目录
1、去页面获取国际化的值,查看Thymeleaf的文档,找到message取值操作为: #{...}。去index.html中修改要替换的值.
2、在dashboard.html、list.html、404.html删除原来的导航栏和侧边栏,在原来的位置导入公共的导航栏和侧边栏。
一、环境配置
1、导入依赖
<!-- lombok -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<!-- 数据层 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.1</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
2、导入实体类
Department
package com.rk.domain;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.springframework.stereotype.Component;
@Data
@AllArgsConstructor
@NoArgsConstructor
@Component
public class Department {
private Integer id;
private String departmentName;
}
Employee
package com.rk.domain;
import lombok.Data;
import lombok.NoArgsConstructor;
import org.springframework.stereotype.Component;
import java.util.Date;
@Data
@NoArgsConstructor
@Component
public class Employee {
private Integer id;
private String lastName;
private String email;
private Integer gender;//1表示男 0表示女
private Department department;//部门
private Date birth;
}
3、导入mapp接口以及对应的配置文件
4、Maven资源导出,放入pom.xml中
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
<filtering>true</filtering>
</resource>
</resources>
二、导入下载好的静态页面模板及准备工作
1、css、js等放在static目录下
2、heml放在templates目录下
导入完成后的目录结构
3、首页实现
编写一个controller实现
@Controller
public class IndexController {
@RequestMapping("/")
public String index(){
return "index";
}
}
因为springboot使用的是Thymeleaf.org引擎,为了保证静态资源稳定,每个静态页面要导入空间,以及所有导入静态资源的地方都要用th:替换路径.
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<link th:href="@{/asserts/css/bootstrap.min.css}" rel="stylesheet">
在配置文件中关闭模板引擎的缓存
#关闭模板引擎缓存
spring.thymeleaf.cache=false
三、页面国际化
1、修改idea中所有编码
2、在resources下编写登录界面国际化的配置文件
login_zh_CN.properties
login.tip=请登录
login.password=密码
login.remember=记住我
login.username=用户名
login.btn=登录
login_en_US.properties
login.tip=Please sign in
login.password=Password
login.remember=Remember me
login.username=UserName
login.btn=Sign in
在application.properties中配置
spring.messages.basename=i18n.login
3、配置页面国际化值
1、去页面获取国际化的值,查看Thymeleaf的文档,找到message取值操作为: #{...}。去index.html中修改要替换的值.
现在登录页面已经识别为中文了
2、根据按钮切换中英文
处理按钮
<a class="btn btn-sm" th:href="@{/index.html(l='zh_CN')}">中文</a>
<a class="btn btn-sm" th:href="@{/index.html(l='en_US')}">English</a>
处理组件的类
package com.rk.config;
import org.springframework.stereotype.Component;
import org.springframework.util.StringUtils;
import org.springframework.web.servlet.LocaleResolver;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.util.Locale;
public class MyLocaleResolver implements LocaleResolver {
//请求解析
@Override
public Locale resolveLocale(HttpServletRequest request) {
String language = request.getParameter("l");
Locale locale = Locale.getDefault(); // 如果没有获取到就使用系统默认的
//如果请求链接不为空
if (!StringUtils.isEmpty(language)){
//分割请求参数
String[] split = language.split("_");
//国家,地区4
locale = new Locale(split[0],split[1]);
}
return locale;
}
@Override
public void setLocale(HttpServletRequest request, HttpServletResponse response, Locale locale) {
}
}
在自定义的MymvcConfig中去注册组件
//自定义的国际化组件
@Bean
public LocaleResolver localeResolver(){
return new MyLocaleResolver();
}
现在就可以自由切换中英文了
4、注意点
1、我们需要配置i18n文件
2、我们如果需要在项目中进行按钮自动切换,我们需要自定义一个组件LocaleResolver
3、将组件配置到spring容器@Bean中
四、登录+拦截器
1、禁用模板缓存
#禁用模板缓存
spring.thymeleaf.cache=false
2、登录
1、设置表单提交地址
<form class="form-signin" th:action="@{/user/login}" method="post">
2、登录的Controller的编写
package com.rk.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import javax.servlet.http.HttpSession;
@Controller
public class LoginController {
@RequestMapping("/user/login")
public String login(@RequestParam("username") String username,
@RequestParam("password") String password,
Model model, HttpSession session){
if(!StringUtils.isEmpty(username) &&password.equals("123456")){
//登录成功后将用户名存入session
session.setAttribute("loginuser",username);
//重定向到首页
return "redirect:/main.html";
}else {
//登录失败,存放错误信息
model.addAttribute("msg","用户名或密码错误");
return "index";
}
}
}
3、登录失败要将信息显示在前端界面
<!--判断是否显示,使用if, ${}可以使用工具类,可以看thymeleaf的中文文档-->
<p style="color: red" th:text="${msg}" th:if="${not #strings.isEmpty(msg)}"></p>
4、在MyMvcConfig中添加一个视图控制映射
registry.addViewController("/main.html").setViewName("dashboard")
3、登录
1、编写拦截器
package com.rk.config;
import org.springframework.web.servlet.HandlerInterceptor;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
//拦截器
public class LoginHandlerInterceptor implements HandlerInterceptor {
//登录成功之后,应该有用户的session
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
//从session获取数据
Object loginuser = request.getSession().getAttribute("loginuser");
if(loginuser==null)//未登录,返回登录界面
{
request.setAttribute("msg","没有权限,请先登录");
request.getRequestDispatcher("/index.html").forward(request,response);
return false;
}else {//登录 放行
return true;
}
}
}
2、将拦截器注册到我们的SpringMVC配置类当中
@Override
public void addInterceptors(InterceptorRegistry registry) {
// 注册拦截器,及拦截请求和要剔除哪些请求!
