Springboot与MybatisPlus整合
数据表
CREATE DATABASE /*!32312 IF NOT EXISTS*/`jt` /*!40100 DEFAULT CHARACTER SET utf8 */;
USE `jt`;
/*Table structure for table `demo_user` */
DROP TABLE IF EXISTS `demo_user`;
CREATE TABLE `demo_user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` char(40) DEFAULT NULL,
`age` int(11) DEFAULT NULL,
`sex` char(40) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `cc` (`id`),
KEY `user_index` (`name`)
) ENGINE=InnoDB AUTO_INCREMENT=231 DEFAULT CHARSET=utf8;
/*Data for the table `demo_user` */
insert into `demo_user`(`id`,`name`,`age`,`sex`) values (1,'黑熊精',3000,'男'),(3,'金角大王',3000,'男'),(4,'银角大王',4000,'男'),(5,'唐僧',30,'男'),(6,'孙悟空',500,'男'),(7,'白龙驴',2000,'男'),(8,'八戒',502,'男'),(9,'沙悟净',503,'男'),(11,'小乔',17,'女'),(12,'貂蝉',18,'女'),(16,'黄月英',18,'女'),(17,'孙尚香',18,'女'),(18,'甄姬c',20,'女'),(21,'孙尚香D',18,'女'),(22,'刘备',40,'男'),(23,'潘凤',35,'男'),(24,'陆逊',33,'男'),(25,'关羽',40,'男'),(27,'阿科',20,'女'),(31,'王昭君',19,'女'),(38,'貂蝉',18,'女'),(39,'西施',18,'女'),(40,'严真煌',16,'女'),(41,'白骨精',3000,'女'),(43,'小乔',19,'男'),(44,'大乔',19,'女'),(46,'不知火舞',18,'女'),(49,'小兰兰',18,'男'),(50,'柳鹏林',18,'男'),(51,'妲己',18,'男'),(52,'如花',17,'男'),(53,'小明',18,'男'),(55,'金刚葫芦娃',7,'男'),(58,'马云',46,'男'),(62,'赵云',18,'男'),(66,'诺克赛斯之手',100,'男'),(68,'复仇炎魂',2000,'男'),(173,'年兽',5000,'公'),(182,'霸波尔奔',4000,'男'),(194,'齐天大圣',600,'男'),(196,'猪八戒',8000,'男'),(227,'小法',20,'男');
准备代码层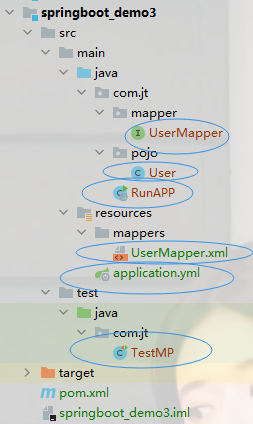
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>org.example</groupId>
<artifactId>springboot_demo3</artifactId>
<version>1.0-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.3</version>
<relativePath/>
</parent>
<properties>
<!--指定JDK版本-->
<java.version>1.8</java.version>
<!--跳过测试类打包-->
<skipTests>true</skipTests>
</properties>
<!--按需导入
历史说明: 2010 原来SSM 需要手动的编辑大量的的配置文件
思想: SpringBoot使用体现了"开箱即用"的思想
-->
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<!--Springboot的启动器 在内部已经将整合的配置写好,实现拿来就用-->
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!--引入插件lombok 自动的set/get/构造方法插件 -->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<!--引入数据库驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<!--springBoot数据库连接 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<!--spring整合mybatis-plus 删除mybatis的包 -->
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.3</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<!--build标签
springboot项目在打包部署发布时,需要依赖maven工具API
如果不添加该插件,则直接影响项目发布
-->
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>2.5.3</version>
</plugin>
</plugins>
</build>
</project>
User类
package com.jt.pojo;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.experimental.Accessors;
import java.io.Serializable;
@Data
@Accessors(chain = true)
//1.将对象与表进行关联
//规则1: 如果表名与对象名一致 则名称可以省略
//规则2: 如果字段名与属性名一致, 则注解可以省略
@TableName("demo_user")
public class User implements Serializable {
//2.主键自增/非空/UUID 生成唯一编号
@TableId(type=IdType.AUTO)
private Integer id;
//3.标识属性与字段的映射.
