基础准备
先人于网络对List - SortedList - Dictionary - SortedDictionary述之可谓备矣,然着墨点至高,如下是我对它们的实践。
首先,建一个工程项目,有个Form1,在Form1上放个Button1。然后,写个简单的类 Teacher
public class Teacher
{
private readonly string name = "";
private readonly string sex = "";
private readonly int age = 20;
private readonly string subject = "";
public Teacher(string Name, string Sex, int Age, string Subject)
{
name = Name;
sex = Sex;
age = Age;
subject = Subject;
}
public string Name { get { return name;}}
public int Age { get { return age; }}
public string Sex { get { return sex; }}
public string Subject { get { return subject; }}
}
类中有几个私有变量,一个构造函数,和几个带属性查询的公有变量。教师类 (string姓名, string性别, int年龄, string学科)
在Button下操练
private void Button1_Click(object sender, EventArgs e)
{
List<Teacher> list = new List<Teacher>();
SortedList sortedlist = new SortedList();
Dictionary<string, Teacher> dictionary = new Dictionary<string, Teacher>();
SortedDictionary<string, Teacher> sorteddictionary = new SortedDictionary<string, Teacher>();
//如上所写,定义出List - SortedList - Dictionary - SortedDictionary, 将张1张2张3张4加入到List - SortedList - Dictionary - SortedDictionary中去如下:
Teacher teacher;
teacher = new Teacher("张1", "男", 21, "数学"); list.Add(teacher); sortedlist.Add(teacher.Name, teacher); dictionary.Add(teacher.Name, teacher); sorteddictionary.Add(teacher.Name, teacher);
teacher = new Teacher("张2", "男", 22, "语言"); list.Add(teacher); sortedlist.Add(teacher.Name, teacher); dictionary.Add(teacher.Name, teacher); sorteddictionary.Add(teacher.Name, teacher);
teacher = new Teacher("张3", "男", 23, "物理"); list.Add(teacher); sortedlist.Add(teacher.Name, teacher); dictionary.Add(teacher.Name, teacher); sorteddictionary.Add(teacher.Name, teacher);
teacher = new Teacher("张4", "男", 24, "化学"); list.Add(teacher); sortedlist.Add(teacher.Name, teacher); dictionary.Add(teacher.Name, teacher); sorteddictionary.Add(teacher.Name, teacher);
//List成员比较简单,成员Teacher类的4个实例,读出它们也比较容易,直接用数组作为teacher实例带上它们的属性
//Using List
Console.WriteLine(list[0].Name + list[0].Sex + list[0].Age.ToString() + list[0].Subject); //teacher 1 in LIST
Console.WriteLine(list[1].Name + list[1].Sex + list[1].Age.ToString() + list[1].Subject); //teacher 2 in LIST
Console.WriteLine(list[2].Name + list[2].Sex + list[2].Age.ToString() + list[2].Subject); //teacher 3 in LIST
Console.WriteLine(list[3].Name + list[3].Sex + list[3].Age.ToString() + list[3].Subject); //teacher 4 in LIST
//SortedList - Dictionary - SortedDictionary较List复杂一点,两了个参数。一个是string,一个是类,用GetKey(索引)即可读出所存的值并ToString转换
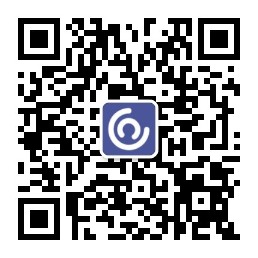
//Using SortedList
Console.WriteLine(sortedlist.GetKey(0).ToString() + sortedlist.GetKey(1).ToString() + sortedlist.GetKey(2).ToString() + sortedlist.GetKey(3).ToString()); //teacher.names in SORTEDLIST
//SortedList键值对中的Value值是类实例,用GetByIndex(索引)将其读出并转换成Teacher类。VB.NET里用CType(变量,类型j)函数,不如C#如理解。
