文章目录
String类
概述
字符串广泛应用 在 Java 编程中,在 Java 中字符串属于对象,Java 提供了 String 类来创建和操作字符串。
特点
- 不可变性: 字符串是常量,创建之后不可改变
- 共享性: 字符串字面值存储在字符串池中,可以共享,字符串池位于堆中的方法区
创建字符串
有两种方式:
-
String str = "中国";
这种方式会产生一个对象,在字符串池中存储。
package com.ibelifly.commonclass.string; public class Test1 { public static void main(String[] args) { String s1="中国"; System.out.println(s1); } }
-
String str2=new String("中国");
这种方式会产生两个对象,堆中和字符串池中各存储一个。
package com.ibelifly.commonclass.string; public class Test1 { public static void main(String[] args) { String s2=new String("中国"); System.out.println(s2); } }
注意: 这种方式虽然会产生两个对象,但实际上堆中的对象并没有被赋值为
中国
,而是直接指向常量池中的中国
,可用下图简单理解这一过程:
不可变性和共享性详解
-
不可变性
package com.ibelifly.commonclass.string; public class Test1 { public static void main(String[] args) { String s1="中国"; System.out.println(s1); System.out.println(s1.hashCode()); s1="China"; System.out.println(s1); System.out.println(s1.hashCode()); } }
注意: 因为字符串是常量,创建之后不可改变,所以当
s1
被重新赋值为China
时,并不会改变原来中国
的值,而是会在常量池(字符串池)中重新创建一个China
常量,故s1
指向的内存地址会发生改变,返回的哈希值会发生改变。我们可以用下图简单理解一下这一过程: -
共享性
package com.ibelifly.commonclass.string; public class Test3 { public static void main(String[] args) { String s1="中国"; System.out.println(s1); System.out.println(s1.hashCode()); String s2="中国"; System.out.println(s2); System.out.println(s2.hashCode()); } }
注意: 在声明一个
s1
变量并创建一个中国
对象后,再度声明一个s2
变量,并赋予相同的中国
字符串时,由于常量池中已有中国
对象,故不会再为s2
创建新的对象,而是将已有的中国
对象的地址赋给s2
,故s1
和s2
返回哈希值相同。可用下图简单理解这一过程:但是当使用
new
关键字创建字符串时,即使赋值为同一字符串,两个变量所指向的地址也是不同的,例如:package com.ibelifly.commonclass.string; public class Test4 { public static void main(String[] args) { String s1=new String("中国"); String s2=new String("中国"); System.out.println(s1==s2); } }
这是因为使用
new
关键字创建字符串,还会在堆中创建对象,每创建一个新对象都会开辟新的内存空间,但是由于常量池中已有中国
对象,故堆中两个新对象指向同一份常量池中的中国
数据。图解:
1. length()方法
概述
length()方法用于返回字符串的长度。
语法
public int length()
举例
package com.ibelifly.commonclass.string;
public class Test5 {
public static void main(String[] args) {
String s="我爱我中国";
System.out.println(s.length());
}
}
2. charAt() 方法
概述
charAt() 方法用于返回指定索引处的字符。索引范围为从 0 到 length() - 1。
语法
public char charAt(int index)
index – 字符的索引。
举例
package com.ibelifly.commonclass.string;
public class Test5 {
public static void main(String[] args) {
String s="我爱我中国";
System.out.println(s.charAt(0));
}
}
注意: 当索引范围越界时,会抛出字符串索引越界异常StringIndexOutOfBoundsException
。
3. contains() 方法
概述
contains() 方法用于判断字符串中是否包含指定的字符或字符串。
语法
public boolean contains(CharSequence chars)
chars – 要判断的字符或字符串。
返回值
如果包含指定的字符或字符串返回 true,否则返回 false。
举例
package com.ibelifly.commonclass.string;
public class Test5 {
public static void main(String[] args) {
String s="我爱我中国";
System.out.println(s.contains("中国"));
System.out.println(s.contains("China"));
}
}
4. toCharArray() 方法
概述
toCharArray() 方法将字符串转换为字符数组。
语法
public char[] toCharArray()
返回值
字符数组。
举例
package com.ibelifly.commonclass.string;
import java.lang.reflect.Array;
import java.util.Arrays;
public class Test5 {
public static void main(String[] args) {
String s="我爱我中国";