//@TableField("name") 休息15分钟
private String name;
private Integer age;
private String sex;
}
UserMapper接口
package com.jt.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.jt.pojo.User;
import org.apache.ibatis.annotations.Mapper;
import java.util.List;
/**
* 1.规则1:继承BaseMapper时,必须添加泛型对象
* 2.规则2:自己的方法不要与接口方法重名
*/
public interface UserMapper extends BaseMapper<User> {
}
启动类
package com.jt;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
@MapperScan("com.jt.mapper")
public class RunAPP {
public static void main(String[] args) {
SpringApplication.run(RunAPP.class,args);
}
}
映射文件UserMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.jt.mapper.UserMapper">
</mapper>
配置文件application.yml
#端口配置
server:
port: 8090
#配置数据源
spring:
datasource:
#如果使用高版本驱动 则添加cj
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://127.0.0.1:3306/jt?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf8&autoReconnect=true&allowMultiQueries=true
username: root
password: root
#Spring整合MP
mybatis-plus:
#定义别名包
type-aliases-package: com.jt.pojo
#导入映射文件
mapper-locations: classpath:/mappers/*.xml
#开启驼峰映射
configuration:
map-underscore-to-camel-case: true
#打印SQL语句
logging:
level:
com.jt.mapper: debug
测试类
package com.jt;
import com.baomidou.mybatisplus.core.conditions.Wrapper;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.conditions.update.UpdateWrapper;
import com.jt.mapper.UserMapper;
import com.jt.pojo.User;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import java.util.*;
@SpringBootTest
public class TestMP {
@Autowired
private UserMapper userMapper;
/**
* 完成数据的入库操作
* 新增user数据(name="阿富",age=40,sex="厉害")
* Sql: insert into demo_user value(xx,xx,xx,xx)
* 思考: MP实现入库流程!!!
*/
//插入数据
@Test
public void test01() {
User user = new User();
user.setName("阿富").setAge(40).setSex("厉害");
//以对象的方式操作数据!!!
userMapper.insert(user);
System.out.println("入库操作成功!!!!");
}
//查询数据
@Test
public void test02(){
User user = new User();
List<User> users = userMapper.selectList(null);
System.out.println(users);
//根据id更新数据
// user.setId(231).setName("江流儿");
// userMapper.updateById(user);
}
@Test
public void test03() {
User user = new User();
UpdateWrapper<User> wrapper = new UpdateWrapper<>();
wrapper.eq("name", "阿富").set("name", "班纳");
int result = this.userMapper.update(null, wrapper);
}
@Test
public void select(){
User user = new User();
int id=231;
User user1 = userMapper.selectById(id);
System.out.println(user1);
System.out.println("--------------------------------");
Map<String, Object> columnMap = new HashMap<>();
columnMap.put("name","小乔");
columnMap.put("sex","女");
List<User> users = userMapper.selectByMap(columnMap);
System.out.println(users);
System.out.println("-------------------");
user.setName("小乔").setSex("女");
/**条件构造器,根据对象不为null的属性充当where条件*/
QueryWrapper<User> queryWrapper = new QueryWrapper(user);
//根据条件构造器,实现查询
List<User> users1 = userMapper.selectList(queryWrapper);
System.out.println("users1: "+users1);
System.out.println("00000000000000000000");
}
//逻辑运算符 == eq ,> gt ,< lt,>= ge ,<= le ,!= ne
/**
* 以mybatis的方式实现数据库查询
* 1.实现user入库操作 insert into
* 2.update 将name="阿富" 改为 name="班纳"
* 3.delete 将name="班纳"数据删除.
* 4.select 查询 name="小乔" 并且 性别 ="女"
* 5.select 查询age < 18岁 性别="女"
* 6.select 查询 name包含 '君'字的数据
* 7.select 查询 sex="女" 按照年龄倒序排列.
* 8.根据 name/sex 不为null的数据查询. 动态Sql!!
* name="xxx" sex=null
* name="xxx" sex="xx"
* name=null sex=null
*/
//1.查询ID查询数据库 id=231 主键查询
@Test
public void selectById(){
int id = 231; //模拟用户参数.
User user = userMapper.selectById(id);
System.out.println(user);
}
/**
* 2.查询 name="小乔" 并且 性别 ="女"
* 思路: 如果将来有多个结果 则使用List进行接收.
* Sql: select * from demo_user where name="小乔" and sex="女"
* 注意事项: 默认的连接符 and
*/
@Test
public void select01(){
//1.通过对象封装数据
User user = new User();
user.setName("小乔").setSex("女");
//2.构建条件构造器 根据对象中不为null的属性充当where条件!