teacher = (Teacher)sortedlist.GetByIndex(0); Console.WriteLine(teacher.Name + teacher.Sex + teacher.Age.ToString() + teacher.Subject); //teacher 1 in SORTEDLIST
teacher = (Teacher)sortedlist.GetByIndex(1); Console.WriteLine(teacher.Name + teacher.Sex + teacher.Age.ToString() + teacher.Subject); //teacher 2 in SORTEDLIST
teacher = (Teacher)sortedlist.GetByIndex(2); Console.WriteLine(teacher.Name + teacher.Sex + teacher.Age.ToString() + teacher.Subject); //teacher 3 in SORTEDLIST
teacher = (Teacher)sortedlist.GetByIndex(3); Console.WriteLine(teacher.Name + teacher.Sex + teacher.Age.ToString() + teacher.Subject); //teacher 4 in SORTEDLIST
//查找并显示
Console.WriteLine(sortedlist.IndexOfKey("张3")); //查找张3所在的索引值
teacher = (Teacher)sortedlist.GetByIndex(sortedlist.IndexOfKey("张3")); Console.WriteLine("teacherFound " + teacher.Name + teacher.Sex + teacher.Age.ToString() + teacher.Subject);
//Dictionary和SortedDictionary不同于List和SortedList,用它们的Keys和Values属性将其转成数组后应该差不多的。
//Using Dictionary2Array
string[] dicKeys = dictionary.Keys.ToArray<string>();
string stringdickeys = "";
for (int i = 0; i<= 3; i++)
{
stringdickeys += dicKeys[i];
}
Console.WriteLine(stringdickeys);
Teacher[] dicVals = dictionary.Values.ToArray<Teacher>();
for (int i = 0; i <= 3; i++)
{
Console.WriteLine("Dictionary " + dicVals[i].Name + dicVals[i].Sex + dicVals[i].Age.ToString() + dicVals[i].Subject);
}
//Using SortedDictionary2Array
string[] sorteddicKeys = sorteddictionary.Keys.ToArray<string>();
stringdickeys = "";
for (int i = 0; i <= 3; i++)
{
stringdickeys += sorteddicKeys[i];
}
Console.WriteLine(stringdickeys);
Teacher[] sorteddicVals = sorteddictionary.Values.ToArray<Teacher>();
for (int i = 0; i <= 3; i++)
{
Console.WriteLine("SortedDictionary " + sorteddicVals[i].Name + sorteddicVals[i].Sex + sorteddicVals[i].Age.ToString() + sorteddicVals[i].Subject);
}
//对Dictionary - SortedDictionary的Keys和Values直接遍历
//Using Dictionary
stringdickeys = "";
foreach (string key in dictionary.Keys)
{
stringdickeys += key;
}
Console.WriteLine(stringdickeys);
foreach (Teacher value in dictionary.Values)
{
Console.WriteLine(value.Name + value.Sex + value.Age.ToString() + value.Subject);
}
//Using SortedDictionary
stringdickeys = "";
foreach (string key in sorteddictionary.Keys)
{
stringdickeys += key;
}
Console.WriteLine(stringdickeys);
foreach (Teacher value in sorteddictionary.Values)
{
Console.WriteLine(value.Name + value.Sex + value.Age.ToString() + value.Subject);
}
}
注意事项
List - SortedList归根结底是List,可以有重值的。Dictionary - SortedDictionary是字典,字典查询是不重值的。比如电话号码本,以电话号为Key应该不会有重的,但以人名为Key会有重值。List - SortedList - Dictionary - SortedDictionary对数据存储查询插入删除等操作用时不同的,效率有差异但也各有所长。如果程序对效率要求不是Critical,那随便用哪个适用就行; 如果程序要求效率Critical,到网上查询一下先人们的测试结果,选效率好的适合要求的那个。感觉对它们的操练还缺点什么,继续努力吧。