while循环
while是最基本的循环
结构形式为:
while(布尔表达式)
{
//循环内容
}
布尔表达式是逻辑函数,常量只能是false和true
只要布尔表达式为true,循环就会一直执行下去
while 循环由四个结构组成:初始化,条件判断,循环体,迭代。
来看个简单的:(来吧,展示)
字符串的使用
什么是字符串?
一下有关内容,大多都来自于
原文链接:https://blog.csdn.net/practical_sharp/article/details/87550434
字符串是由多个字符组成的一段数据
Java中的字符串可以分为两大类:
1.String类
2.StringBuffer和StringBuilder类
String类是immutable(不可变的),不可修改,所有当我们用”=,+“这些运算的时候会重新生成一个新的String类的实例,在循环中使用String类的”+=“运算会带来一定的效率问题。
String类声明与创建
字符串声明:String stringName;
字符串创建:stringName = new String(字符串常量);或stringName = 字符串常量;
String name;
name = "Jack"; //String name = new String("Jack");
String类构造方法
1,public String(),无参构造方法,用来创建空字符串的String对象。
例如:String string1 = new String(); // 则有string1==null
2,public String(String value),用已知的字符串value创建一个String对象。
例如:String str2 = new String("Helllo,world!");则str2 = "Hello,world!"
String str3 = new String(str2);则str3 = "Hello,world!"
3,public String(char[] value),用字符数组value创建一个String对象。
例如:char[] value = {"a","b","c","d"};
String str4 = new String(value);//相当于String str4 = new String("abcd");
4,public String(char chars[], int startIndex, int numChars),用字符数组chars的startIndex开始的numChars个字符创建一个String对象。
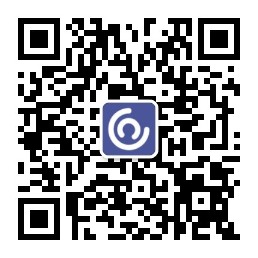
例如:char[] value = {"a","b","c","d"};
String str5 = new String(value, 1, 2);//相当于String str5 = new String("bc");
String类的常用方法
String类在java.lang包中,java使用String类创建一个字符串变量,字符串变量属于对象。java把String类声明的final类。**String类对象创建后不能修改,**由0或多个字符组成,包含在一对双引号之间。String类中有很多方法,可以查看API理解。
1、求字符串长度
public int length() //返回该字符串的长度
2、求字符串某一位置字符
public char charAt(int index)//返回字符串中指定位置的字符;注意字符串中第一个字符索引是0,最后一个是length()-1。
3、提取子串
用String类的substring方法可以提取字符串中的子串,该方法有两种常用参数:
- public String substring(int beginIndex) //该方法从beginIndex位置起,从当前字符串中取出剩余的字符作为一个新的字符串返回。
2)public String substring(int beginIndex, int endIndex) //该方法从beginIndex位置起,从当前字符串中取出到endIndex-1位置的字符作为一个新的字符串返回。
String str1 = new String("abcdefg");
String str2 = str1.substring(2);//str2 = "cdefg";
String str3 = str1.substring(2,5);//str3 = "cde";
4、字符串比较
1)public int compareTo(String anotherString)//该方法是对字符串内容按字典顺序进行大小比较,通过返回的整数值指明当前字符串与参数字符串的大小关系。若当前对象比参数大则返回正整数,反之返回负整数,相等返回0。
2)public int compareToIgnore(String anotherString)//与compareTo方法相似,但忽略大小写。
3)public boolean equals(Object anotherObject)//比较当前字符串和参数字符串,在两个字符串相等的时候返回true,否则返回false。
4)public boolean equalsIgnoreCase(String anotherString)//与equals方法相似,但忽略大小写
String name = "Liu Yankee";
name.compareTo("Hello,World!"); //name.compareTo("Hello,World!")返回负整数
name.compareTo("Yellow"); //name.compareTo("Yellow")返回正整数
name.compareTo("Liu Yankee"); //返回0
name.equal("Liu Kee"); //返回false
String name = "Liu YanKee";
System.out.println(name.equalsIgnoreCase("Liu YanKee")); //输出true
5、字符串连接
public String concat(String str)//将参数中的字符串str连接到当前字符串的后面,效果等价于"+"。
String name1 = "Tom";
String name2 = "son";
System.out.println(name1 + name2); //输出Tomson
6、字符串中单个字符查找
1)public int indexOf(int ch/String str)//用于查找当前字符串中字符或子串,返回字符或子串在当前字符串中从左边起首次出现的位置,若没有出现则返回-1。
2)public int indexOf(int ch/String str, int fromIndex)//改方法与第一种类似,区别在于该方法从fromIndex位置向后查找。
3)public int lastIndexOf(int ch/String str)//该方法与第一种类似,区别在于该方法从字符串的末尾位置向前查找。
4)public int lastIndexOf(int ch/String str, int fromIndex)//该方法与第二种方法类似,区别于该方法从fromIndex位置向前查
String name = "Liu YanKee";
name.indexOf("Kee"); //name.indexOf("Kee")==7
//name.indexOf("cdoihj")=-1
7、字符串中字符的大小写转换
1)public String toLowerCase()//返回将当前字符串中所有字符转换成小写后的新串
2)public String toUpperCase()//返回将当前字符串中所有字符转换成大写后的新串
String name ="Jack Ma";
System.out.println(name.toLowerCase());
System.out.println(name.toUpperCase());
输出:
jack ma
JACK MA
8、字符串中字符的替换
1)public String replace(char oldChar, char newChar)//用字符newChar替换当前字符串中所有的oldChar字符,并返回一个新的字符串。
2)public String replaceFirst(String regex, String replacement)//该方法用字符replacement的内容替换当前字符串中遇到的第一个和字符串regex相匹配的子串,应将新的字符串返回。
3)public String replaceAll(String regex, String replacement)//该方法用字符replacement的内容替换当前字符串中遇到的所有和字符串regex相匹配的子串,应将新的字符串返回。
String str = "asdzxcasd";
String str1 = str.replace('a','g');//str1 = "gsdzxcgsd";
String str2 = str.replace("asd","fgh");//str2 = "fghzxcfgh";
String str3 = str.replaceFirst("asd","fgh");//str3 = "fghzxcasd";
String str4 = str.replaceAll("asd","fgh");//str4 = "fghzxcfgh";
字符串与基本类型的转换
public byte[] getBytes(): 把字符串转换为字节数组。
public char[] toCharArray(): 把字符串转换为字符数组。
public static String valueOf(char[] chs): 把字符数组转成字符串。
public static String valueOf(int i): 把int类型的数据转成字符串。(String类的valueOf方法可以把任意类型的数据转成字符串。)
public String toLowerCase(): 把字符串转成小写。
public String toUpperCase(): 把字符串转成大写。
public String concat(String str): 把字符串拼接。
StringBuffer和StringBuilder类
既然在Java中已经存在了String类,那为什么还需要StringBuilder和StringBuffer类呢?事实上,StringBuilder和StringBuffer类拥有的成员属性以及成员方法基本相同,区别是StringBuffer类的成员方法前面多了一个关键字:synchronized,不用多说,这个关键字是在多线程访问时起到安全保护作用的,也就是说StringBuffer是线程安全的。
String、StringBuilder、StringBuffer三者的执行效率:
StringBuilder > StringBuffer > String