用Android Studio创建工程的时候,src文件夹下会同时生成三个文件夹main、test、androidTest
test和androidTest是专门针对源码级别的白盒测试的。
test:文件夹用于写不依赖设备环境的单元测试,即可在PC上直接运行;
androidTest:文件夹用于写需要在设备上才能运行的测试。
分类
功能测试:和UI无关,测试IO操作、算法、流程等;
UI测试:测试UI交互逻辑,比如点击、登陆等。
Java单元测试框架:JUnit、Mockito等;
Android:AndroidJUnitRunner、Espresso等。
本文主要介绍JUnit的常用注解和断言及其基本使用,其他测试框架会在后续博文中介绍。
JUnit
依赖:
dependencies {
testCompile 'junit:junit:4.12'
}
常用注解和断言:
Junit3中每个测试方法必须以test打头,Junit4中增加了注解,对方法名没有要求。
例:
创建一个计算的工具类
/**
* Created by scy on 2018/4/26.
*/
public class Calculater {
public int sum(int a, int b){
return a+b;
}
public int substract(int a, int b){
return a-b;
}
public int divide(int a, int b){
return a/b;
}
public int multiply(int a, int b){
return a*b;
}
}
然后依照下图进行操作
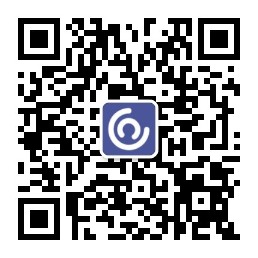
注:1选择要测试的方法 2点击确定 3选择测试类生成位置
自动创建测试类:
注意导包import static org.junit.Assert.*;
然后修改代码:
/**
* Created by scy on 2018/4/26.
*/
public class CalculaterTest {
private Calculater mCalculator;
private int a = 1;
private int b = 3;
@Before//在测试开始之前回调的方法
public void setUp() throws Exception {
mCalculator = new Calculater();
}
@Test//我们需要测试的方法
public void sum() throws Exception {
int result = mCalculator.sum(a, b);
// 第一个参数:"sum(a, b)" 打印的tag信息 (可省略)
// 第二个参数: 3 期望得到的结果
// 第三个参数 result:实际返回的结果
// 第四个参数 0 误差范围(可省略)
assertEquals("sum(a, b)",3,result,0);
}
@Test
public void substract() throws Exception {
int result = mCalculator.substract(a, b);
assertEquals(-3,result,2);
}
}
如果期望值和实际值在误差范围内,测试通过,否则测试不通过!
Android Studio对类右键->run CalculaterTest,用例中所有被@Test注解的方法,就会被执行。以上测试结果如下:
显示有一个错误sum()
方法期望得到3 实际返回4 误差为0,因此不通过;而第二个方法substract()
期望得到-3 实际返回-2 但是在误差范围2内,所以测试通过。
如果代码改成sum() ->Assert.assertEquals(4,result);
,测试会全部通过。
下面贴一个w3c的断言样例:
/**
* Created by scy on 2018/4/27.
*/
public class AssertTest {
@Test
public void testAssertions() {
//test data
String str1 = new String("abc");
String str2 = new String("abc");
String str3 = null;
String str4 = "abc";
String str5 = "abc";
int val1 = 5;
int val2 = 6;
String[] expectedArray = {"one", "two", "three"};
String[] resultArray = {"one", "two", "three"};
//Check that two objects are equal
assertEquals(str1, str2);
//Check that a condition is true
assertTrue(val1 < val2);
//Check that a condition is false
assertFalse(val1 > val2);
//Check that an object isn't null
assertNotNull(str1);
//Check that an object is null
assertNull(str3);
//Check if two object references point to the same object
assertSame(str4, str5);
//Check if two object references not point to the same object
assertNotSame(str1, str3);
//Check whether two arrays are equal to each other.
assertArrayEquals(expectedArray, resultArray);
}
}