今天和大家分享在 JAVA 中使用 I/O 类进行基本的读写操作。
1.整行读取文本
BufferedReader 提供 readLine() 直接读取整行的方法,如果 readLine() 返回 null ,则表示读到了文件末尾。
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new FileReader("E:\\abc.txt"));
String s;
while ((s = br.readLine()) != null) {
System.out.println(s);
}
br.close();
}
* Reads text from a character-input stream, buffering characters so as to
* provide for the efficient reading of characters, arrays, and lines.
*
* <p> The buffer size may be specified, or the default size may be used. The
* default is large enough for most purposes.
2.读取内存中的文本
StringReader 可以读取内存中的文本,read() 方法依次读取每个字符,返回值为 int 型,故需要转换为 char 类型。
public static void main(String[] args) throws IOException {
StringReader sr = new StringReader("abcdef");
int c = 0;
while ((c=sr.read())!=-1){
System.out.println((char)c);
}
sr.close();
}
a
b
c
d
e
f
3.写入文本
FileWriter 可以往文件写入文本,第一个参数为文件路径,第二个参数为是否追加写(否则会覆盖原来的文本);
这里使用 BufferedWriter 包装开启缓冲输入;
最外层再次使用 PrintWriter 包装,提供整行写入的功能。
public static void main(String[] args) throws IOException {
PrintWriter pw = new PrintWriter(new BufferedWriter(new FileWriter("E:\\abc.txt", true)));
String[] strings = {
"Today", "is", "a", "good", "day"};
for (String string : strings) {
pw.println(string);
}
pw.close();
}
4.文本的存储和恢复
DataOutputStream 用来往流中写入不同类型的数据,DataInputStream 用来恢复数据(恢复的数据类型顺序必须和写入的顺序一致,否则会抛出 I/O 异常),可用来解决跨平台数据交换的问题。
public static void main(String[] args) throws IOException {
DataOutputStream out = new DataOutputStream(new BufferedOutputStream(new FileOutputStream("E" +
":\\abc.txt")));
out.writeDouble(1.1);
out.writeUTF("Tom");
out.writeDouble(2.2);
out.writeUTF("Jerry");
out.close();
DataInputStream in = new DataInputStream(new BufferedInputStream(new FileInputStream("E" +
":\\abc.txt")));
System.out.println(in.readDouble());
System.out.println(in.readUTF());
System.out.println(in.readDouble());
System.out.println(in.readUTF());
in.close();
}
1.1
Tom
2.2
Jerry
本次分享至此结束,希望本文对你有所帮助,若能点亮下方的点赞按钮,在下感激不尽,谢谢您的【精神支持】。
扫描二维码关注公众号,回复:
13489933 查看本文章
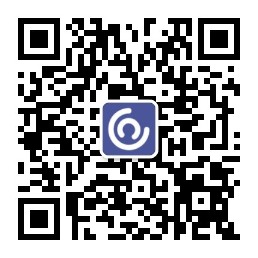
若有任何疑问,也欢迎与我交流,若存在不足之处,也欢迎各位指正!