1.按照时间生成文件,将每天的磁盘使用状态写入到对应的文件中(2020-06-02.log)
分析:
1.时间打印:date +%F
2.磁盘状态:df -h
#!/bin/bash
#每日磁盘使用状态
#v1.0 by zjz
df -h > /tmp/`date +%F`-disk.log
[root@localhost ]#crontab
30 23 * * * /shell/1day-disk-status.sh
2. 统计nginx每个IP的访问量?
分析:
1.筛选出所有IP地址
2.排序、去重、统计
wc
uniq
写一个脚本,计算100以内能被3整除的正整数之和
分析:
1.找出1-100内能除于3等于0的数
2.让数值相加
[root@localhost shell]# cat 3%.sh
#!/bin/bash
#计算100以内能被3整除的正整数之和
sum=0 #赋值为0
for i in {
1..100} #循环1到100的数
do
xc=$[$i%3] #将i除于3
if [ $xc -eq 0 ];then #判断是否被整除,整除=0,将i(可整除的数累加到sum)
sum=$[$i+$sum]
fi
done
echo $sum
执行结果:
[root@localhost shell]# bash 3%.sh
1683
用shell打印下面这句话中字母数小于6个的单词
Bash also interprets a number of multi-user options
分析:
1.使用循环遍历
2.统计一个单词的字母数 wc -c
#!/bin/bash
#统计一个单词的字母数
#v1.0 by zjz 20200618
for i in Bash also interprets a number of multi-user options
do
number=$(echo $i | wc -c )
if [ $number -lt 7 ];then
echo $i
fi
done
执行结果:
[root@localhost ~]# bash shell/tongji.sh
Bash
also
a
of
添加user_00->user_09 10个用户,并设置一个随机密码(10位大小写字母加数字),注意需要把每个用户的密码记录到一个日志文件中。
分析:
1.10个用户 echo user_{00..09}
或seq -w 0 10
2.随机密码 mkpasswd (expect)
mkpasswd命令
需安装# yum install -y expect-5.45-14.el7_1.x86_64`
-l 定义密码长度,默认为0
-d 数字个数,默认为2
-c 小写字符,默认为3
-C 大写字符,默认位2
-s 特殊字符,默认位1
-v version
方式一:
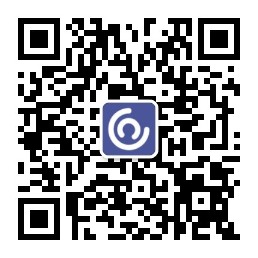
[root@localhost shell]# vim add-user09.sh
#!/bin/bash
# 生成10个用户,并设置密码
# v1.0 by zjz 20200618
if [ $UID -ne 0 ];then
echo "please use root account"
exit 1
fi
for i in $(seq -w 50 59)
do
username=user_$i
pass=`mkpasswd`
id $username &>/dev/null
if [ $? -eq 1 ];then
useradd $username && \
echo "$pass" | passwd --stdin $username &>/dev/null
if [ $? -eq 0 ];then
echo "user $i create ok"
else
echo "user $i create error"
fi
else
echo "user $username is alreay exists"
fi
echo -e "$username \t $pass" >> user_pass.txt
done
方式二:
[root@localhost shell]# cat add-user09.sh
#!/bin/bash
# 生成10个用户,并设置密码
# v1.0 by zjz 20200618
if [ $UID -ne 0 ];then
echo "please use root account"
exit 1
fi
for i in $(echo user{
70..79})
do
#username=user_$i
pass=`mkpasswd`
id $i &>/dev/null
if [ $? -eq 1 ];then
useradd $i && \
echo "$pass" | passwd --stdin $i &>/dev/null
if [ $? -eq 0 ];then
echo "user $i create ok"
else
echo "user $i create error"
fi
else
echo "user $i is alreay exists"
fi
echo -e "$i \t $pass" >> user_pass_echo.txt
done
执行结果:
[root@localhost shell]# bash add-user09.sh
user 50 create ok
user 51 create ok
user 52 create ok
user 53 create ok
user 54 create ok
user 55 create ok
查看账号密码表:
[root@localhost shell]# ll
total 16
-rw-r--r-- 1 root root 113 Jun 19 03:09 3%.sh
-rw-r--r-- 1 root root 533 Jun 19 15:25 add-user09.sh
-rw-r--r-- 1 root root 206 Jun 19 04:12 tongji.sh
-rw-r--r-- 1 root root 400 Jun 19 15:25 user_pass.txt
[root@localhost shell]# cat user_pass.txt
user_40 e9kz3lBX=
user_41 oHej"x10A
user_42 ytdo5C{8M
user_43 9vcLJ6?rl
user_44 Wku3oW+k0
user_45 (4tDXpea7
user_46 ygQx*Od43
user_47 d1nG0aVf@
user_48 k8Dz4E?lm
user_49 =k57gNohI
统计linux中普通用户有多少个
分析:
1.查看/etc/passwd文件
2.普通用户UID大于等于1000的
半成品:
#!/bin/bash
declare -A array_uid
for i in `cat /etc/passwd | awk -F ":" '{print $3}'`
do
if [ $i -gt 999 ];then
let array_uid[$i]++
else
continue
fi
done
for j in ${!array_uid[*]}
do
echo "uid: $j, uid_count:${array_uid[$j]}"
done
方式一:
[root@localhost shell]# cat tongji-1000.sh
#!/bin/bash
username=` cat /etc/passwd | awk -F ":" '{print $1}'`
username_uid=`cat /etc/passwd | awk -F ":" '{print $3}'`
