1、什么是链表?
C++ Lists(链表)
(1)Lists将元素按顺序储存在链表中. 与 向量(vectors)相比, 它允许快速的插入和删除,但是随机访问却比较慢.
(2)std::list
是 STL 提供的 双向链表 数据结构。能够提供线性复杂度的随机访问,以及常数复杂度的插入和删除。
2、相关操作:
assign() 给list赋值 back() 返回最后一个元素 begin() 返回指向第一个元素的迭代器 clear() 删除所有元素 empty() 如果list是空的则返回true end() 返回末尾的迭代器 erase() 删除一个元素 front() 返回第一个元素 get_allocator() 返回list的配置器 insert() 插入一个元素到list中 max_size() 返回list能容纳的最大元素数量 merge() 合并两个list pop_back() 删除最后一个元素 pop_front() 删除第一个元素 push_back() 在list的末尾添加一个元素 push_front() 在list的头部添加一个元素 rbegin() 返回指向第一个元素的逆向迭代器 remove() 从list删除元素 remove_if() 按指定条件删除元素 rend() 指向list末尾的逆向迭代器 resize() 改变list的大小 reverse() 把list的元素倒转 size() 返回list中的元素个数 sort() 给list排序 splice() 合并两个list swap() 交换两个list unique() 删除list中重复的元素
3、创建方式
#include <list>
// list构造 :
1. list<Typename T> s;
2. list<Typename T, Container> s;
/* list的 Container 需要满足有如下接口 :
* back()
* push_back()
* pop_back()
* 标准容器 std::vector / deque / list 满足这些要求
* 如使用 1 方式构造,默认容器使用 list
*/
4、元素访问
由于
list
的实现是链表,因此它不提供随机访问的接口。若需要访问中间元素,则需要使用迭代器。
front()
返回首元素的引用。back()
返回末尾元素的引用。
5、基本操作
list
类型还提供了一些针对其特性实现的 STL 算法函数。由于这些算法需要 随机访问迭代器,因此list
提供了特别的实现以便于使用。这些算法有splice()
、remove()
、sort()
、unique()
、merge()
等。
6、代码示例:
(1)、链表中的元素倒转
#include<iostream>
#include<list>
using namespace std;
int main()
{
list<int> l;
list<int>::iterator it;
for (int i = 10; i >= 1; i--)
l.insert(l.begin(),1,i);//头插法
cout << "倒转前:" << endl;
for (it = l.begin(); it != l.end(); it++)//迭代器遍历链表
cout << *it << ' ';
l.reverse();//倒转
cout <<endl<< "倒转后:" << endl;
for (it = l.begin(); it != l.end(); it++)//迭代器遍历链表
cout << *it<<' ';
return 0;
}
(2)、排序(sort()方法默认升序)
#include<iostream>
#include<list>
using namespace std;
int main()
{
list<int> l;
list<int>::iterator it;
for (int i = 10; i >= 1; i--)
l.insert(l.begin(),1,i);//头插法
cout << "倒转前:" << endl;
for (it = l.begin(); it != l.end(); it++)//迭代器遍历链表
cout << *it << ' ';
l.reverse();//倒转
l.sort();//排序(默认升序排列)
cout <<endl<< "倒转后:" << endl;
for (it = l.begin(); it != l.end(); it++)//迭代器遍历链表
cout << *it<<' ';
return 0;
}
(3)、使用list库求两个集合的交并集问题。
#include<iostream>
#include<list>
using namespace std;
int main()
{
list<int> l_1;
list<int> l_2;
list<int>::iterator it_1;
list<int>::iterator it_2;
//================================================A集合=====================
cout << "输入集合A:" << endl;
int A[5] = { 0 };
for (int i = 0; i < 5; i++)
cin >> A[i];
for (int i = 0; i < 5; i++)//A链表赋值
l_1.insert(l_1.end(), A[i]);//尾插法
//================================================B集合=====================
cout << "输入集合B:" << endl;
int B[5] = { 0 };
for (int i = 0; i < 5; i++)
cin >> B[i];
for (int i = 0; i < 5; i++)//B链表赋值
l_2.insert(l_2.end(), B[i]);
//==============================================求交集=======================
cout << "输出A,B交集:" << endl;
for (it_1 = l_1.begin(); it_1 != l_1.end(); it_1++)
{
for (it_2 = l_2.begin(); it_2 != l_2.end(); it_2++)
{
if (*it_1 == *it_2)
cout << *it_1 << ' ';
}
}
cout << endl;
//==============================================求并集======================
//l_1.splice(l_1.end(),l_2);//将l2表接在l1后面
l_1.merge(l_2);//合并两个列表
l_1.unique();//删除多余元素
cout << "输出A,B并集:" << endl;
for (it_1 = l_1.begin(); it_1 != l_1.end(); it_1++)
cout << *it_1 << ' ';
cout << endl;
return 0;
}
运行结果:
扫描二维码关注公众号,回复:
13281601 查看本文章
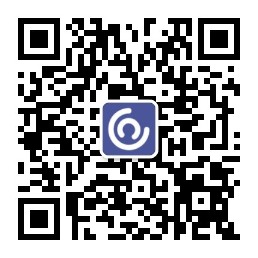