QueryWrapper<User> queryWrapper = new QueryWrapper(user);
//3.根据条件构造器 实现数据查询
List<User> userList = userMapper.selectList(queryWrapper);
System.out.println(userList);
}
/**
* 查询 name="小乔" 并且 性别 ="女"
* 逻辑运算符: = eq, > gt, < lt
* >= ge, <= le
* != ne
*/
@Test
public void select02(){
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("name","小乔")
.eq("sex","女");
List<User> userList =
userMapper.selectList(queryWrapper);
System.out.println(userList);
}
/**
* 案例: select 查询age < 18岁 性别="女"
* 方式1: 利用mybatis方式实现
* 方式2: 利用MP方式实现
*/
@Test
public void select03(){
//1.mybatis写法
List<User> userList = userMapper.findList(18,"女");
System.out.println(userList);
//2.mp写法
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.lt("age",18)
.eq("sex","女");
List<User> userList2 = userMapper.selectList(queryWrapper);
System.out.println(userList2);
}
/**
* 6.select 查询 name包含 '君'字的数据
* 关键字: like "%xxx%"
* 以君开头: likeRight "君%"
* 以君结尾: likeLeft "%君"
*/
@Test
public void select04(){
QueryWrapper queryWrapper = new QueryWrapper();
queryWrapper.like("name","君");
List<User> userList = userMapper.selectList(queryWrapper);
System.out.println(userList);
}
/**
* 7.select 查询 sex="女" 按照年龄倒序排列.
*/
@Test
public void select05(){
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("sex","女")
.orderByDesc("age");
List<User> userList = userMapper.selectList(queryWrapper);
System.out.println(userList);
}
/**
* 需求: 动态Sql查询. 如果数据有值 则拼接where条件.
* 如果数据为null 则不拼接where条件
* 语法: condition: true 则拼接where条件
* false 不拼接where条件
*/
@Test
public void select06(){
String name = "小乔";
int age = 0;
boolean nameFlag = name == null ? false : true;
boolean ageFlag = age == 0 ? false : true;
QueryWrapper<User> queryWrapper = new QueryWrapper();
queryWrapper.eq(nameFlag,"name",name)
.eq(ageFlag,"age",age);
List<User> userList = userMapper.selectList(queryWrapper);
System.out.println(userList);
}
/**
* 批量查询
*/
@Test
public void select07(){
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.in("id", 1,2,3,4,5,6);
List<User> users = userMapper.selectList(queryWrapper);
for (User list: users) {
System.out.println(list);
}
Integer[] arry=new Integer[]{
1,2,3,4,5,6};
List<Integer> ids = Arrays.asList(arry);
List<User> users1 = userMapper.selectBatchIds(ids);
System.out.println(users1);
}
/*
* 9.查询性别为男的用户,只查询ID字段
* selectObjs(); 只查询第一列字段(主键)
* 实际用途: 根据业务只需要主键的查询
* */
@Test
public void selectObjs(){
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("sex", "男");
List<Object> ids = userMapper.selectObjs(queryWrapper);
System.out.println(ids);
}
//更新数据
@Test
public void update01(){
User user = new User();
user.setId(231).setName("周诺").setAge(25).setSex("男");
userMapper.updateById(user);
}
@Test
public void update02(){
User user = new User();
user.setName("班纳").setAge(40).setSex("911");//更新内容
UpdateWrapper<User> updateWrapper = new UpdateWrapper<>();
updateWrapper.eq("name", "阿富");
userMapper.update(user,updateWrapper);//条件
System.out.println("更新成功");
}
//删除数据
@Test
public void dete(){
User user = new User();
Map<String, Object> columnMap = new HashMap<>();
columnMap.put("name", "班纳");
Integer result = userMapper.deleteByMap(columnMap);
System.out.println("result: "+result);
}
}
总结知识点
1.
**MybatisPlus中的
逻辑运算符 == eq ,> gt ,< lt,>= ge ,<= le ,!= ne
万能转译符:<![ CDATA[
SQL语句
]>
**
*例:
<select id="findList" resultType="User">
<![CDATA[
select * from demo_user
where age < #{age}
and
sex = #{sex}
]]>
</select>
2.
**模糊查询
select 查询 name包含 '君’字的数据
* 关键字: like “%xxx%”
* 以君开头: likeRight “君%”
* 以君结尾: likeLeft “%君”
**
3
条件构造器: new QueryWrapper<>(); 动态拼接where条件.