今天主要梳理一下Python中有关File的基本操作,通过实例来验证有关File各方法的功能,对文件操作的各种模式,异常处理,最后还涉及到有关format()的基本应用。
File IO Summary
Function | Interpretation | Example |
---|---|---|
open() | opens file access | f=open(“myfile.txt”) |
close() | closes file access | f.close() |
read() | reads the entire content of the file into a string | f.read() |
readline() | reads one line from a file, advances iterator to the next | f.readline() |
readlines() | reads all lines from a file into a list with each line as a list ite | f.readlines() |
write() | writes data to a file | f.write(data) |
writelines() | writes a list of strings to a file, each item on a separate line | f.writelines(list_of_lines) |
with open() | creates a context manager for a file | with open(“myfile”) as f: do something with f |
open() function
open(name[, mode[, encoding, buffering]])
参数 | 描述 |
---|---|
name | 文件路径 |
mode | 操作文件模式 |
encoding | 编码方式 |
buffering | 寄存状态,buffering设为0,访问不寄存,为1访问文件寄存,如果为大于1的整数,表明寄存区的缓冲大小 |
编码格式:
windows一般默认txt为ANSI编码格式,出现乱码一般都是原文件的编码方式和打开指定的编码不一致所致。可以用国际兼容的编码方式UTF-8,中文也支持。
操作模式 | 描述 |
---|---|
r | 只读模式打开文件,文件的指针在文件开头,即从第一行第一列读取文件内容 |
rb | 在二进制格式下以只读模式打开文件,,文件指针放在开头,即从第一行第一列读取文件内容 |
r+ | 读写模式打开文件,文件指针在开头 |
rb+ | 在二进制格式下以读写模式打开文件,文件指针在文件开头 |
w | 以写模式打开文件,文件指针在文件开头,即从文件头开始编辑,原有内容被删除,如果文件不存在,会自动创建文件 |
wb | 在二进制格式下以写模式打开文件,如果文件存在,从头开始编辑,原有内容被删除,文件不存在,自动创建文件 |
w+ | 读写模式打开文件,文件存在,从头开始编辑,原有内容被删除,文件不存在,自动创建文件 |
wb+ | 在二进制格式下以读写模式打开文件,从头开始编辑,原有内容被删除,文件不存在则自动创建文件 |
a | 打开文件追加内容,如果文件存在,文件指针放在文件结尾,即继续先前的文件继续编辑,文件不存在,自动创建文件 |
ab | 在二进制格式下追加文件内容,文件指针放在文件结尾,即继续先前的文件继续编辑,文件不存在,自动创建文件 |
a+ | 以读写模式追加文件内容,文件指针在文件结尾,即继续先前的文件继续编辑,文件不存在,自动创建文件 |
ab+ | 在二进制格式下追加文件内容,文件指针在文件结尾,即继续先前的文件继续编辑,文件不存在,自动创建文件 |
注意:后面有带b的方式,不需要考虑编码方式。有带+号的,则可读可写,不过它们之间还是有区别的
下面通过几个典型的例子说明各操作模式的差别:
text.txt content:
line1
line2
last line
r模式:原样输出
f = open(r'c:\Users\xiakx\Desktop\test.txt', 'r')
print(f.read())
# output:
line1
line2
last line
rb模式:一行输出,但是有字节格式b开头和换行标识符
f = open(r'c:\Users\xiakx\Desktop\test.txt', 'rb')
print(f.read())
#output:
b'line1\r\nline2\r\nlast line'
w模式:覆盖原来的内容
f = open(r'c:\Users\xiakx\Desktop\test.txt', 'w')
f.write('new line')
f.close()
# result:
new line
a模式:紧接着最后一个字符追加写入新的内容
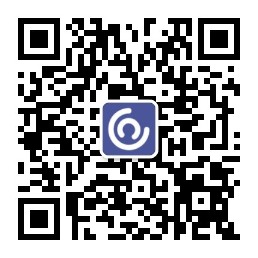
f = open(r'c:\Users\xiakx\Desktop\test.txt', 'a')
f.write('new line')
f.close()
# result:
line1
line2
last linenew line
r+模式:有点出乎意料了,其实文件内空已经变成了
new lineine2
last line
但是read输出只是一部分呢。这是因为光标的变化引起的,进行write的时候,光标在开头,会覆盖原有的内容,写完光标会停在写完的最后一个字符上,接着就read操作,所以光标前面的字符不会读到。
f = open(r'c:\Users\xiakx\Desktop\test.txt', 'r+')
f.write('new line')
print(f.read())
f.close()
#output:
ine2
last line
w+模式:写之前,写之后,read输出都为空了。其实最后文件内容是:
new line
只能说明open时就已经覆盖了原来的内容,后面写入新内容,但是此时光标是停在最后一个字符后面的,接着进行读,光标后面就不有内容了。
f = open(r'c:\Users\xiakx\Desktop\test.txt', 'w+')
print(f'read: {f.read()}')
f.write('new line')
print(f'read: {f.read()}')
f.close()
# output:
read:
read:
wb模式:原来的文件内容被覆盖了,新内容会根据换行符换行,所以现在文件内容是:
new line1
new line2
f = open(r'c:\Users\xiakx\Desktop\test.txt', 'wb')
f.write(b'new line1\nnew line2')
f.close()
下面是举例看看各function
ANSI编码格式test.txt content:
line1
line2
第三行
last line