// 我们还需要过滤静态资源文件,否则样式显示不出来
registry.addInterceptor(new LoginHandlerInterceptor())
.addPathPatterns("/**")
.excludePathPatterns("/index.html","/","/user/login","/asserts/**");
}
3、在后台主页显示登录的用户信息
<a class="navbar-brand col-sm-3 col-md-2 mr-0" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#" th:text="${session.loginuser}"></a>
五、展示员工列表
1、员工列表页面跳转
1、将首页的侧边栏Customers改为员工管理
2、a标签添加请求
<a class="nav-link" th:href="@{/1 emps}">员工管理</a>
3、将lis.htmlt放在emp文件夹下
4、编写处理请求的controller
package com.rk.controller;
import com.rk.dao.EmployeeDao;
import com.rk.domain.Employee;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.ui.ModelMap;
import org.springframework.web.bind.annotation.RequestMapping;
import java.util.Collection;
@Controller
public class EmployeeController {
@Autowired
EmployeeDao employeeDao;
@GetMapping("/emps")
public String emplist(Model model){
Collection<Employee> employees = employeeDao.getAll();
model.addAttribute("emps",employees);
return "emp/list";
}
}
2、公共页面元素抽取
抽取三个页面公共的侧边栏和头部导航栏
1、提取公共部分创建commons.html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<!--公共模板-->
<!--头部导航栏-->
<nav class="navbar navbar-dark sticky-top bg-dark flex-md-nowrap p-0" th:fragment="topbar">
<a class="navbar-brand col-sm-3 col-md-2 mr-0" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#" th:text="${session.loginuser}"></a>
<input class="form-control form-control-dark w-100" type="text" placeholder="Search" aria-label="Search">
<ul class="navbar-nav px-3">
<li class="nav-item text-nowrap">
<a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">注销</a>
</li>
</ul>
</nav>
<!--侧边栏-->
<nav class="col-md-2 d-none d-md-block bg-light sidebar" th:fragment="sidebar">
<div class="sidebar-sticky">
<ul class="nav flex-column">
<li class="nav-item">
<a th:class="${active=='main.html'?'nav-link active':'nav-link'}" th:href="@{/index.html}">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-home">
<path d="M3 9l9-7 9 7v11a2 2 0 0 1-2 2H5a2 2 0 0 1-2-2z"></path>
<polyline points="9 22 9 12 15 12 15 22"></polyline>
</svg>
首页 <span class="sr-only">(current)</span>
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-file">
<path d="M13 2H6a2 2 0 0 0-2 2v16a2 2 0 0 0 2 2h12a2 2 0 0 0 2-2V9z"></path>
<polyline points="13 2 13 9 20 9"></polyline>
</svg>
Orders
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-shopping-cart">
<circle cx="9" cy="21" r="1"></circle>
<circle cx="20" cy="21" r="1"></circle>
<path d="M1 1h4l2.68 13.39a2 2 0 0 0 2 1.61h9.72a2 2 0 0 0 2-1.61L23 6H6"></path>
</svg>
Products
</a>
</li>
<li class="nav-item">
<a th:class="${active=='list.html'?'nav-link active':'nav-link'}" th:href="@{emps}">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-users">
<path d="M17 21v-2a4 4 0 0 0-4-4H5a4 4 0 0 0-4 4v2"></path>
<circle cx="9" cy="7" r="4"></circle>
<path d="M23 21v-2a4 4 0 0 0-3-3.87"></path>
