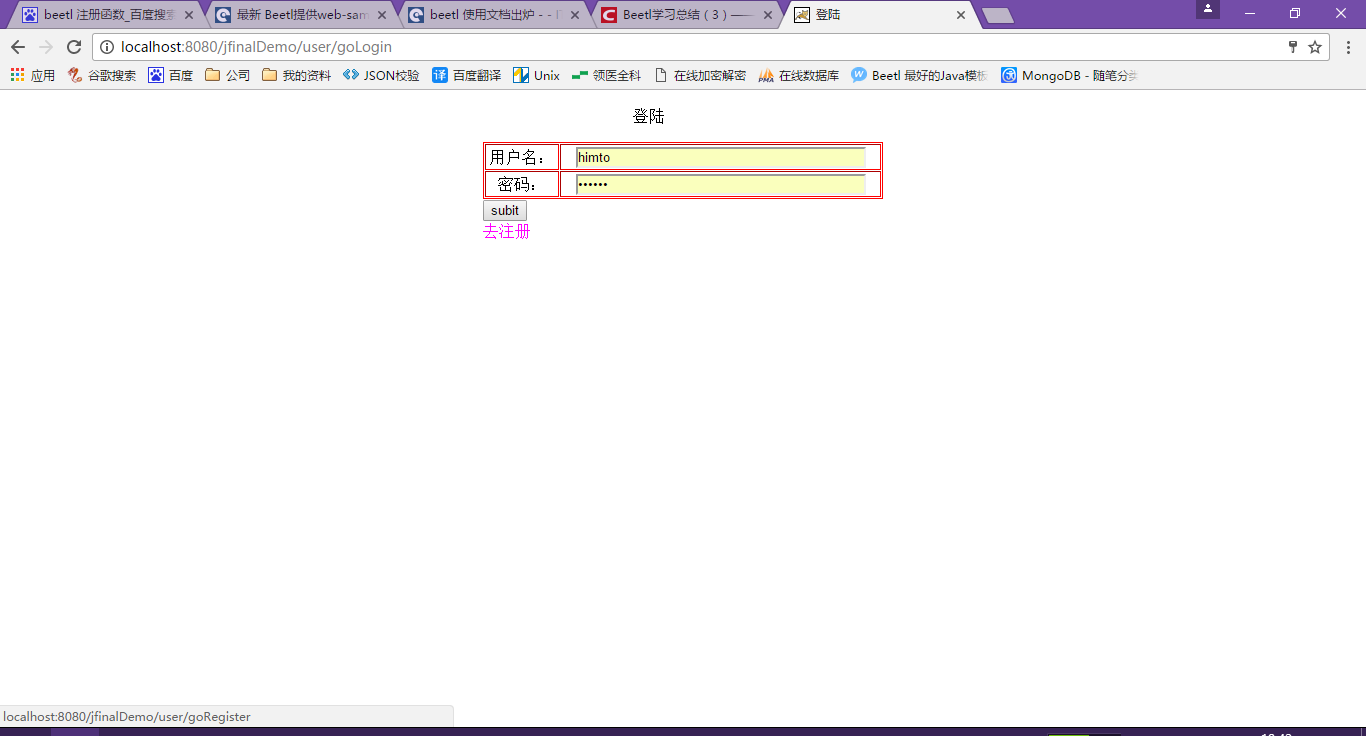
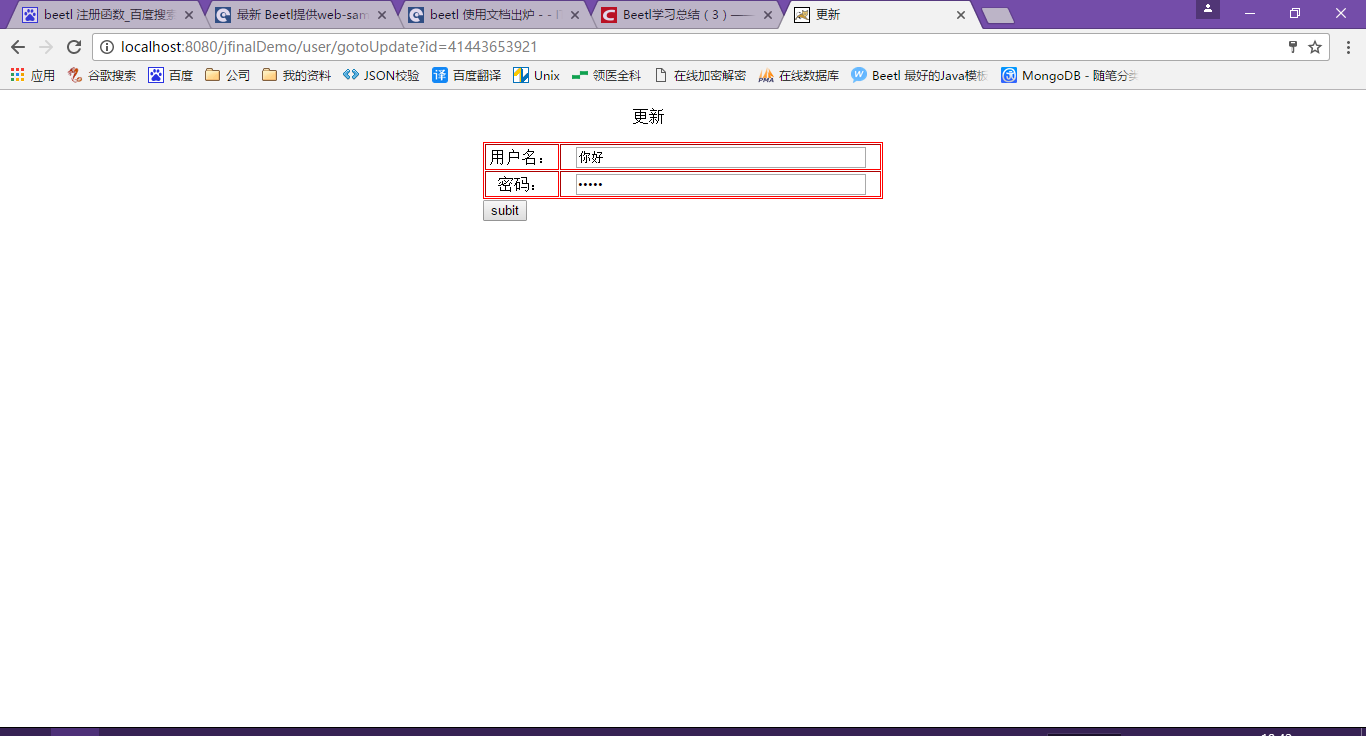
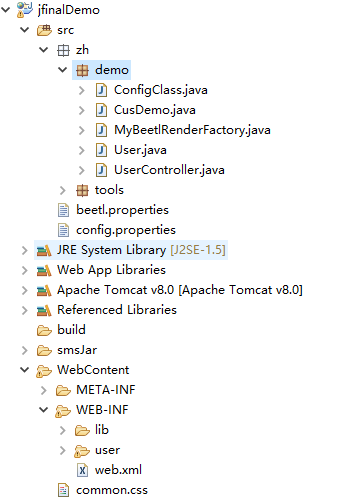
直接来代码吧
1.ConfigClass.class
package zh.demo;
import com.jfinal.config.Constants;
import com.jfinal.config.Handlers;
import com.jfinal.config.Interceptors;
import com.jfinal.config.JFinalConfig;
import com.jfinal.config.Plugins;
import com.jfinal.config.Routes;
import com.jfinal.kit.PropKit;
import com.jfinal.plugin.activerecord.ActiveRecordPlugin;
import com.jfinal.plugin.c3p0.C3p0Plugin;
import com.jfinal.render.ViewType;
public class ConfigClass extends JFinalConfig {
@Override
public void configConstant(Constants me) {
me.setDevMode(true);
PropKit.use("config.properties");
// 配置模板
me.setMainRenderFactory(new MyBeetlRenderFactory());
// 获取GroupTemplate模板,可以设置共享变量操作
//GroupTemplate groupTemplate = MyBeetlRenderFactory.groupTemplate;
//me.setDevMode(getPropertyToBoolean("config.devModel", false));
me.setViewType(ViewType.OTHER);
me.setEncoding("UTF-8");
}
@Override
public void configRoute(Routes me) {
//me.add("/hello", HelloController.class);
me.add("/user", UserController.class);
}
@Override
public void configPlugin(Plugins me) {
C3p0Plugin c3p0Plugin = new C3p0Plugin(PropKit.get("jdbcUrl"), PropKit.get("user"), PropKit.get("password"));
ActiveRecordPlugin arp = new ActiveRecordPlugin(c3p0Plugin);
arp.setShowSql(true);
arp.addMapping("t_user_tab", User.class);
me.add(c3p0Plugin);
me.add(arp);
}
@Override
public void configInterceptor(Interceptors me) {
}
@Override
public void configHandler(Handlers me) {
// me.add(new ContextPathHandler("ctx"));
}
}
2.CusDemo.java
package zh.demo;
import java.io.IOException;
import org.beetl.core.Context;
import org.beetl.core.Function;
public class CusDemo implements Function {
public Object call(Object[] paras, Context ctx) {
Object o = paras[0];
if (o != null) {
try {
ctx.byteWriter.writeString("中国 "+o.toString());
} catch (IOException e) {
throw new RuntimeException(e);
}
}
return "";
}
}
3.MyBeetlRenderFactory.java
package zh.demo;
import org.beetl.ext.jfinal.BeetlRender;
import org.beetl.ext.jfinal.BeetlRenderFactory;