System.out.println(s.toCharArray());
System.out.println(Arrays.toString(s.toCharArray()));
}
}
5. lastIndexOf(int ch) 方法
概述
lastIndexOf() 方法有以下四种形式:
- public int lastIndexOf(int ch): 返回指定字符在此字符串中最后一次出现处的索引,如果此字符串中没有这样的字符,则返回 -1。
- public int lastIndexOf(int ch, int fromIndex): 返回指定字符在此字符串中最后一次出现处的索引,从指定的索引处开始进行反向搜索,如果此字符串中没有这样的字符,则返回 -1。
- public int lastIndexOf(String str): 返回指定子字符串在此字符串中最后一次出现的索引,如果此字符串中没有这样的字符串,则返回 -1。
- public int lastIndexOf(String str, int fromIndex): 返回指定子字符串在此字符串中最后一次出现处的索引,从指定的索引开始反向搜索,如果此字符串中没有这样的字符,则返回 -1。
注意: 上述提到的反向搜索指的是,在搜索过程中,从指定位置开始从右到左检索字符串,但在返回结果中,还是从起始位置 (0)开始,截至指定的索引处,从左到右计算子字符串(或字符)最后出现的位置。
语法
public int lastIndexOf(int ch)
或
public int lastIndexOf(int ch, int fromIndex)
或
public int lastIndexOf(String str)
或
public int lastIndexOf(String str, int fromIndex)
ch – 字符。
fromIndex – 开始搜索的索引位置。
str – 要搜索的子字符串。
返回值
指定子字符串在字符串中第一次出现处的索引值。
举例
package com.ibelifly.commonclass.string;
import java.lang.reflect.Array;
import java.util.Arrays;
public class Test5 {
public static void main(String[] args) {
String s="我爱我中国";
System.out.print("查找字符 我 最后出现的位置 :");
System.out.println(s.lastIndexOf('我'));
System.out.print("从第1个位置查找字符 我 最后出现的位置 :");
System.out.println(s.lastIndexOf('我',1));
System.out.print("子字符串 中国 最后出现的位置:");
System.out.println(s.lastIndexOf("中国"));
System.out.print("从2个位置开始搜索子字符串 中国 最后出现的位置 :");
System.out.println(s.lastIndexOf("中国",2));
}
}
6. trim() 方法
概述
trim() 方法用于删除字符串的头尾空白符。
语法
public String trim()
返回值
删除头尾空白符的字符串。
举例
package com.ibelifly.commonclass.string;
public class Test7 {
public static void main(String[] args) {
String s=" Hello world ";
System.out.println(s.trim());
}
}
7. toUpperCase()与toLowerCase() 方法
概述
- toUpperCase() 方法将字符串所有字符转换为大写。
- toLowerCase() 方法将字符串所有字符转换为小写。
语法
public String toUpperCase()
public String toLowerCase()
返回值
转换后的字符串。
举例
package com.ibelifly.commonclass.string;
public class Test7 {
public static void main(String[] args) {
String s="Hello World";
System.out.println(s.toUpperCase());
System.out.println(s.toLowerCase());
}
}
8. endsWith() 与startsWith() 方法方法
概述
- endsWith() 方法用于测试字符串是否以指定的后缀结束。
- startsWith() 方法用于检测字符串是否以指定的前缀开始。
语法
public boolean endsWith(String suffix)
public boolean startsWith(String prefix)
suffix – 指定的后缀。
prefix – 指定的前缀。
返回值
endsWith(): 如果参数表示的字符序列是此对象表示的字符序列的后缀,则返回 true;否则返回 false。注意,如果参数是空字符串,或者等于此 String 对象(用 equals(Object) 方法确定),则结果为 true。
startsWith(): 如果字符串以指定的前缀开始,则返回 true;否则返回 false。
举例
package com.ibelifly.commonclass.string;
public class Test7 {
public static void main(String[] args) {
String s="HelloWorld.java";
System.out.println(s.endsWith(".java"));
System.out.println(s.endsWith(""));
System.out.println(s.endsWith("HelloWorld.java"));
System.out.println(s.endsWith(".jav"));
System.out.println(s.startsWith("h"));
}
}
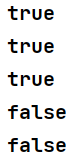
9. replace() 方法
概述
replace() 方法通过用 newChar 字符替换字符串中出现的所有 searchChar 字符,并返回替换后的新字符串。
语法
public String replace(char searchChar, char newChar)
searchChar – 原字符。
newChar – 新字符。
返回值
替换后生成的新字符串。
