订阅模型
希望发送的消息部分消费者消费,交换机与队列的绑定不能是任意绑定,而是指定一个routinkey,消息的发送方在向交换机发送消息时,必须指定消息的路由。
交换机不在把消息交给每一个绑定的队列,而是根据消息的routingkey进行判断,只能队列和消息的routingkey一致时,才会接收到消息。
In this setup, we can see the direct exchange X with two queues bound to it. The first queue is bound with binding key orange, and the second has two bindings, one with binding key black and the other one with green.
In such a setup a message published to the exchange with a routing key orange will be routed to queue Q1. Messages with a routing key of black or green will go to Q2. All other messages will be discarded.
相关代码
package com.baizhi.routing;
import com.baizhi.Utils.rabbitmqUtils;
import com.rabbitmq.client.Channel;
import com.rabbitmq.client.Connection;
import java.io.IOException;
public class Provider {
public static void main(String[] args) {
Connection connection= rabbitmqUtils.getConnection();
try {
Channel channel=connection.createChannel();
channel.exchangeDeclare("logs_direct", "direct");
//
String routingName="Info";
channel.basicPublish("logs_direct", routingName, null, ("这是routingkey为"+routingName+"发布的消息").getBytes());
rabbitmqUtils.closeChannlAndConnection(channel, connection);
} catch (IOException e) {
e.printStackTrace();
}
}
}
package com.baizhi.routing;
import com.baizhi.Utils.rabbitmqUtils;
import com.rabbitmq.client.*;
import java.io.IOException;
public class Customer1 {
public static void main(String[] args) {
Connection connection= rabbitmqUtils.getConnection();
Channel channel= null;
try {
channel = connection.createChannel();
channel.exchangeDeclare("logs_direct", "direct");
String queueName=channel.queueDeclare().getQueue();
channel.queueBind(queueName, "logs_direct", "error");
channel.basicConsume(queueName, true, new DefaultConsumer(channel){
@Override
public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException {
System.out.println("消费者1"+new String(body));
}
});
} catch (IOException e) {
e.printStackTrace();
}
}
}
package com.baizhi.routing;
import com.baizhi.Utils.rabbitmqUtils;
import com.rabbitmq.client.*;
import java.io.IOException;
public class Customer2 {
public static void main(String[] args) {
Connection connection= rabbitmqUtils.getConnection();
Channel channel= null;
try {
channel = connection.createChannel();
channel.exchangeDeclare("logs_direct", "direct");
String queueName=channel.queueDeclare().getQueue();
//通道可以绑定多个路由
channel.queueBind(queueName, "logs_direct", "Info");
channel.queueBind(queueName, "logs_direct", "error");
channel.queueBind(queueName, "logs_direct", "warning");
channel.basicConsume(queueName, true, new DefaultConsumer(channel){
@Override
public void handleDelivery(String consumerTag, Envelope envelope, AMQP.BasicProperties properties, byte[] body) throws IOException {
System.out.println("消费者2"+new String(body));
}
});
} catch (IOException e) {
e.printStackTrace();
}
}
}
测试代码
provider提供Info信息,理论上消费者1无法接受,因为绑定的routingkey是error,消费者2可以正常接收。
扫描二维码关注公众号,回复:
13141822 查看本文章
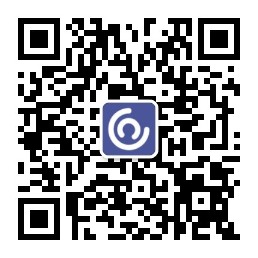
provider提供error信息,消费者1,2都能接收。