state与this指向
有状态和无状态组件
- 函数组件叫做无状态组件;类组件叫做有状态组件
- 状态 (
state
),组件中使用的数据都保存在state
中,并且是组件的私有数据 - 函数组件只负责数据展示(静)
- 类组件有状态,负责动态展示 UI 界面 (动)
state的基本使用
state
用来为组件绑定私有数据,有两种绑定形式
第一种: 在构造函数中定义 state
第二种: 在类中直接定义state
(推荐)
在构造函数中定义 state
class Hero extends React.Component {
// 使用构造函数定义数据
constructor () {
super ()
this.state = {
name: '皮卡丘',
level: '1',
hp: 1680,
mp: 1320
}
}
}
在类中直接定义state
class Hero extends React.Component {
// 定义state (数据)
state = {
name: '安琪拉',
level: 1,
hp: 680,
mp: 320
}
}
案例:在类组件中定义私有数据,并在页面上渲染出来
import React from 'react'
import ReactDOM from 'react-dom'
// 目标: 使用 state 定义数据,并在页面上展示出来
class Hero extends React.Component {
// 定义state (数据)
state = {
name: '安琪拉',
level: 1,
hp: 680,
mp: 320
}
render () {
// 使用 {} 调用数据
return (
<div>
<ul>
<li>英雄: {
this.state.name}</li>
<li>等级: {
this.state.level}</li>
<li>血量: {
this.state.hp}</li>
<li>蓝量: {
this.state.mp}</li>
</ul>
<button>升1级</button>
</div>
)
}
}
ReactDOM.render(<Hero />, document.querySelector('#app'))
效果:
使用setState()方法修改数据
state = {
name: 'zs',
age: 20
}
this.setState({
name: 'ls',
age: 25
})
修改数据需要使用到 setState()
方法,方法内是一个对象参数,要修改哪个数据写哪个数据即可
案例:将上例button按钮上注册点击事件,点击按钮,页面上的数值发生变化
import React from 'react'
import ReactDOM from 'react-dom'
// 目标: button按钮上注册点击事件,每升一级,hp增加100,mp增加120
class Hero extends React.Component {
// 定义state (数据)
state = {
name: '安琪拉',
level: 1,
hp: 680,
mp: 320
}
upLevel = () => {
if (this.state.level === 18) {
alert('已经到达满级了')
return
}
this.setState({
level: this.state.level + 1,
hp: this.state.hp + 100,
mp: this.state.mp +120
})
}
render () {
return (
<div>
<ul>
<li>英雄: {
this.state.name}</li>
<li>等级: {
this.state.level}</li>
<li>血量: {
this.state.hp}</li>
<li>蓝量: {
this.state.mp}</li>
</ul>
<button onClick = {
this.upLevel}>升1级</button>
</div>
)
}
}
ReactDOM.render(<Hero />, document.querySelector('#app'))
效果:
this的指向
在上例中如果将 onClick 指定的事件函数独立出来定义成一个函数则会报错,此时函数内部的this是undefined
扫描二维码关注公众号,回复:
12482119 查看本文章
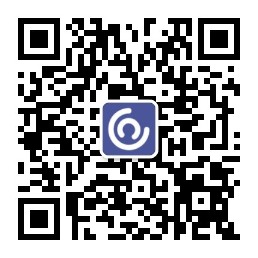
upLevel () {
this.setState({
level: this.state.level + 1,
hp: this.state.hp + 100,
mp: this.state.mp + 120
})
}
原因是:this 的指向不同
- 事件函数直接定义在类当中,this指向 undefined
- 事件函数写在 onClick 中,this指向 Hero 组件
解决办法:
- 使用箭头函数代替传统函数
- 使用
bind()
方法修改this指向this.upLevel = this.upLevel.bind(this)
- call、apply、bind 等方法也可以
利用箭头函数解决this指向问题
使用箭头函数代替传统函数方式,调整this的指向
upLevel = () => {
this.setState({
level: this.state.level + 1,
hp: this.state.hp + 100,
mp: this.state.mp + 120
})
}
使用{}
调用数据
render () {
return (
<div>
<ul>
<li>英雄: {
this.state.name}</li>
<li>等级: {
this.state.level}</li>
<li>血量: {
this.state.hp}</li>
<li>蓝量: {
this.state.mp}</li>
</ul>
<button onClick={
this.upLevel}>升1级</button>
</div>
)
}
}
利用bind()方法解决this指向问题
如果想要使用传统的函数定义方式,则需要使用==bind()==方法来修改this指向
this.upLevel = this.upLevel.bind(this)
}