1.定义统一的接口实体类
package com.iot.simmanager.base;
import java.io.Serializable;
/**
* 接口返回结果实体类
* @author lenny
* @date 20210429
*/
public class Result<T> implements Serializable {
private Integer code;
private boolean success;
private String message;
private T data;
public boolean isSuccess() {
return ResultEnum.SUCCESS.getCode() == this.code;
}
public Integer getCode() {
return this.code;
}
public String getMessage() {
return this.message;
}
public T getData() {
return this.data;
}
@Override
public boolean equals(final Object o) {
if (o == this) {
return true;
} else if (!(o instanceof Result)) {
return false;
} else {
Result<?> other = (Result)o;
if (!other.canEqual(this)) {
return false;
} else {
Object this$code = this.getCode();
Object other$code = other.getCode();
if (this$code == null) {
if (other$code != null) {
return false;
}
} else if (!this$code.equals(other$code)) {
return false;
}
if (this.isSuccess() != other.isSuccess()) {
return false;
} else {
Object this$message = this.getMessage();
Object other$message = other.getMessage();
if (this$message == null) {
if (other$message != null) {
return false;
}
} else if (!this$message.equals(other$message)) {
return false;
}
Object this$data = this.getData();
Object other$data = other.getData();
if (this$data == null) {
if (other$data != null) {
return false;
}
} else if (!this$data.equals(other$data)) {
return false;
}
return true;
}
}
}
}
protected boolean canEqual(final Object other) {
return other instanceof Result;
}
@Override
public int hashCode() {
boolean PRIME = true;
int result = 1;
Object $code = this.getCode();
result = result * 59 + ($code == null ? 43 : $code.hashCode());
result = result * 59 + (this.isSuccess() ? 79 : 97);
Object $message = this.getMessage();
result = result * 59 + ($message == null ? 43 : $message.hashCode());
Object $data = this.getData();
result = result * 59 + ($data == null ? 43 : $data.hashCode());
return result;
}
@Override
public String toString() {
return "XCoreResult(code=" + this.getCode() + ", success=" + this.isSuccess() + ", message=" + this.getMessage() + ", data=" + this.getData() + ")";
}
public Result(final Integer code, final boolean success, final String message, final T data) {