readlines() reads all lines in the file,puts them in a list
and keeps new line character \n
f = open(r'c:\Users\xiakx\Desktop\test.txt', encoding = 'ansi')
list_lines = f.readlines()
print(f'readlines():{list_lines}')
for line in list_lines:
print(line)
f.close()
数组里的每个item的\n换行符也输出了
# output:
readlines():['line1\n', 'line2\n', '第三行\n', 'last line']
line1
line2
第三行
last line
readline():一行接着一行读,而且每行都会带\n换行符
另外Reads n characters for the line (readline(n))
f = open(r'c:\Users\xiakx\Desktop\test.txt', encoding = 'ansi')
line1 = f.readline()
line2 = f.readline()
line3 = f.readline()
line4 = f.readline()
print(line1)
print(line2)
print(line3)
print(line4)
f.close()
#output:
line1
line2
第三行
last line
writelines(): Writing ALL lines to a file
一个新的文件test_copy.txt生成,而且内容和text.txt是一样的:
line1
line2
第三行
last line
f = open(r'c:\Users\xiakx\Desktop\test.txt', encoding = 'ansi')
readlines = f.readlines()
f = open(r'c:\Users\xiakx\Desktop\test_copy.txt', 'w')
f.writelines(readlines)
f.close()
File I/O exception handling
如果文件存在将按行逐行输出,否则抛出异常。
try:
f = open('test.txt', encoding = 'ansi')
for data in f:
print(data)
except IOError as e:
print(e)
finally:
if f:
f.close()
# output:
[Errno 2] No such file or directory: 'test.txt'
还有一种嵌套异常处理, 文件不存在,open()失败,也就不用call close(), 因为文件是None,但是如果读的时候出现异常,不管怎样一定要执行close()
try:
f = open('test.txt', encoding = 'ansi')
try:
for data in f:
print(data)
finally:
f.close()
except IOError as e:
print(e)
with open():以上操作我们都要f.close(),我们读取文件是把文科读取到内存中的,如果没关闭它,它就会一直占用系统资源,而且还可能导致其他不安全隐患。还有一种方法让我们不用特意关注关闭文件。那就是 with open(),无论在文件使用中遇到什么问题都能安全的退出,即使发生错误,退出运行时环境时也能安全退出文件并给出报错信息。
try:
with open(r'c:\Users\xiakx\Desktop\test.txt', encoding = 'ansi') as fin,\
open(r'c:\Users\xiakx\Desktop\test_copy.txt', 'w') as fout:
for line in fin:
fout.write(line)
except IOError as e:
print(e)
Strings: format():
# positional arguments
my_str = 'I am {0} student of Python {1} course.'
new_str = my_str.format('John Brown', 200)
# keyword arguments
my_str2 = 'I am {name} student of Python {level} course.'
new_str2 = my_str2.format(name='John Brown', level=200)
print ('new_str: ' + new_str)
print ('new_str2: ' +new_str2)
# output:
new_str: I am John Brown student of Python 200 course.
new_str2: I am John Brown student of Python 200 course.
字符截断:
.n—>截取字符个数
:n—>宽度,不足空格填充
s1 = 'Timothy'
# truncating strings to 3 letters
print('{:.3}'.format(s1))
# truncating strings to 3 letters and padding
print('{:>5.3}'.format(s1))
# truncating strings to 3 letters, central aligment and padding
print('{:^5.3}'.format(s1))
# output:
Tim
Tim
Tim
Output Formatting: format()
d: integer
f: float
s: string
:n---->n个宽度
:0n—>不足n个宽度用0补齐
.nf---->n位小数
# Number formating
a = 53
b = 4.897
print ("{:4d}".format(a))
print ("{:04d}".format(a))
print ("{:4.2f}".format(b))
print ("{:06.2f}".format(b))
# output:
53
0053
4.90
004.90
• <: left align
• >: right align
• =: padding between the sign and number 符号和数字之单填空格
• ^: centered
# Number formating
a = 53
b = 4.897
# Alignement
print ("{:^4d}".format(a))
print ("{:>5.2f}".format(b))
print ("{:<5.2f}".format(b))
print ("{:=7.2f}".format(-b))
# output:
53
4.90
4.90
- 4.90