import com.jfinal.render.Render;
public class MyBeetlRenderFactory extends BeetlRenderFactory {
@Override
public Render getRender(String view) {
BeetlRender render = new BeetlRender(groupTemplate, view);
return render;
}
/*@Override
public String getViewExtension() {
//默认是html 不需要重写
return ".html";
}*/
}
4.User.java
package zh.demo;
import com.jfinal.plugin.activerecord.Model;
public class User extends Model<User> {
/**
*
*/
private static final long serialVersionUID = 5680502164779131689L;
public static User me = new User();
}
5.UserController.java
package zh.demo;
import java.util.List;
import com.jfinal.core.Controller;
import zh.tools.GenerateIdKit;
public class UserController extends Controller {
/**
* 注册页面
*
* @author zhangheng
* @date 2016年11月25日下午3:51:40
*/
public void goRegister() {
render("register.html");
}
/**
* 注册
*
* @author zhangheng
* @date 2016年11月25日下午3:52:46
*/
public void register() {
long id = GenerateIdKit.getId();
String name = getPara("name");
String password = getPara("pwd");
User user = new User();
user.set("name", name);
user.set("pwd", password);
user.set("id", id);
user.save();
System.out.println("注册成功");
goLogin();
}
/**
* 登陆页面
*
* @author zhangheng
* @date 2016年11月25日下午3:52:23
*/
public void goLogin() {
render("login.html");
}
/**
* 登陆
*
* @author zhangheng
* @date 2016年11月25日下午3:52:35
*/
public void login() {
String name = getPara("name");
String password = getPara("pwd");
List<User> users = User.me
.find("SELECT * FROM t_user_tab u WHERE u.name = '" + name + "' AND u.pwd = '" + password + "'");
setAttr("users", users);
if (users.size() > 0) {
System.out.println("登录成功");
} else {
System.out.println("登录失败");
}
redirect("/user/list");
}
/**
* 用户管理页面
*
* @author zhangheng
* @date 2016年11月25日下午3:51:59
*/
public void list() {
List<User> users = User.me.find("select * FROM t_user_tab where 1=1");
setAttr("users", users);
render("userList.html");
}
/**
* 删除用户
*
* @author zhangheng
* @date 2016年11月25日下午3:53:06
*/
public void delete() {
User.me.deleteById(getParaToLong("id"));
redirect("/user/list");
}
/**
* 更新页面
*
* @author zhangheng