this.code = code;
this.success = success;
this.message = message;
this.data = data;
}
}
2.定义返回状态枚举
package com.iot.simmanager.base;
/**
* 结构返回状态枚举
* @author lenny
* @date 20210429
*/
public enum ResultEnum {
SUCCESS(200, "成功"),
USER_NOT_EXISTS(100, "用户不存在"),
DATA_NOT_EXISTS(100,"数据不存在"),
PASSWORD_ERROR(101, "登录密码错误"),
TOKEN_ERROR(102, "Token 验证失败"),
OPERATOR_NOT_ALLOW(103,"操作不允许"),
DATA_EXISTS(104,"数据已存在"),
PRARM_ERROR(105, "参数错误"),
FAIL(400, "失败"),
NOT_FOUND(404, "不存在"),
SERVER_ERROR(500, "服务异常");
private int code;
private String message;
private ResultEnum(int code, String message) {
this.code = code;
this.message = message;
}
public int getCode() {
return this.code;
}
public String getMessage() {
return this.message;
}
}
3.接口数据返回方法封装
package com.iot.simmanager.base;
/**
* 接口返回内容方法封装
* @author lenny
* @date 20210429
*/
public class ResultGenerator {
public ResultGenerator() {
}
/**
* 接口执行成功的返回
* @param <T>
* @return
*/
public static <T> Result<T> success() {
return new Result(ResultEnum.SUCCESS.getCode(), true, ResultEnum.SUCCESS.getMessage(), (Object)null);
}
/**
* 接口执行成功的返回
* @param data 需要返回的数据实体
* @param <T>
* @return
*/
public static <T> Result<T> success(final T data) {
return new Result(ResultEnum.SUCCESS.getCode(), true, ResultEnum.SUCCESS.getMessage(), data);
}
/**
* 接口执行成功的返回
* @param message 需要返回的消息
* @param data 需要返回的数据实体
* @param <T>
* @return
*/
public static <T> Result<T> success(final String message, final T data) {
return new Result(ResultEnum.SUCCESS.getCode(), true, message, data);
}
/**
* 接口执行失败的返回
* @param <T>
* @return
*/
public static <T> Result<T> error() {
return new Result(ResultEnum.SERVER_ERROR.getCode(), false, ResultEnum.SERVER_ERROR.getMessage(), (Object)null);
}
/**
* 接口执行失败的返回
* @param message 自定义的错误信息
* @param <T>
* @return
*/
public static <T> Result<T> error(final String message) {
return new Result(ResultEnum.SERVER_ERROR.getCode(), false, message, (Object)null);
}
/**
* 接口执行失败的返回
* @param code 自定义的错误编码
* @param message 自定义的错误信息
* @param <T>
* @return
*/
public static <T> Result<T> error(final int code, final String message) {
return new Result(code, false, message, (Object)null);
}
/**
* 接口执行失败的返回
