数据库的开发基本语法
数据库中常用的字段类型有
1、数值型:number(p[,s]):表示该类型最多保存 p位数,其中s位为小数
number(5):表示该类型最多存5位整数
number(5,2):表示该类型最多存3(5-2)位整数,2位小数
2、字符型:
定长字符串:char(n):表示该类型如果填写,则内容长度一定是n个字节,如果长度小于n则会用空格自动补齐到n个字节,如果超过n则报错
变长字符串:varchar2(n):表示该类型最多存n个字节,如果内容长度小于n则不会自动补齐,超过n则报错
char和varchar的不同:
1、char为定长字符串,varchar为变长字符串
2、char最多存2000个字节,varchar最多存4000个字节
3、根据char类型的查询效率要高于varchar类型的查询效率
3、日期类型:Date
操作数据库的DDL语句
create table student
(
stuno number(2),
stuname varchar2(20),
sex char(2),
height number(3,2),
birthday date
)
/**
插入 insert into 表名(列,列…) values(值,值…)
注意:值与列对应(类型和个数对应)
*/
insert into student(stuno,stuname,sex,height,birthday)
values(2,'李四','女',1.68,to_date('1996-09-08','yyyy-mm-dd'))
/**
查询 select 列,列… from 数据源(表)
注意:1、在查询语句中, select 和 from 必须有 select用于声明要显示的列 from 用于声明数据来源
2、如果只有 select from 则表示展示表中的每一条数据,如果要进行条件查询需要 select …from…where 查询条件
这样会展示数据源中符合条件的信息
*/
–显示所有学生的学号,姓名,性别
select stuno,stuname,sex from student
–查询所有的女学生的姓名和生日
select stuname,birthday from student where sex='女'
–查询身高在1.65到1.75之间的学员信息
select stuno,stuname,sex,height,birthday from student where height>=1.65 and height<=1.75
–查询所有身高高于1.70或者姓名是男的学员
select stuno,stuname,sex,height,birthday from student where height>=1.7 or sex='男'
/**
删除:
delete [from] 表名 where 条件:
从表中删除符合条件的信息
注意:删除语句where是关键,因为where决定了删除那些信息,如果没有where表示清空表中全部数据
*/
–删除 学号为 4的学员信息
delete from student where stuno=4;
commit;
/**
修改:
update 表名 set 列=修改的值,列=修改的值,… where 条件
将表中符合条件的对应字段进行修改
注意:修改where 是关键,因为where决定了那些条数据被修改,如果没有where则表示表中所有的数据都被修改
*/
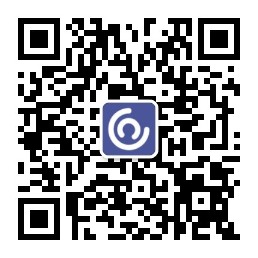
select stuno, stuname, sex, height, birthday from student
–将1号学员的姓名改为张三丰,身高改为1.85,生日改为1900-09-02
update student
set stuname = '张三丰',
height = 1.85,
birthday = to_date('1900-09-02', 'yyyy-mm-dd')
where stuno = 1
commit;
rollback;
对于null值的相关操作
–查询所有没有设置奖金的人
select * from emp where comm is null;--查询comm列状态为null的员工信息
--将员工姓名为ALLEN的员工奖金设置为null
UPDATE EMP set comm = null where ename ='ALLEN';--表中记录严格区分大小写
–注意任何数据与null进行运算结果依然为null
– nvl(列,默认值):如果该列为null则返回默认值,如果不为null正常返回
–显示所有的员工姓名,工资,奖金,和月收入(工资+奖金) (如果奖金为null则默认为0)
select ename ,sal,comm as 奖金,sal+nvl(comm,0) as 月收入 from emp
集合查询
列in (集合)如果列的值等于集合中的某一个值则表示true
–查询10部门和20部门的员工信息
select * from emp where deptno=10 or deptno=20
select * from emp where deptno in (10,20)
–查询不在10部门也不在20部门工作的员工
select * from emp where deptno != 10 and deptno <> 20
– 列 not in(集合) 如果列的值不等于集合中的某个值,就返回true
select * from emp where deptno not in(10,20)
模糊查询
模糊查询是对字符串类型进行部分匹配的查询
模糊查询的运算符为 like
占位符: % ,无限制占位 即占 >=0
_,占而且只占一位 即占 =1
–查询姓名以A开头的员工信息
select * from emp where ename like 'A%'
–查找姓名中有字符 A的员工
select * from emp where ename like '%A%'
–查询姓名以S结尾的员工
select * from emp where ename like '%S'
–查询姓名中第二个字母为A的员工信息
select * from emp where ename like '_A%'
时间相关的操作
–查询1982年之后入职的员工
select * from emp where hiredate>to_date('1982-01-01','yyyy-mm-dd')
–to_char(date,格式)将时间中指定的属性转为字符串
–查找入职时间为1987年的员工
select * from emp where to_char(hiredate,'yyyy')='1987'
–查找1987-04-19入职的员工
select * from emp where hiredate = to_date('1987-04-19','yyyy-mm-dd')
select * from emp where to_char(hiredate,'yyyy-mm-dd') = '1987-04-19'
–查找所有在4月份入职的员工
select * from emp where to_char(hiredate,'mm')='04'
对结果集进行排序显示
select ... from ... [where ] [order by 排序列1,排序列2]
–查询工资大于1000的员工,并按照部门编号升序排序,如果部门编号一致则按照工资倒序,如果工资一致按照员工号倒序
select * from emp where sal>1000 order by deptno asc,sal desc,empno desc
小结:建议大家根据业务上的需求来进行实际开发SQL,首先要一步一步去解决问题,根据你要实现的功能选择实现的方式(集合、子查询、内连、外连、函数),接下来我会为大家分享如何用存储过程、函数来处理业务上的逻辑。