新建项目
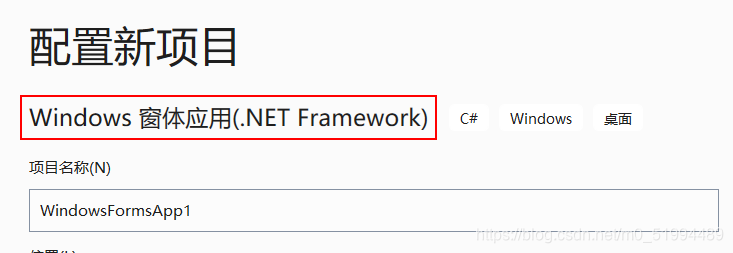
实体类
namespace WindowsFormsApp3
{
public class User
{
public int id {
get; set; }
public string username {
get; set; }
public string pwd {
get; set; }
public int auth {
get; set; }
public User() {
}
public User(int id, string username, string pwd, int auth)
{
this.id = id;
this.username = username;
this.pwd = pwd;
this.auth = auth;
}
}
}
数据库连接 工具类
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Data.SqlClient;
namespace WindowsFormsApp3
{
class SqlUtils
{
static string connStr = @"server=LAPTOP-H9JFQJGF\SQLEXPRESS;uid=sa;pwd=123456;database=warehouse";
static SqlConnection conn = null;
public static SqlCommand ConnectSql(string sql)
{
try
{
conn = new SqlConnection(connStr);
conn.Open();
return new SqlCommand(sql, conn);
}
catch (Exception ex)
{
Console.WriteLine(ex);
}
return null;
}
public static void CloseSql(SqlDataReader sdr)
{
if (sdr != null)
{
sdr.Close();
}
conn.Close();
}
}
}
POST/GET 工具类
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Net;
using System.IO;
namespace WindowsFormsApp3
{
class HttpUtils
{
public static string Get(string Url)
{
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(Url);
request.Proxy = null;
request.KeepAlive = false;
request.Method = "GET";
request.ContentType = "application/json; charset=UTF-8";
request.AutomaticDecompression = DecompressionMethods.GZip;
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
Stream myResponseStream = response.GetResponseStream();
StreamReader myStreamReader = new StreamReader(myResponseStream, Encoding.UTF8);
string retString = myStreamReader.ReadToEnd();
myStreamReader.Close();
myResponseStream.Close();
if (response != null)
{
response.Close();
}
if (request != null)
{
request.Abort();
}
return retString;
}
public static string Post(string Url, string Data,string Referer)
{
HttpWebRequest request = (HttpWebRequest)WebRequest.Create(Url);
request.Method = "POST";
request.Referer = Referer;
byte[] bytes = Encoding.UTF8.GetBytes(Data);
request.ContentType = "application / x - www - form - urlencoded";
request.ContentLength = bytes.Length;
Stream myResponseStream = request.GetRequestStream();
myResponseStream.Write(bytes,0,bytes.Length);
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
StreamReader myStreamReader = new StreamReader(response.GetResponseStream(), Encoding.UTF8);
string retString = myStreamReader.ReadToEnd();
myStreamReader.Close();
myResponseStream.Close();
if (response != null) {
response.Close();
}
if (request != null) {
request.Abort();
}
return retString;
}
}
}
App.config配置文件
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<configSections>
<sectionGroup name="applicationSettings" type="System.Configuration.ApplicationSettingsGroup, System, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" >
<section name="WindowsFormsApp3.Properties.Settings" type="System.Configuration.ClientSettingsSection, System, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" requirePermission="false" />
</sectionGroup>
</configSections>
<connectionStrings>
<add name="WindowsFormsApp3.Properties.Settings.warehouseConnectionString"
