Description
A robot on an infinite XY-plane starts at point (0, 0) and faces north. The robot can receive one of three possible types of commands:
- -2: turn left 90 degrees,
- -1: turn right 90 degrees, or
- 1 <= k <= 9: move forward k units.
Some of the grid squares are obstacles. The ith obstacle is at grid point obstacles[i] = (xi, yi).
If the robot would try to move onto them, the robot stays on the previous grid square instead (but still continues following the rest of the route.)
Return the maximum Euclidean distance that the robot will be from the origin squared (i.e. if the distance is 5, return 25).
Note:
- North means +Y direction.
- East means +X direction.
- South means -Y direction.
- West means -X direction.
Example 1:
Input: commands = [4,-1,3], obstacles = []
Output: 25
Explanation: The robot starts at (0, 0):
1. Move north 4 units to (0, 4).
2. Turn right.
3. Move east 3 units to (3, 4).
The furthest point away from the origin is (3, 4), which is 32 + 42 = 25 units away.
Example 2:
Input: commands = [4,-1,4,-2,4], obstacles = [[2,4]]
Output: 65
Explanation: The robot starts at (0, 0):
1. Move north 4 units to (0, 4).
2. Turn right.
3. Move east 1 unit and get blocked by the obstacle at (2, 4), robot is at (1, 4).
4. Turn left.
5. Move north 4 units to (1, 8).
The furthest point away from the origin is (1, 8), which is 1^2 + 8^2 = 65 units away.
Constraints:
- 1 <= commands.length <= 104
- commands[i] is one of the values in the list [-2,-1,1,2,3,4,5,6,7,8,9].
- 0 <= obstacles.length <= 104
- -3 * 104 <= xi, yi <= 3 * 104
- The answer is guaranteed to be less than 2^31.
分析
题目的意思是:一个机器人,站在(0,0)位置,现在给定一些commands命令,-2 表示左转 90 度,-1 表示右转 90 度,正数表示沿当前方向前进该正数步,然后给了一个障碍数组obstacles,就是机器人无法通过的地方,比如当前机器人面前有个障碍物,此时机器人接到的指令是前进x步,但是由于障碍物的因素,该指令无法执行,机器人呆在原地不动。
思路很直接,把机器人的命令模拟出来,就知道最终到达的地方了,注意接收命令-2和-1的时候,转弯的代码的处理,-2是向左,则
di=(di-1)%4
-1是向右
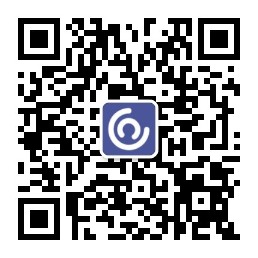
di=(di+1)%4
可以对照dirs=[[0,1],[1,0],[0,-1],[-1,0]] 模拟一下,看是不是这样的。[0,1]变成[1,0]是向右,[1,0]变成[0,1]则是向左了,跟上面公式是一致的。
- 如果是cmd不是命令,而是机器人所要走的步数,则要判断到达的地方是否在obstacles,在的话,则不能到达,否则就可以到达,更新坐标和res。注意cmd的步数是通过循环一步一步的判断的,不能直接加入cmd步数哈,可能会跳过obstacles坐标。
有兴趣的话,可以自己调试,调试代码见代码二
代码
class Solution:
def robotSim(self, commands: List[int], obstacles: List[List[int]]) -> int:
dirs=[[0,1],[1,0],[0,-1],[-1,0]]
x=0
y=0
di=0
obstacleSet=set(map(tuple,obstacles))
res=0
for cmd in commands:
if(cmd==-2):
di=(di-1)%4
elif(cmd==-1):
di=(di+1)%4
else:
for k in range(cmd):
x1=x+dirs[di][0]
y1=y+dirs[di][1]
if((x1,y1) not in obstacleSet):
x=x1
y=y1
res=max(res,x*x+y*y)
return res
代码二
class Solution:
def robotSim(self, commands, obstacles) -> int:
dirs=[[0,1],[1,0],[0,-1],[-1,0]]
x=0
y=0
di=0
obstacleSet=set(map(tuple,obstacles))
res=0
print(obstacleSet)
for cmd in commands:
if(cmd==-2):
di=(di-1)%4
elif(cmd==-1):
di=(di+1)%4
else:
for k in range(cmd):
x1=x+dirs[di][0]
y1=y+dirs[di][1]
if((x1,y1) not in obstacleSet):
x=x1
y=y1
res=max(res,x*x+y*y)
return res
if __name__=="__main__":
commands = [4,-1,4,-2,4]
obstacles = [[2,4]]
solution=Solution()
res=solution.robotSim(commands,obstacles)
print(res)