<path d="M16 3.13a4 4 0 0 1 0 7.75"></path>
</svg>
员工管理
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-bar-chart-2">
<line x1="18" y1="20" x2="18" y2="10"></line>
<line x1="12" y1="20" x2="12" y2="4"></line>
<line x1="6" y1="20" x2="6" y2="14"></line>
</svg>
Reports
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-layers">
<polygon points="12 2 2 7 12 12 22 7 12 2"></polygon>
<polyline points="2 17 12 22 22 17"></polyline>
<polyline points="2 12 12 17 22 12"></polyline>
</svg>
Integrations
</a>
</li>
</ul>
<h6 class="sidebar-heading d-flex justify-content-between align-items-center px-3 mt-4 mb-1 text-muted">
<span>Saved reports</span>
<a class="d-flex align-items-center text-muted" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-plus-circle"><circle cx="12" cy="12" r="10"></circle><line x1="12" y1="8" x2="12" y2="16"></line><line x1="8" y1="12" x2="16" y2="12"></line></svg>
</a>
</h6>
<ul class="nav flex-column mb-2">
<li class="nav-item">
<a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-file-text">
<path d="M14 2H6a2 2 0 0 0-2 2v16a2 2 0 0 0 2 2h12a2 2 0 0 0 2-2V8z"></path>
<polyline points="14 2 14 8 20 8"></polyline>
<line x1="16" y1="13" x2="8" y2="13"></line>
<line x1="16" y1="17" x2="8" y2="17"></line>
<polyline points="10 9 9 9 8 9"></polyline>
</svg>
Current month
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-file-text">
<path d="M14 2H6a2 2 0 0 0-2 2v16a2 2 0 0 0 2 2h12a2 2 0 0 0 2-2V8z"></path>
<polyline points="14 2 14 8 20 8"></polyline>
<line x1="16" y1="13" x2="8" y2="13"></line>
<line x1="16" y1="17" x2="8" y2="17"></line>
<polyline points="10 9 9 9 8 9"></polyline>
</svg>
Last quarter
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-file-text">
<path d="M14 2H6a2 2 0 0 0-2 2v16a2 2 0 0 0 2 2h12a2 2 0 0 0 2-2V8z"></path>
<polyline points="14 2 14 8 20 8"></polyline>
<line x1="16" y1="13" x2="8" y2="13"></line>
<line x1="16" y1="17" x2="8" y2="17"></line>
<polyline points="10 9 9 9 8 9"></polyline>
</svg>
Social engagement
</a>
</li>
<li class="nav-item">
<a class="nav-link" href="http://getbootstrap.com/docs/4.0/examples/dashboard/#">
<svg xmlns="http://www.w3.org/2000/svg" width="24" height="24" viewBox="0 0 24 24" fill="none" stroke="currentColor" stroke-width="2" stroke-linecap="round" stroke-linejoin="round" class="feather feather-file-text">
<path d="M14 2H6a2 2 0 0 0-2 2v16a2 2 0 0 0 2 2h12a2 2 0 0 0 2-2V8z"></path>
<polyline points="14 2 14 8 20 8"></polyline>
<line x1="16" y1="13" x2="8" y2="13"></line>
<line x1="16" y1="17" x2="8" y2="17"></line>
<polyline points="10 9 9 9 8 9"></polyline>
</svg>
Year-end sale
</a>
</li>
</ul>
</div>
</nav>
2、在dashboard.html、list.html、404.html删除原来的导航栏和侧边栏,在原来的位置导入公共的导航栏和侧边栏。
<!--导入顶部导航栏-->
<div th:replace="~{commons/commons::topbar}"></div>
<!--导入侧边栏 给组件传递active参数,判断是否激活-->
<div th:replace="~{commons/commons::sidebar(active='main.html')}"></div>