* @date 2016年11月25日下午3:53:24
*/
public void gotoUpdate() {
User u = User.me.findById(getParaToLong("id"));
setAttr("user", u);
render("update.html");
}
/**
* 更新用户
*
* @author zhangheng
* @date 2016年11月25日下午3:53:34
*/
public void update() {
User u = User.me.findById(getParaToLong("id"));
u.set("name", getPara("name"));
u.set("pwd", getPara("pwd"));
u.update();
// list();
// forwardAction("user/list");
redirect("/user/list");
}
}
6.beetl.properties
RESOURCE.root=/WEB-INF/
FN.helloP = zh.demo.CusDemo
7.config.properties
jdbcUrl=jdbc:mysql://localhost:3306/demo
user=root
password=root
最重要的 web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://java.sun.com/xml/ns/javaee"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd"
id="WebApp_ID" version="2.5">
<display-name>jfinalDemo</display-name>
<welcome-file-list>
<welcome-file>user/login.jsp</welcome-file>
</welcome-file-list>
<filter>
<filter-name>jfinal</filter-name>
<filter-class>com.jfinal.core.JFinalFilter</filter-class>
<init-param>
<param-name>configClass</param-name>
<param-value>zh.demo.ConfigClass</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>jfinal</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
视图
都是谢类似的html或者jsp 笔者这里就不全部列出来了 如果有需要的朋友 可以私下联系 我放给你们
下面我就贴一个userList.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=8">
<meta http-equiv="Expires" content="0">
<meta http-equiv="Pragma" content="no-cache">
<meta http-equiv="Cache-control" content="no-cache">
<meta http-equiv="Cache" content="no-cache">
<title>list</title>
<link href="${ctxPath}/common.css" rel="stylesheet" type="text/css" />
</head>
<body>
<!-- ${users.~size} -->
${helloP('nihao')}
<p class="center">用户列表<p>
<table class="table" border="1">
<tr>
<td>索引</td>
<td>用户名</td>
<td>密码</td>
<td>编辑</td>
</tr>
<%
for(test in users!){%>
<tr>
<td> ${testLP.index}</td>
<td>${test.name}</td>
<td>${test.pwd!''}</td>
<td><a href = "delete?id=${test.id}">删除</a> <a href = "gotoUpdate?id=${test.id}">更新</a></td>
</tr>
<%
}
%>
</table>
<a href ="goRegister">注册</a> <a href ="goLogin">登陆</a>
</body>
</html>
以上内容纯属个人手打 转发请标出来源 谢谢,如果有bug 欢迎大家提