字符串逆序
法一:利用反向迭代器reverse_iterator:rbegin()和rend():
c.begin() 返回一个迭代器,它指向容器c的第一个元素
c.end() 返回一个迭代器,它指向容器c的最后一个元素的下一个位置
c.rbegin() 返回一个逆序迭代器,它指向容器c的最后一个元素
c.rend() 返回一个逆序迭代器,它指向容器c的第一个元素前面的位置
string str1("1234567890");
string str2(str1.rbegin(), str1.rend());
这样的话逆序string就保存在str2里了。要是想保存在原string里就这样:
string str1("1234567890");
str1 = string(str1.rbegin(), str1.rend());
法二:利用成员函数assign
string &operator=(const string &s); 把字符串s赋给当前字符串
string &assign(const char *s); 用c类型字符串s赋值
string &assign(const char *s,int n); 用c字符串s开始的n个字符赋值
string &assign(const string &s); 把字符串s赋给当前字符串
string &assign(int n,char c); 用n个字符c赋值给当前字符串
string &assign(const string &s,int start,int n); 把字符串s中从start开始的n个字符赋给当前字符串
string &assign(const_iterator first,const_itertor last); 把first和last迭代器之间的部分赋给字符串
函数以下列方式赋值:
用str为字符串赋值;
用str的开始num个字符为字符串赋值;
用str的子串为字符串赋值,子串以index索引开始,长度为len;
用num个字符ch为字符串赋值。
void assign(input_iteratorstart,input_iteratorend );
void assign( size_type num, constTYPE&val );
assign() 函数要么将区间[start, end)的元素赋到当前vector,或者赋num个值为val的元素到vector中.这个函数将会清除掉为vector赋值以前的内容。
代码:
扫描二维码关注公众号,回复:
13195731 查看本文章
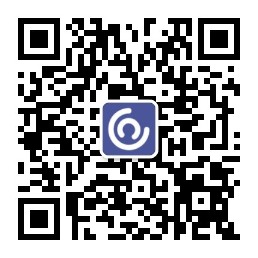
string str1("1234567890");
str1.assign(str1.rbegin(), str1.rend());
别的大佬的讲解,也是我参考的文章:传送门
法三:reverse
template <class BidirectionalIterator>
void reverse ( BidirectionalIterator first, BidirectionalIterator last)
{
while ((first!=last)&&(first!=--last))
swap (*first++,*last);
reverse函数功能是逆序(或反转),多用于字符串、数组、容器。头文件是#include
reverse函数用于反转在[first,last)范围内的顺序(包括first指向的元素,不包括last指向的元素),reverse函数无返回值
eg.
string str="hello world , hi";
reverse(str.begin(),str.end());//str结果为 ih , dlrow olleh
vector<int> v = {
5,4,3,2,1};
reverse(v.begin(),v.end());//容器v的值变为1,2,3,4,5
注意!!!reverse区间是左闭右开的!!(但是做题的时候好像没什么影响)
大佬讲解:传送门
附上几种方法的完整代码:
#include <bits/stdc++.h>
using namespace std;
string str;
int main(){
getline(cin, str);
reverse(str.begin(), str.end());
cout << str;
return 0;
}
#include <bits/stdc++.h>
using namespace std;
string str;
int main(){
getline(cin, str);
reverse(str.begin(), str.end());
int len = str.length();
for (int i = 0; i < len; ++i){
//ab 长度为2 但对应0 1,所以不能给len+等于好
cout << str[i];
}
return 0;
}
#include <bits/stdc++.h>
using namespace std;
string str;
int main(){
getline(cin, str);
int len = str.length();
for (int i = 0; i < len; ++i){
cout << str[len - i - 1];
}
return 0;
}
#include <iostream>
#include <algorithm>
#include <string>
using namespace std;
string str;
int main(){
getline(cin, str);
string sc(str.rbegin(), str.rend());
cout << sc << endl;
return 0;
}