最近用java写一些算法时,经常遇到要使用栈和队列结构(比如树的各种遍历等等),使用栈的话,Stack已经不被推荐使用了,所以栈和队列我们通常都是用LinkedList这种双链表结构实现,用的多了自然就开始好奇它的各种操作具体是怎么实现的?
先下面分别从用法和源码角度简单记录下如何用LinkedList实现栈和队列
作为栈使用时,push是入栈,pop / poll是出栈,peek是获取栈顶元素
具体使用如下示例:
LinkedList<String> stack = new LinkedList<>();
stack.push("a");
stack.push("b");
stack.push("c");
stack.push("d");
System.out.println(stack.peek());//栈为d c b a,输出:d
System.out.println(stack.poll());//栈为d c b a,输出:
System.out.println(stack.pop());//栈为c b a,输出c
push源码分析:
在使用LinkedList作为栈时,我们可以去看一下源码中push方法的实现,可以看到,push使用的时双链表的头插法,也就是在链表头部插入元素
public void push(E e) {
addFirst(e);
}
public void addFirst(E e) {
linkFirst(e);
}
private void linkFirst(E e) {
final Node<E> f = first;
final Node<E> newNode = new Node<>(null, e, f);
first = newNode;
if (f == null)
last = newNode;
else
f.prev = newNode;
size++;
modCount++;
}
作为队列使用时,offer是入队列,poll是出队列,peek是获取队头元素
但LinkedList实现队列有两种用法
①直接使用LinkedList提供的offer和poll来实现出队、入队、取队头
LinkedList<String> queue = new LinkedList<>();
queue.offer("a");
queue.offer("b");
queue.offer("c");
queue.offer("d");
System.out.println(queue.peek());//队列为:a b c d,输出:a
System.out.println(queue.poll());//队列为:a b c d,输出:a
System.out.println(queue.pop());//队列为:b c d,输出:
②LinkedList实现了Deque接口,Deque继承自Queue,所以可以使用父类指向子类的方式来访问子类方法从而实现队列。
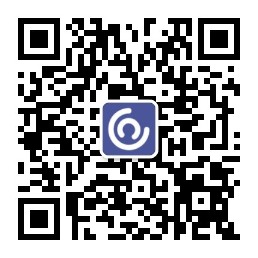
注意:Queue接口中出队列只提供了poll方法,所以LinkedList的pop方法就不能使用了。
LinkedList<String> queue = new LinkedList<>();
queue.offer("a");
queue.offer("b");
queue.offer("c");
queue.offer("d");
System.out.println(queue.peek());//队列为:a b c d,输出:a
System.out.println(queue.poll());//队列为:a b c d,输出:a
offer源码分析:
使用LinkedList作为队列时,offer方法就是从双向链表尾部添加元素,然后取出时调用pop/poll/peek从链表头部取出,从而实现FIFO。
public boolean offer(E e) {
return add(e);
}
public boolean add(E e) {
linkLast(e);
return true;
}
void linkLast(E e) {
final Node<E> l = last;
final Node<E> newNode = new Node<>(l, e, null);
last = newNode;
if (l == null)
first = newNode;
else
l.next = newNode;
size++;
modCount++;
}
综合上面的再补充一些总结:
push:头插,在双链表头部插入元素。
offer / add:尾插,在双链表尾部插入元素。
pop / poll:在双链表头部取出元素(取出时会删除该元素)。
pop和poll的区别是,如果获取元素为空时pop会抛出异常,poll会返回null。限于篇幅这里就不粘贴源码了,有兴趣的可以自己去翻一翻。
peek:在双链表头部读取元素(不会删除元素)。
水平有限,如有错误,麻烦评论或私信修改,谢谢了