* @param resultEnum 自定义返回错误编码的枚举
* @param <T>
* @return
*/
public static <T> Result<T> error(final ResultEnum resultEnum) {
return new Result(resultEnum.getCode(), false, resultEnum.getMessage(), (Object)null);
}
/**
* 接口执行失败的返回
* @param e 返回异常详情
* @param <T>
* @return
*/
public static <T> Result<T> error(final Exception e) {
return new Result(ResultEnum.SERVER_ERROR.getCode(), false, e.getMessage(), (Object)null);
}
}
4.Controller方法处理
package com.iot.simmanager.controller;
import com.iot.simmanager.base.Result;
import com.iot.simmanager.base.ResultEnum;
import com.iot.simmanager.base.ResultGenerator;
import com.iot.simmanager.model.AdminAgent;
import com.iot.simmanager.service.AdminAgentService;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiImplicitParam;
import io.swagger.annotations.ApiImplicitParams;
import io.swagger.annotations.ApiOperation;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
/**
* @author lenny
*/
@Api(tags = "公司管理")
@RestController
@RequestMapping("/agent")
public class AdminAgentController {
@Autowired
private AdminAgentService adminAgentService;
@ApiOperation(value = "查询公司列表", notes = "查询公司列表")
@PostMapping(value = "queryAdminAgentList")
public Result<Object> queryAdminAgentList(){
try {
List<AdminAgent> adminAgentList = adminAgentService.queryAdminAgentList();
return ResultGenerator.success(adminAgentList);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
@ApiOperation(value = "查询公司列表带参数", notes = "查询公司列表带参数")
@ApiImplicitParams({
@ApiImplicitParam(name = "key",value = "查询条件"),
@ApiImplicitParam(name = "pageNum",value = "当前页",required = true),
@ApiImplicitParam(name = "pageSize",value = "每页显示条数",required = true)
})
@PostMapping(value = "queryAdminAgentListParam")
public Result<Object> queryAdminAgentListParam(String key, int pageNum, int pageSize){
try {
if(pageNum<=0||pageSize<=0)
{
return ResultGenerator.error(ResultEnum.PRARM_ERROR);
}
List<AdminAgent> adminAgentList = adminAgentService.queryAdminAgentList();
return ResultGenerator.success("自定义消息描述",adminAgentList);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
5.测试实例
5.1.正常数据返回
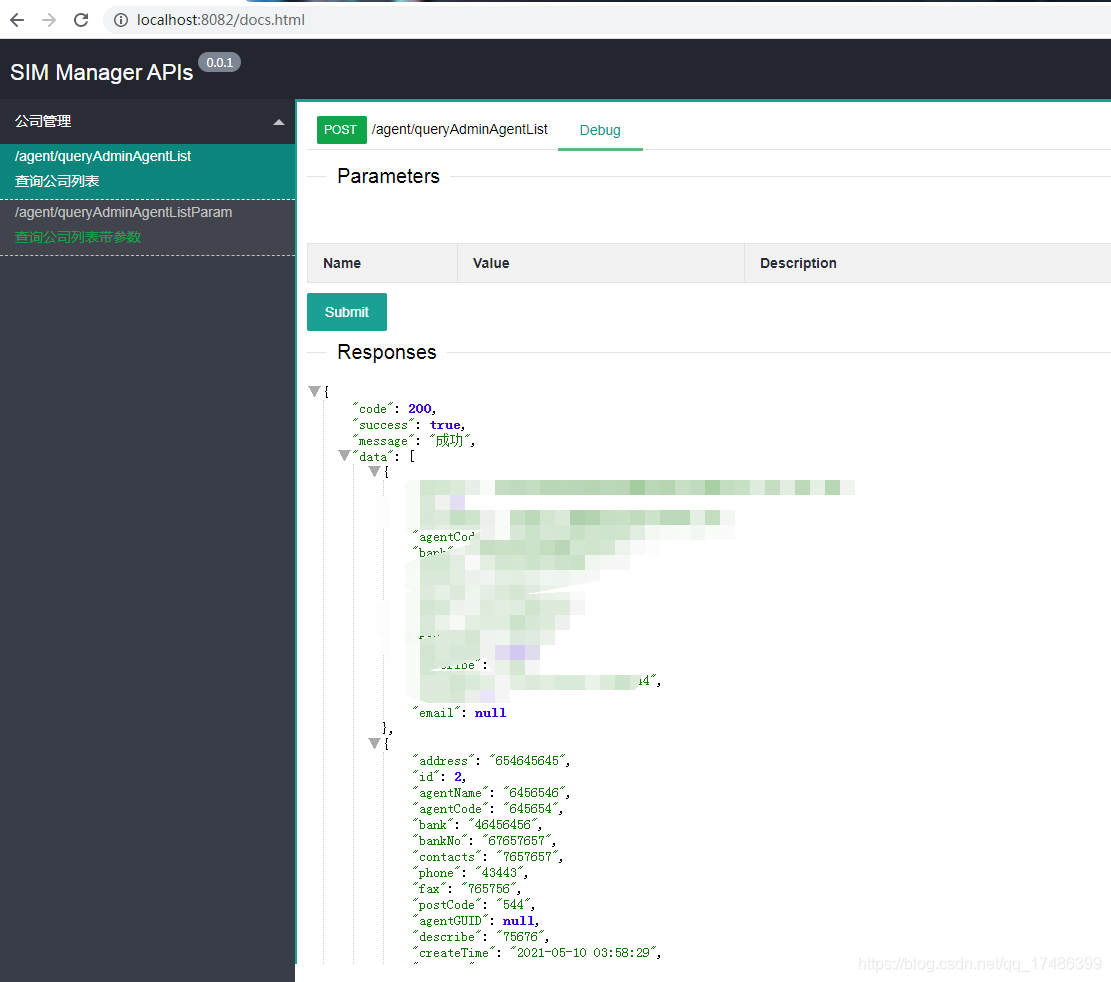
5.2.异常数据返回
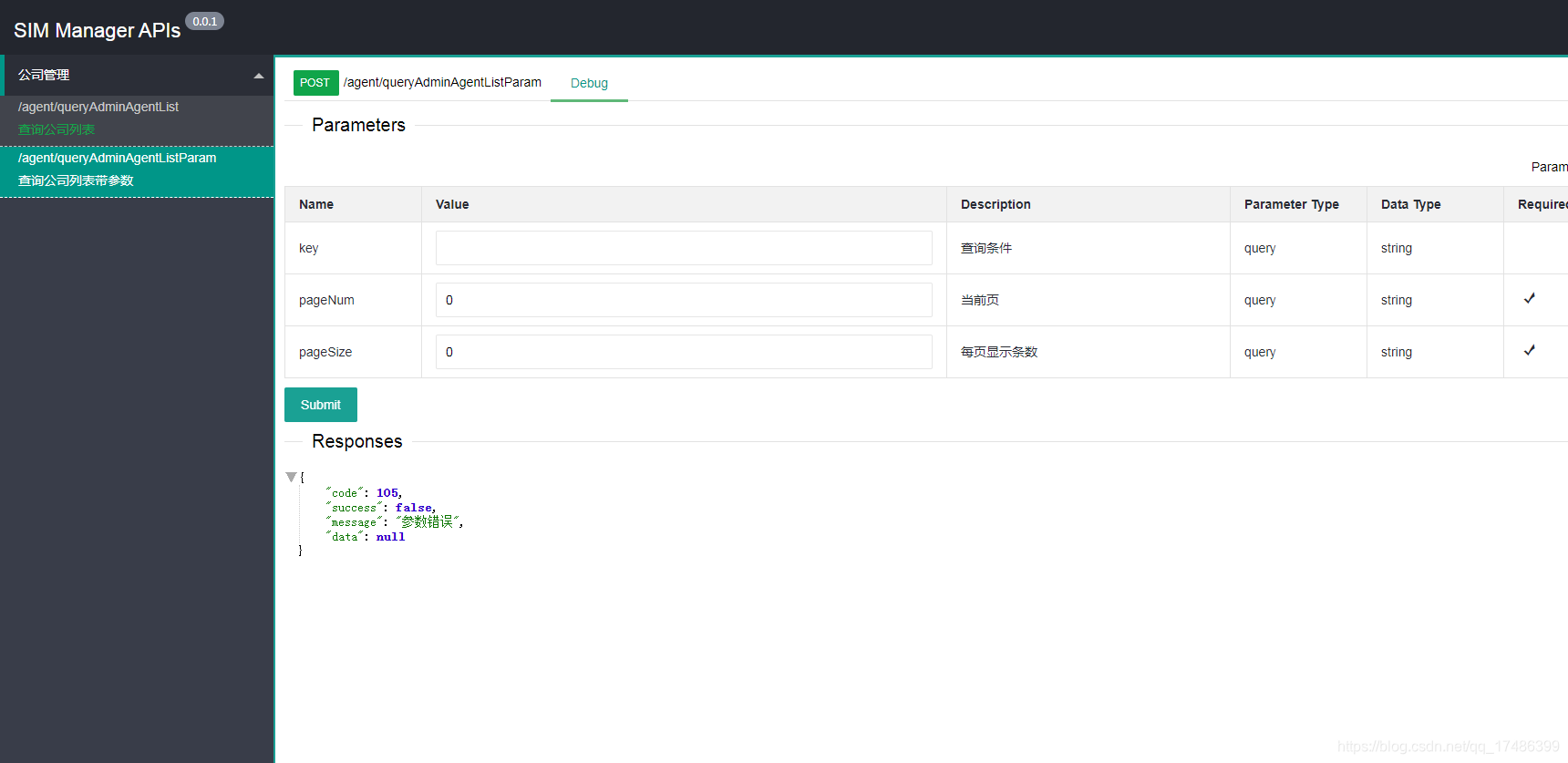