connectionString="Data Source=LAPTOP-H9JFQJGF\SQLEXPRESS;Initial Catalog=warehouse;Integrated Security=True"
providerName="System.Data.SqlClient" />
</connectionStrings>
<startup>
<supportedRuntime version="v4.0" sku=".NETFramework,Version=v4.5" />
</startup>
<applicationSettings>
<WindowsFormsApp3.Properties.Settings>
<setting name="WindowsFormsApp3_localhost_WebServiceTest01" serializeAs="String">
<value>https://localhost:44365/WebServiceTest01.asmx</value>
</setting>
</WindowsFormsApp3.Properties.Settings>
</applicationSettings>
<system.serviceModel>
<bindings>
<basicHttpBinding>
<binding name="WebServiceTest01Soap">
<security mode="Transport" />
</binding>
<binding name="WebServiceTest01Soap1" />
</basicHttpBinding>
</bindings>
<client>
<endpoint address="https://localhost:44365/WebServiceTest01.asmx"
binding="basicHttpBinding" bindingConfiguration="WebServiceTest01Soap"
contract="AddService.WebServiceTest01Soap" name="WebServiceTest01Soap" />
</client>
</system.serviceModel>
<system.webServer>
<httpProtocol>
<customHeaders>
<add name="Access-Control-Allow-Origin" value="*" />
<add name="Access-Control-Allow-Headers" value="Content-Type" />
<add name="Access-Control-Allow-Methods" value="GET, POST, PUT, DELETE, OPTIONS" />
</customHeaders>
</httpProtocol>
</system.webServer>
</configuration>
窗体
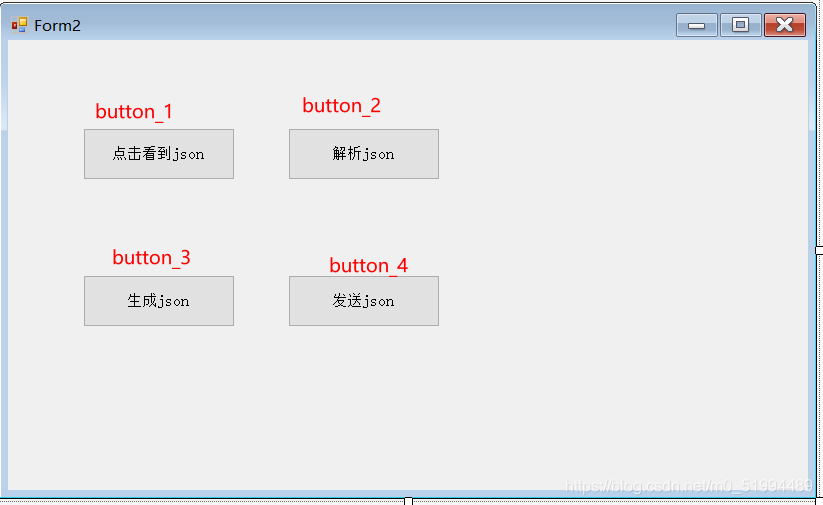
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Data.SqlClient;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp3
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
string url = "http://www.kuaidi100.com/query?type=shunfeng&postid=367847964498";
string getJson = HttpUtils.Get(url);
MessageBox.Show(getJson);
}
private void button2_Click(object sender, EventArgs e)
{
string url = "http://www.kuaidi100.com/query?type=shunfeng&postid=367847964498";
string getJson = HttpUtils.Get(url);
Root rt = JsonConvert.DeserializeObject<Root>(getJson);
MessageBox.Show("com="+rt.com+"\r\n"+"condition="+rt.condition+"\r\n"+"ischeck="+rt.ischeck+"\r\n"+
"state="+rt.state+"\r\n"+"status="+rt.status);
for (int i = 0; i < rt.data.Count; i++) {
MessageBox.Show("Data="+rt.data[i].context+"\r\n"+rt.data[i].location+"\r\n"+rt.data[i].time+"\r\n"+rt.data[i].ftime);
}
}
private void button3_Click(object sender, EventArgs e)
{
string json = JsonConvert.SerializeObject(UserList());
MessageBox.Show(json);
}
private void button4_Click(object sender, EventArgs e)
{
HttpUtils.Post("http://localhost:8081/user",JsonConvert.SerializeObject(UserList()), "Access - Control - Allow - Origin");
MessageBox.Show("ok");
}
private List<User> UserList()
{
List<User> userList = new List<User>();
User user = null;
string sql = "select * from user_table";
SqlCommand cmd = SqlUtils.ConnectSql(sql);
SqlDataReader sdr = cmd.ExecuteReader();
while (sdr.Read())
{
user = new User(sdr.GetInt32(0), sdr.GetString(1), sdr.GetString(2), sdr.GetInt32(3));
userList.Add(user);
}
return userList;
}
}
}
测试效果
button_3 将User对象转换为json格式
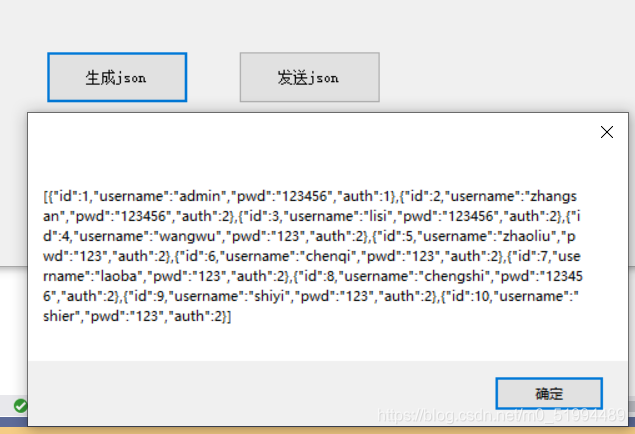
button_4 将json数据发送到服务器 报错
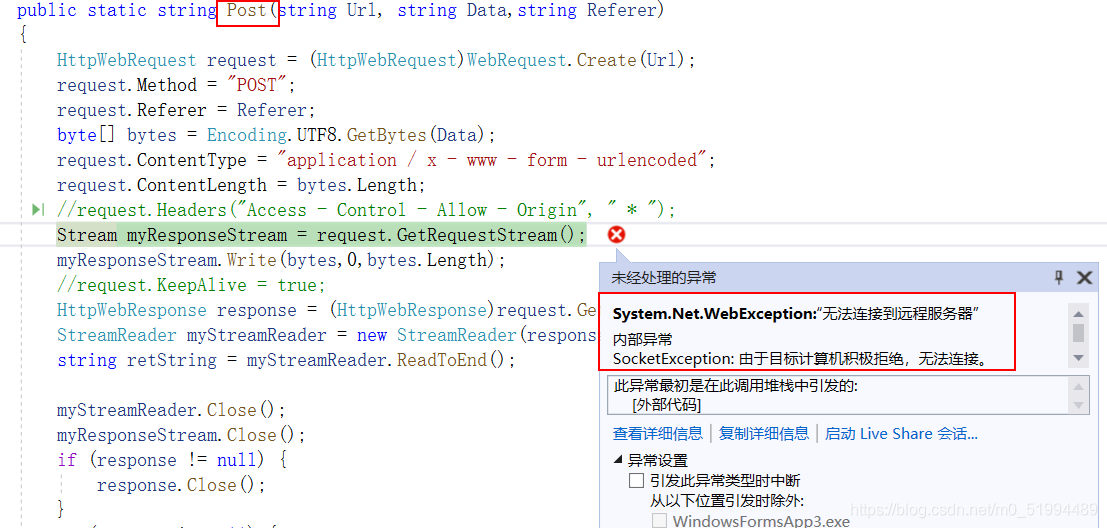