3、激活侧边栏
1、将首页的超链接地址改到项目中
2、我们在a标签中加一个判断,使用class改变标签的值;
<a class="nav-link active"
th:class="${active=='main.html'?'nav-link active':'nav-link'}"
th:href="@{/main.html}">
首页
</a>
<a class="nav-link"
th:class="${active=='emps'?'nav-link active':'nav-link'}"
th:href="@{/emps}">员工管理
</a>
3、修改请求连接
<div th:replace="~{commons/bar::sitebar(active='main.html')}"></div>
<div th:replace="~{commons/bar::sitebar(active='emps')}"></div>
3、员工信息页面展示
list.html
<main role="main" class="col-md-9 ml-sm-auto col-lg-10 pt-3 px-4">
<div class="table-responsive">
<table class="table table-striped table-sm">
<thead>
<tr>
<th>id</th>
<th>lastName</th>
<th>email</th>
<th>gender</th>
<th>department</th>
<th>birth</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr th:each="emp:${emps}">
<td th:text="${emp.id}"></td>
<td th:text="${emp.lastName}"></td>
<td th:text="${emp.email}"></td>
<td th:text="${emp.gender}==0?'女':'男'"></td>
<td th:text="${emp.department.getDepartmentName()}"></td>
<td th:text="${#dates.format(emp.birth,'yyyy-MM-dd HH:mm:ss')}"></td>
<td>
<button class="btn btn-sm btn-primary">编辑</button>
<button class="btn btn-sm btn-danger">删除</button>
</td>
</tr>
</tbody>
</table>
</div>
</main>
注意:性别要用表达式转换 日期要使用格式化转换工具:${#dates.format(Date date,'格式')}
六、添加员工
1、添加员工按钮
<h2>
<a class="btn btn-sm btn-success" th:href="@{/toaddemp}">添加员工</a>
</h2>
2、跳转到添加员工页面
1、编写跳转controller
@RequestMapping("/toaddemp")
public String toadd(Model model){
//查出所有部门
Collection<Department> departments = departmentdao.getDepartments();
model.addAttribute("departments",departments);
return "emp/addEmp";
}
2、添加页面addEmp.html编写
<form>
<div class="form-group">
<label>LastName</label>
<input type="text" name="lastName" class="form-control" placeholder="rk">
</div>
<div class="form-group">
<label>Email</label>
<input type="email" name="email" class="form-control"
placeholder="[email protected]">
</div>
<div class="form-group">
<label>Gender</label><br/>
<div class="form-check form-check-inline">
<input class="form-check-input" type="radio" name="gender"
value="1">
<label class="form-check-label">男</label>
</div>
<div class="form-check form-check-inline">
<input class="form-check-input" type="radio" name="gender"
value="0">
<label class="form-check-label">女</label>
</div>
</div>
<div class="form-group">
<label>department</label>
<select class="form-control" name="department">
<option th:each="detp:${departments}" th:text="${detp.departmentName}" th:value="${detp.id}"></option>
</select>
</div>
<div class="form-group">
<label>Birth</label>
<input type="text" name="birth" class="form-control" placeholder="">
</div>
<button type="submit" class="btn btn-primary">添加</button>
</form>
注意:部门信息下拉框应该选择的是我们提供的数据
添加页面其它部分的代码和list.html相同,上面只写了表单部分
3、添加员工成功,返回首页
1、添加界面的form表单提交地址
<form th:action="@{/1 emp}" method="post">
2、编写增加controller
@PostMapping("/addemp")