totle_uid=`cat /etc/passwd | awk -F ":" '$3>1000' | wc -l`
for i in $username_uid
do
if [ $i -ge 1000 ];then
#这一句有点东西
username_and_uid=`cat /etc/passwd | awk -F ":" '{print $1","$3}' | grep $i`
echo $username_and_uid
fi
done
echo "TOTAL USER COUNT:$totle_uid"
执行结果:
[root@localhost shell]# bash tongji-1000.sh
user70,1000
user71,1001
user72,1002
user73,1003
user74,1004
user75,1005
user76,1006
user77,1007
user78,1008
user79,1009
TOTAL USER COUNT:9
写一个shell脚本查看使用最多的命令是哪些,列出常用的命令top10
方式一:
[root@localhost ~]# history | awk '{print $2}' | sort | uniq -c | sort -rn | head
80 vim
74 ll
46 cd
39 bash
31 systemctl
31 cat
27 yum
26 /usr/local/python3/bin/python3
13 ping
13 history
方式二:
[root@localhost ~]# hash | sort -rn | head
1 /usr/bin/man
hits command
方式三:
[root@localhost ~]# cat ~/.bash_history | sort | uniq -c | sort -rn | head
72 ll
11 systemctl restart network
10 ip add
9 vim shell/tongji.sh
9 vim shell/add-user09.sh
9 ping www.baidu.com
9 bash shell/tongji.sh
8 bash shell/add-user09.sh
7 vim add-user09.sh
7 cat /etc/passwd
写一个脚本判断Linux服务器是否开启web-80服务,并判断是nginx还是httpd
分析:
1.判断80端口是否开放
2.grep nginx 或grep httpd 查看开启的服务
[root@localhost ~]# cat shell/web.sh
#!/bin/bash
nginx_status=`ss -lntup |grep "*:80" | grep nginx | wc -l`
httpd_status=`ss -lntup |grep "*:80" | grep httpd | wc -l`
if [[ $nginx_status -eq 0 && $httpd_status -eq 0 ]];then
echo "80port not start"
exit 1
fi
if [ $nginx_status -eq 1 ];then
echo "U satrt nginx"
fi
if [ $httpd_status -eq 1 ];then
echo "U satrt httpd"
fi
执行结果:
[root@localhost ~]# bash shell/web.sh
U satrt nginx
判断mysql服务是否正常,检查当前的mysql服务是主或从。
已知:
1.当前mysql服务root密码为Aa123456
分析:
1.使用非交互式的方式登录mysql进行取值 mysql -uroot -pAa123456 -e “show databases;”
2.检查mysql服务的主从。如果是从,判断主从服务是否正常,如果是主,啥事没有
3.检查从的IO线程和SQL线程是否正常
systemctl status mysqld | grep running
ps -aux | grep mysql.sock | grep -v color
输入网卡的名称,用脚本输出网卡的IP
分析:
1、取得设备中所有的网卡的名称
2、选取相应的网卡名称输出对应IP
#!/bin/bash
#输入网卡的名称,用脚本输出网卡的IP
#v1.0 by zjz 2020-0622
netname=`ifconfig -s | awk '{print $1}' | grep -v 'Iface'`
#netcount=`ifconfig -s | awk '{print $1}' | grep -v 'Iface' |wc -l`
echo "HOSTNAME NETWORK-DEVICE:" $netname
read -p "Please search NETWORK NAME:" netnumber
netip=`ifconfig $netnumber | grep -w "inet" |awk '{print $2}'`
echo "NETWORK-DEVICE IP:" $netip
写一个脚本判断输入的用户是否登入,如果未登陆提示没有登陆。如果登陆,显示登陆的终端以及通过那个IP登陆
#!/bin/bash
#判断输入的用户是否登入,如果未登陆提示没有登陆。如果登陆,显示登陆的终端以及通过那个IP登陆
#v1.0 by zjz 2020-0623
read -p "请输入你想要查看的用户:" user
login_user=`who|grep "$user" &>/dev/null;echo $?` #判断用户是否登录
login_total=`w | grep "$user" |awk '{print $1,$2,$3}'` #输出登录用户的IP
if [ $login_user -eq 0 ];then
echo $login_total|xargs -n3
else
echo "no login user"
fi
写一个shell脚本,通过curl -l返回的状态码来判断所访问的网站是否正常,比如:当状态码为200时,才算正常
[root@bogon ~]# cat shell/web-status.sh
#!/bin/bash
#通过返回状态码,判断访问的网页是否正常
#v1.0 by zjz 2020-0624
read -p "请输入要查看网站地址:" web
status=`curl -sI $web | grep HTTP | awk '{print $2}'`
if [ $status -eq 200 -o $status -eq 301 -o $status -eq 302 ];then
echo "你的网站是正常的,返回值" $status
else
echo "你的网站是不正常的,返回值" $status
fi
执行结果:
[root@bogon ~]# bash shell/web-status.sh
请输入要查看网站地址:www.baidu.com
你的网站是正常的,返回值 200
[root@bogon ~]# bash shell/web-status.sh
请输入要查看网站地址:192.168.31.251
你的网站是正常的,返回值 302
已知Nginx访问的日志文件在/var/log/nginx/access.log内,请统计下早上10点到12点来访IP最多的是哪个?
grep "27/May/2020:1[0-2]:[0-5]:[0-9]" access.log | awk '{print $1}' |sort |uniq -c | sort -rn | head
更多更好的原创文章,请访问官方网站: 点我就能跳转咯-》https://nothingzh.gitee.io
也可关注“哎呦运维”微信订阅号,随时接受文章推送。