我们平日采用spring 框架 开发项目时,配置文件web.xml 中总是有这样一些配置,比如:
<!-- 加载spring配置文件--> <context-param> <param-name>contextConfigLocation</param-name> <param-value> classpath:config/spring-config.xml classpath:config/spring-cxf.xml </param-value> </context-param>
<!-- web启动监听配置-->
<listener> <listener-class> org.springframework.web.context.ContextLoaderListener </listener-class> </listener>
而这些配置到底有什么作用呢?我们从源码角度分析一下:
1、首先看ContextLoaderListener 类,它继承ContextLoader 实现 ServletContextListener
public class ContextLoaderListener extends ContextLoader implements ServletContextListener
ContextLoaderListener的作用就是启动Web容器时,读取在contextConfigLocation中定义的xml文件,自动装配ApplicationContext的配置信息,并产生WebApplicationContext对象,然后将这个对象放置在ServletContext的属性里,这样我们只要得到Servlet就可以得到WebApplicationContext对象,并利用这个对象访问spring容器管理的bean。
总之,就是上面这段配置为项目提供了spring支持,初始化了Ioc容器。
ContextLoader 主要作用作为父类指定Web应用程序启动时载入相应的Ioc容器,完成实际的WebApplicationContext,即Ioc容器的初始化工作在这里完成,后面会源码展示。
ServletContextListener 接口继承EventListener
public interface ServletContextListener extends EventListener
ServletContextListener是ServletContext的监听者,接口里的方法会结合Web容器的生命周期被调用,ServletContext发生某些变化,会触发相应的事件,web服务器启动时、ServletContext创建时、web服务关闭时、ServletContext销毁时等等,都会触发相应事件,做出预先设计好的相应动作,比如此类中:监听器的响应动作就是在服务器启动时contextInitialized会被调用,关闭的时候contextDestroyed被调用。
/** * Initialize the root web application context. 启动时在这里开始调用,初始化根上下文应用程序 */ @Override public void contextInitialized(ServletContextEvent event) { initWebApplicationContext(event.getServletContext());//点击进入 }
2、进入 ContextLoader 类
public WebApplicationContext initWebApplicationContext(ServletContext servletContext) { //如果servletContext对象中存放了spring context,则抛出异常 if (servletContext.getAttribute(WebApplicationContext.ROOT_WEB_APPLICATION_CONTEXT_ATTRIBUTE) != null) { throw new IllegalStateException( "Cannot initialize context because there is already a root application context present - " + "check whether you have multiple ContextLoader* definitions in your web.xml!"); } servletContext.log("Initializing Spring root WebApplicationContext"); Log logger = LogFactory.getLog(ContextLoader.class); if (logger.isInfoEnabled()) { logger.info("Root WebApplicationContext: initialization started"); } long startTime = System.currentTimeMillis(); try { // Store context in local instance variable, to guarantee that // it is available on ServletContext shutdown. if (this.context == null) { //创建WebApplicationContext容器并被强制转换为ConfigurableWebApplicationContext类型 this.context = createWebApplicationContext(servletContext); } if (this.context instanceof ConfigurableWebApplicationContext) { ConfigurableWebApplicationContext cwac = (ConfigurableWebApplicationContext) this.context; if (!cwac.isActive()) { // The context has not yet been refreshed -> provide services such as // setting the parent context, setting the application context id, etc if (cwac.getParent() == null) { // The context instance was injected without an explicit parent -> // determine parent for root web application context, if any. //加载父容器 ApplicationContext parent = loadParentContext(servletContext); cwac.setParent(parent); } //加载spring配置文件,点击进入 configureAndRefreshWebApplicationContext(cwac, servletContext); } }//把web容器放到servlet容器中 servletContext.setAttribute(WebApplicationContext.ROOT_WEB_APPLICATION_CONTEXT_ATTRIBUTE, this.context); ClassLoader ccl = Thread.currentThread().getContextClassLoader(); if (ccl == ContextLoader.class.getClassLoader()) { currentContext = this.context; } else if (ccl != null) { currentContextPerThread.put(ccl, this.context); } if (logger.isInfoEnabled()) { long elapsedTime = System.currentTimeMillis() - startTime; logger.info("Root WebApplicationContext initialized in " + elapsedTime + " ms"); } return this.context; } catch (RuntimeException | Error ex) { logger.error("Context initialization failed", ex); servletContext.setAttribute(WebApplicationContext.ROOT_WEB_APPLICATION_CONTEXT_ATTRIBUTE, ex); throw ex; } }
