如题 为了记录删除List内重复数据方法
比较简单,只是重写了equals方法,直接贴代码
public class Demo {
private static List<User> list = Arrays.asList(
new User("猪场", BigDecimal.valueOf(88.88), "英语"),
new User("鹅厂", BigDecimal.valueOf(85), "数学"),
new User("狗厂", BigDecimal.valueOf(77.99), "语文"),
new User("狗厂", BigDecimal.valueOf(77.99), "语文"),
new User("猪场", BigDecimal.valueOf(88.88), "英语"));
public static void main(String[] args) {
getDeleteRepeatList(list);
}
private static List<User> getDeleteRepeatList(List<User> oldList){
List<User> list = new ArrayList<User>();
if(CollectionUtils.isNotEmpty(oldList)){
for (User user : oldList) {
if(!list.contains(user)){
list.add(user);
}
}
}
System.out.println("删除重复后数据:");
if(CollectionUtils.isNotEmpty(list)){
for (User user : list) {
System.out.println(user.toString());
}
}
return list;
}
static class User{
private String userName;
private BigDecimal score;
private String name;
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public BigDecimal getScore() {
return score;
}
public void setScore(BigDecimal score) {
this.score = score;
}
public String getAge() {
return name;
}
public void setAge(String age) {
this.name = name;
}
public User(String userName, BigDecimal score, String name) {
super();
this.userName = userName;
this.score = score;
this.name = name;
}
public User() {
}
@Override
public String toString() {
return "姓名:"+ this.userName + ",科目:" + this.name + ",分数:" + this.score;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
if (this == obj) {
return true;
}
User user = (User) obj;
if (this.getAge() .compareTo(user.getAge())==0
&& this.getUserName().equals(user.getUserName())
&& this.getScore().compareTo(user.getScore())==0) {
return true;
}
return false;
}
}
}
执行前数据
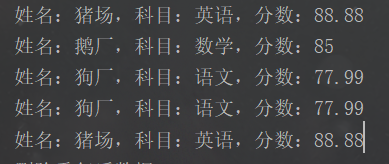
执行后数据
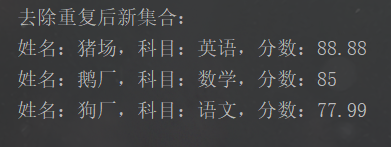