public String addemp(Employee employee){
employeeDao.save(employee);//调用底层业务方法 保存员工信息
return "redirect:/emps";//重定向到查询的controller
}
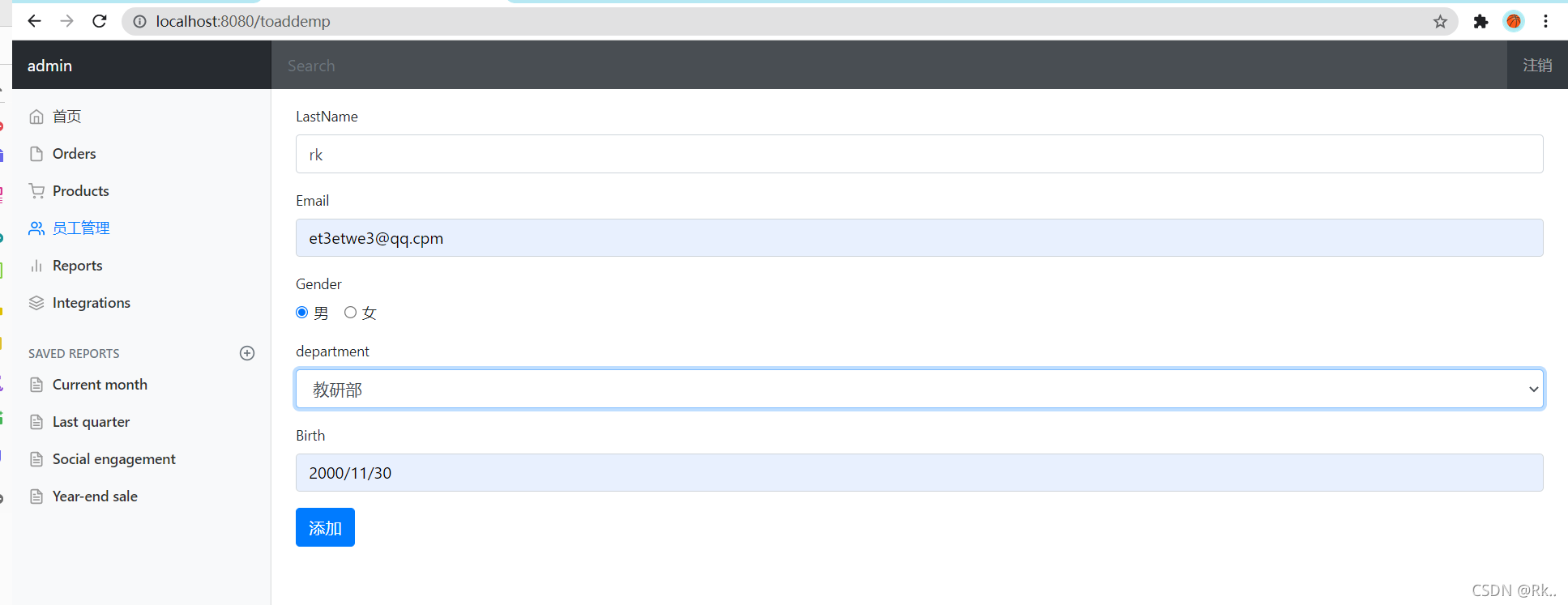
七、修改员工信息
1、编辑按钮路径
<a class="btn btn-sm btn-primary" th:href="@{/toupdate(id=${emp.id})}">编辑</a>
请求信息是:/toupdate?id=xxx
2、跳转到修改员工页面
1、编写跳转controller
@RequestMapping("/toupdate")
public String toUpdate(int id,Model model){
//根据id查询该员工
Employee employee = employeeDao.getEmployee(id);
//将员工信息返回到首页
model.addAttribute("emp",employee);
//查出所有部门,提供选择
Collection<Department> departments = departmentdao.getDepartments();
model.addAttribute("departments",departments);
return "emp/updateEmp";
}
2、编写修改页面updateEmp.html
<form th:action="@{/updateemp}" method="post">
<input type="hidden" name="id" th:value="${emp.id}">
<div class="form-group">
<label>LastName</label>
<input type="text" name="lastName" class="form-control" th:value="${emp.lastName}">
</div>
<div class="form-group">
<label>Email</label>
<input type="email" name="email" class="form-control"
placeholder="[email protected]" th:value="${emp.email}">
</div>
<div class="form-group">
<label>Gender</label><br/>
<div class="form-check form-check-inline">
<input class="form-check-input" type="radio" name="gender"
value="1" th:checked="${emp.gender==1}">
<label class="form-check-label">男</label>
</div>
<div class="form-check form-check-inline">
<input class="form-check-input" type="radio" name="gender"
value="0" th:checked="${emp.gender==0}">
<label class="form-check-label">女</label>
</div>
</div>
<div class="form-group">
<label>department</label>
<select class="form-control" name="department.id">
<option th:each="detp:${departments}"
th:selected="${detp.id==emp.department}"
th:text="${detp.departmentName}"
th:value="${detp.id}"></option>
</select>
</div>
<div class="form-group">
<label>Birth</label>
<input th:value="${#dates.format(emp.birth,'yyyy-MM-dd HH:mm:ss')}" type="text" name="birth" class="form-control">
</div>
<button type="submit" class="btn btn-primary">修改</button>
</form>
复制增加页面,修改表单,注意: 1、男女选则 2、department的默认 3 、生日日期赋值
3、添加员工成功,返回首页
1、修改界面的form表单提交地址
<form th:action="@{/updateemp}" method="post">
2、编写修改Controller
@RequestMapping("/updateemp")
public String Update(Employee employee){
employeeDao.save(employee);//调用底层业务方法 保存员工信息
return "redirect:/emps";//重定向到查询的controller
}
八、删除及404处理
1、删除
1、删除按钮路径
<a class="btn btn-sm btn-danger" th:href="@{/deleteEmp(id=${emp.id})}">删除</a>
2、编写删除Controller
@RequestMapping("/deleteEmp")
public String delete(int id){
employeeDao.deleteByid(id);
return "redirect:/emps";//重定向到查询的controller
}
2、404处理
在templates下面创建一个error的目录,把404.html放进该目录就ok了
3、注销
1、注销按钮
<a class="nav-link" th:href="@{/user/logout}">注销</a>
2、编写注销Controller
@RequestMapping("/user/logout")
public String logout(HttpSession session){
session.removeAttribute("loginuser");//移除session
return "redirect:/index.html";//重定向到登录页面
}