来到configureAndRefreshWebApplicationContext方法中
protected void configureAndRefreshWebApplicationContext(ConfigurableWebApplicationContext wac, ServletContext sc) { if (ObjectUtils.identityToString(wac).equals(wac.getId())) { // The application context id is still set to its original default value // -> assign a more useful id based on available information //获取 web.xml 中定义的 contextId 初始化值,未定义 String idParam = sc.getInitParameter(CONTEXT_ID_PARAM); if (idParam != null) { wac.setId(idParam); } else { // Generate default id... 设置一个 全局的id wac.setId(ConfigurableWebApplicationContext.APPLICATION_CONTEXT_ID_PREFIX + ObjectUtils.getDisplayString(sc.getContextPath())); } } wac.setServletContext(sc);//配置了 servletContext 属性 //获取 web.xml 中定义的 contextConfiLocation 初始化参数值 ,已定义 String configLocationParam = sc.getInitParameter(CONFIG_LOCATION_PARAM); if (configLocationParam != null) { wac.setConfigLocation(configLocationParam); } // The wac environment's #initPropertySources will be called in any case when the context // is refreshed; do it eagerly here to ensure servlet property sources are in place for // use in any post-processing or initialization that occurs below prior to #refresh //初始化 应用上下文 中 和 Servlet 相关的 占位符 属性资源 ConfigurableEnvironment env = wac.getEnvironment(); if (env instanceof ConfigurableWebEnvironment) { ((ConfigurableWebEnvironment) env).initPropertySources(sc, null); } /// 加载web.xml 中 自定义的 其他初始化内容 customizeContext(sc, wac); wac.refresh();//WebApplicationContext 的刷新,核心方法,点击进入后豁然开朗,ctr+t 进入 }
3、进入这个方法,非常熟悉,前面几篇已经详细解析过,是spring 源码的重点方法,这里就不介绍了。
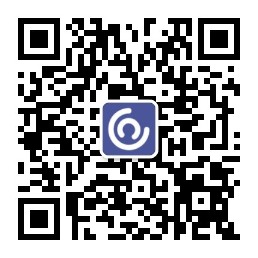
public abstract class AbstractApplicationContext extends DefaultResourceLoader implements ConfigurableApplicationContext {
@Override public void refresh() throws BeansException, IllegalStateException { synchronized (this.startupShutdownMonitor) { // Prepare this context for refreshing. prepareRefresh(); // Tell the subclass to refresh the internal bean factory. ConfigurableListableBeanFactory beanFactory = obtainFreshBeanFactory(); // Prepare the bean factory for use in this context. prepareBeanFactory(beanFactory); try { // Allows post-processing of the bean factory in context subclasses. postProcessBeanFactory(beanFactory); // Invoke factory processors registered as beans in the context. invokeBeanFactoryPostProcessors(beanFactory); // Register bean processors that intercept bean creation. registerBeanPostProcessors(beanFactory); // Initialize message source for this context. initMessageSource(); // Initialize event multicaster for this context. initApplicationEventMulticaster(); // Initialize other special beans in specific context subclasses. onRefresh(); // Check for listener beans and register them. registerListeners(); // Instantiate all remaining (non-lazy-init) singletons. finishBeanFactoryInitialization(beanFactory); // Last step: publish corresponding event. finishRefresh(); } catch (BeansException ex) { if (logger.isWarnEnabled()) { logger.warn("Exception encountered during context initialization - " + "cancelling refresh attempt: " + ex); } // Destroy already created singletons to avoid dangling resources. destroyBeans(); // Reset 'active' flag. cancelRefresh(ex); // Propagate exception to caller. throw ex; } finally { // Reset common introspection caches in Spring's core, since we // might not ever need metadata for singleton beans anymore... resetCommonCaches(); } } }
4、重点这是spring 的容器初始化,下篇介绍springMVC 容器初始化;他俩是父子容器关系。敬请期待!