举例
package com.ibelifly.commonclass.string;
public class Test8 {
public static void main(String[] args) {
String s="Java is the best programming language.Java is great.";
System.out.println(s.replace("Java","Python"));
}
}
10. split() 方法
概述
split() 方法根据匹配给定的正则表达式来拆分字符串。
注意: . 、 $、 | 和 ***** 等转义字符,必须得加 \。
**注意:**多个分隔符,可以用 | 作为连字符。
语法
public String[] split(String regex, int limit)
regex – 正则表达式分隔符。
limit – 分割的份数。
返回值
字符串数组。
举例
package com.ibelifly.commonclass.string;
public class Test9 {
public static void main(String[] args) {
String s1 ="Welcome-to-learn-JAVA";
System.out.println("- 分隔符返回值 :" );
for (String str: s1.split("-")){
System.out.println(str);
}
System.out.println("");
System.out.println("- 分隔符设置分割份数返回值 :" );
for (String str: s1.split("-", 4)){
System.out.println(str);
}
System.out.println("");
String s2 ="www.JAVA.com";
System.out.println("转义字符返回值 :" );
for (String str: s2.split("\\.", 3)){
System.out.println(str);
}
System.out.println("");
String s3 = "account=? and uu =? or n=?";
System.out.println("多个分隔符返回值 :" );
for (String str: s3.split("and|or")){
System.out.println(str);
}
}
}
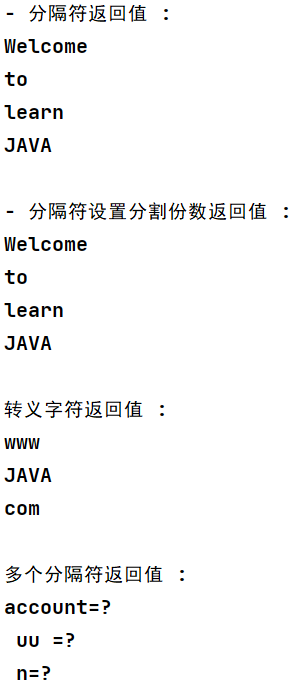
11. equals()与equalsIgnoreCase()方法
概述
- equals() 方法用于将字符串与指定的对象比较。String 类中重写了 equals() 方法用于比较两个字符串的内容是否相等。
- equalsIgnoreCase() 方法用于将字符串与指定的对象比较,不考虑大小写。
语法
public boolean equals(Object anObject)
public boolean equalsIgnoreCase(String anotherString)
anObject – 与字符串进行比较的对象。
返回值
如果给定对象与字符串相等,则返回 true;否则返回 false。
举例
package com.ibelifly.commonclass.string;
public class Test10 {
public static void main(String[] args) {
String s1="hello";
String s2="HELLO";
System.out.println(s1.equals(s2));
System.out.println(s1.equalsIgnoreCase(s2));
}
}
12. compareTo() 方法
概述
compareTo() 方法按字典顺序比较两个字符串。
语法
int compareTo(String anotherString)
anotherString – 要比较的字符串。
返回值
返回值是整型,它是先比较对应字符的大小(ASCII码顺序),如果第一个字符和参数的第一个字符不等,结束比较,返回他们之间的长度ASCII码差值,如果第一个字符和参数的第一个字符相等,则以第二个字符和参数的第二个字符做比较,以此类推,直至比较的字符或被比较的字符有一方结束。
- 如果参数字符串等于此字符串,则返回值 0;
- 如果此字符串小于字符串参数,则返回一个小于 0 的值;
- 如果此字符串大于字符串参数,则返回一个大于 0 的值。
说明:
如果第一个字符和参数的第一个字符不等,结束比较,返回第一个字符的ASCII码差值。
如果第一个字符和参数的第一个字符相等,则以第二个字符和参数的第二个字符做比较,以此类推,直至不等为止,返回该字符的ASCII码差值。 如果两个字符串不一样长,可对应字符又完全一样,则返回两个字符串的长度差值。
举例
package com.ibelifly.commonclass.string;
public class Test11 {
public static void main(String[] args) {
String s1="abc";
String s2="bcd";
String s3="abc123";
System.out.println(s1.compareTo(s2));
System.out.println(s2.compareTo(s1));
System.out.println(s1.compareTo(s3));
}
}
13. substring() 方法
概述
substring() 方法返回字符串的子字符串。
语法
public String substring(int beginIndex)
或
public String substring(int beginIndex, int endIndex)
beginIndex – 起始索引(包括), 索引从 0 开始。
endIndex – 结束索引(不包括)。
举例
package com.ibelifly.commonclass.string;
public class Test12 {
public static void main(String[] args) {
String s = "This is text";
System.out.println(s.substring(5));
System.out.println(s.substring(5, 10));
}
}
参考视频:https://www.bilibili.com/video/BV1vt4y197nY?p=20
参考文章:https://www.runoob.com/java/java-string.html