Android中获取系统时间有多种方法,可分为Java中Calendar类获取,java.util.date类实现,还有android中Time实现。
现总结如下:
方法一:
1
2
3
4
5
6
7
|
void
getTime1(){
long
time=System.currentTimeMillis();
//long now = android.os.SystemClock.uptimeMillis();
SimpleDateFormat format=
new
SimpleDateFormat(
"yyyy-MM-dd HH:mm:ss"
);
Date d1=
new
Date(time);
String t1=format.format(d1);
Log.e(
"msg"
, t1);
}
|
方法二:
1
2
3
|
SimpleDateFormat format =
new
SimpleDateFormat(
"yyyy-MM-dd-HH:mm:ss"
);
String t=format.format(
new
Date());
Log.e(
"msg"
, t);
|
方法三:
1
2
3
4
5
6
7
8
9
|
void
getTime3(){
Calendar calendar = Calendar.getInstance();
String created = calendar.get(Calendar.YEAR) +
"年"
+ (calendar.get(Calendar.MONTH)+
1
) +
"月"
//从0计算
+ calendar.get(Calendar.DAY_OF_MONTH) +
"日"
+ calendar.get(Calendar.HOUR_OF_DAY) +
"时"
+ calendar.get(Calendar.MINUTE) +
"分"
+calendar.get(Calendar.SECOND)+
"s"
;
Log.e(
"msg"
, created);
}
|
方法四:
1
2
3
4
5
6
|
void
getTime4(){
Time t=
new
Time();
// or Time t=new Time("GMT+8"); 加上Time Zone资料。
t.setToNow();
// 取得系统时间。
String time=t.year+
"年 "
+(t.month+
1
)+
"月 "
+t.monthDay+
"日 "
+t.hour+
"h "
+t.minute+
"m "
+t.second;
Log.e(
"msg"
, time);
}
|
获取星期日期:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
Calendar calendar = Calendar.getInstance();
int
day = calendar.get(Calendar.DAY_OF_WEEK);
String today =
null
;
if
(day ==
2
) {
today =
"Monday"
;
}
else
if
(day ==
3
) {
today =
"Tuesday"
;
}
else
if
(day ==
4
) {
today =
"Wednesday"
;
}
else
if
(day ==
5
) {
today =
"Thursday"
;
}
else
if
(day ==
6
) {
today =
"Friday"
;
}
else
if
(day ==
7
) {
today =
"Saturday"
;
}
else
if
(day ==
1
) {
today =
"Sunday"
;
}
System.out.println(
"Today is:- "
+ today);
|
最后说一下日期格式化,日期格式化通常使用SimpleDateFormat类实现,其中的日期格式不能够自己随意定义,主要有以下几种形式:
1
2
|
SimpleDateFormat f1=
new
SimpleDateFormat();
//其中没有些格式化参数,我们使用默认的日期格式。
System.out.println(f.formate(
new
Date()));
|
代码输出的日期格式为:12-3-22 下午4:36
1
2
3
4
5
6
7
|
SimpleDateFormat f4=
new
SimpleDateFormat(
"今天是"
+
"yyyy年MM月dd日 E kk点mm分"
);
//可根据不同样式请求显示不同日期格式,要显示星期可以添加E参数
System.out.println(f4.format(
new
Date()));
//代码输出的日期格式为:今天是2012年03月22日 星期四 16点46分
SimpleDateFormat formater =
new
SimpleDateFormat(
"yyyyMMdd hh:mm:ss"
);
System.out.println(
"Date to String "
+formater.format(
new
Date()));
//相近的常用形式还有 yyMMdd hh:mm:ss yyyy-MM-dd hh:mm:ss dd-MM-yyyy hh:mm:ss
|
应有的时候通常还会需要把具体日期转换为毫秒或者Timestamp形式,如下:
文本 - > Timestamp,日期 -> Timestamp
扫描二维码关注公众号,回复:
1257782 查看本文章
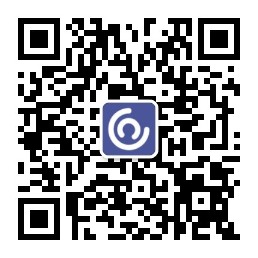
1
2
3
4
5
6
7
8
9
10
|
Timestamp t ;
SimpleDateFormat format =
new
SimpleDateFormat(
"yyyy-MM-dd hh:mm:ss"
);
try
...{
t =
new
Timestamp(format.parse(
"2007-07-19 00:00:00"
).getTime());
}
catch
(ParseException e) ...{
e.printStackTrace();
}
Timestamp t ;
SimpleDateFormat format =
new
SimpleDateFormat(
"yyyy-MM-dd hh:mm:ss"
);
t =
new
Timestamp(
new
Date().getTime());
|
以上就是本文的全部内容,希望对大家的学习有所帮助,也希望大家多多支持脚本之家。
+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
android获取时间差的方法
有些时候我们需要获取当前时间和某个时间之间的时间差,这时如何获取呢?
1. 引用如下命名空间:
1
2
|
import
java.util.Date;
import
android.text.format.DateFormat;
|
2. 设置时间格式:
1
|
SimpleDateFormat df =
new
SimpleDateFormat(
"yyyy-MM-dd HH:mm:ss"
);
|
3. 获取时间:
1
2
3
4
|
Date curDate =
new
Date(System.currentTimeMillis());
//PROCESSING
Date endDate =
new
Date(System.currentTimeMillis());
long
diff = endDate.getTime() - curDate.getTime();
|
这样获取的就是时间间隔了,并且是ms级别的。
希望本文所述对大家的Android程序设计有所帮助。
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
二、获取网络时间
很多时候,由于手机的不确定性,导致各个手机的时间都不尽相同,如果开发中需要获取统一的时间来匹配一些规则的时候,比如我之前公司就是通过或去当前时间并且转换之后来匹配网络连接的规则,防止大批量的抓包,那么这个时候就需要一个统一的时间和后台进行匹配,这是简单的使用获取系统时间就可能存在问题,用户手机如果调成自动获取网络时间的话没问题,但是如果不是呢?所以获取网络时间就派上了用处
1
2
3
4
5
6
7
8
9
10
|
URL url =
null
;
//取得资源对象
try
{
URLConnection uc = url.openConnection();
//生成连接对象
uc.connect();
//发出连接
ld = uc.getDate();
//取得网站日期时间
Logger.i(TAG,
"ld---->>>>"
+ld);
}
catch
(Exception e) {
e.printStackTrace();
}
|